本篇是创建游戏内核(7)【接口与实现分离版】的续篇,关于该内核的细节说明请参考创建游戏内核(8),这个版本主要是按照功能划分模块的思想,并严格按照接口与实现相分离的原则来写的,没有用面向对象的思想来写,没有继承没有多态。大家可以对比两个版本,比较优劣。
接口:
void set_light_type(D3DLIGHT9* light, D3DLIGHTTYPE type);
void move_light(D3DLIGHT9* light,
float x_pos, float y_pos, float z_pos);
void move_light_rel(D3DLIGHT9* light,
float x_pos, float y_pos, float z_pos);
void get_light_pos(const D3DLIGHT9* light,
float* x_pos, float* y_pos, float* z_pos);
void point_light(D3DLIGHT9* light,
float x_from, float y_from, float z_from,
float x_at, float y_at, float z_at);
void get_light_direction(const D3DLIGHT9* light,
float* x_dir, float* y_dir, float* z_dir);
void set_light_diffuse(D3DLIGHT9* light,
unsigned char red, unsigned char green, unsigned char blue);
void get_light_diffuse(const D3DLIGHT9* light,
unsigned char* red, unsigned char* green, unsigned char* blue);
void set_light_specular(D3DLIGHT9* light,
unsigned char red, unsigned char green, unsigned char blue);
void get_light_specular(const D3DLIGHT9* light,
unsigned char* red, unsigned char* green, unsigned char* blue);
void set_light_ambient(D3DLIGHT9* light,
unsigned char red, unsigned char green, unsigned char blue);
void get_light_ambient(const D3DLIGHT9* light,
unsigned char* red, unsigned char* green, unsigned char* blue);
void set_light_range(D3DLIGHT9* light, float range);
float get_light_range(const D3DLIGHT9* light);
void set_light_falloff(D3DLIGHT9* light, float falloff);
float get_light_falloff(const D3DLIGHT9* light);
void set_light_attenuation_0(D3DLIGHT9* light, float attenuation);
float get_light_attenuation_0(const D3DLIGHT9* light);
void set_light_attenuation_1(D3DLIGHT9* light, float attenuation);
float get_light_attenuation_1(const D3DLIGHT9* light);
void set_light_attenuation_2(D3DLIGHT9* light, float attenuation);
float get_light_attenuation_2(const D3DLIGHT9* light);
void set_light_theta(D3DLIGHT9* light, float theta);
float get_light_theta(const D3DLIGHT9* light);
void set_light_phi(D3DLIGHT9* light, float phi);
float get_light_phi(const D3DLIGHT9* light);
实现:
//---------------------------------------------------------------------------
// set light type (D3DLIGHT_POINT, D3DLIGHT_SPOT, D3DLIGHT_DIRECTIONAL).
//---------------------------------------------------------------------------
void set_light_type(D3DLIGHT9* light, D3DLIGHTTYPE type)
{
light->Type = type;
}
//---------------------------------------------------------------------------
// move light source to specified position.
//---------------------------------------------------------------------------
void move_light(D3DLIGHT9* light,
float x_pos, float y_pos, float z_pos)
{
light->Position.x = x_pos;
light->Position.y = y_pos;
light->Position.z = z_pos;
}
//---------------------------------------------------------------------------
// move light source to specified position which is relative to current position.
//---------------------------------------------------------------------------
void move_light_rel(D3DLIGHT9* light,
float x_pos, float y_pos, float z_pos)
{
light->Position.x += x_pos;
light->Position.y += y_pos;
light->Position.z += z_pos;
}
//---------------------------------------------------------------------------
// Get current position.
//---------------------------------------------------------------------------
void get_light_pos(const D3DLIGHT9* light,
float* x_pos, float* y_pos, float* z_pos)
{
if(x_pos != NULL)
*x_pos = light->Position.x;
if(y_pos != NULL)
*y_pos = light->Position.y;
if(z_pos != NULL)
*z_pos = light->Position.z;
}
//---------------------------------------------------------------------------
// move light source to specified position and pointer it to specified direction.
//---------------------------------------------------------------------------
void point_light(D3DLIGHT9* light,
float x_from, float y_from, float z_from,
float x_at, float y_at, float z_at)
{
// move the light
move_light(light, x_from, y_from, z_from);
// calculate vector between angles
light->Direction.x = x_at - x_from;
light->Direction.y = y_at - y_from;
light->Direction.z = z_at - z_from;
}
//---------------------------------------------------------------------------
// Get the direction of current light source.
//---------------------------------------------------------------------------
void get_light_direction(const D3DLIGHT9* light,
float* x_dir, float* y_dir, float* z_dir)
{
if(x_dir != NULL)
*x_dir = light->Direction.x;
if(y_dir != NULL)
*y_dir = light->Direction.y;
if(z_dir != NULL)
*z_dir = light->Direction.z;
}
//---------------------------------------------------------------------------
// set diffuse color of light source.
//---------------------------------------------------------------------------
void set_light_diffuse(D3DLIGHT9* light,
unsigned char red, unsigned char green, unsigned char blue)
{
light->Diffuse.r = red / 255.0f;
light->Diffuse.g = green / 255.0f;
light->Diffuse.b = blue / 255.0f;
}
//---------------------------------------------------------------------------
// Get diffuse color of light source.
//---------------------------------------------------------------------------
void get_light_diffuse(const D3DLIGHT9* light,
unsigned char* red, unsigned char* green, unsigned char* blue)
{
if(red != NULL)
*red = (unsigned char)(255.0f * light->Diffuse.r);
if(green != NULL)
*green = (unsigned char)(255.0f * light->Diffuse.g);
if(blue != NULL)
*blue = (unsigned char)(255.0f * light->Diffuse.b);
}
//---------------------------------------------------------------------------
// set specular color of light source.
//---------------------------------------------------------------------------
void set_light_specular(D3DLIGHT9* light,
unsigned char red, unsigned char green, unsigned char blue)
{
light->Specular.r = red / 255.0f;
light->Specular.g = green / 255.0f;
light->Specular.b = blue / 255.0f;
}
//---------------------------------------------------------------------------
// Get specular color of light source.
//---------------------------------------------------------------------------
void get_light_specular(const D3DLIGHT9* light,
unsigned char* red, unsigned char* green, unsigned char* blue)
{
if(red != NULL)
*red = (unsigned char)(255.0f * light->Specular.r);
if(green != NULL)
*green = (unsigned char)(255.0f * light->Specular.g);
if(blue != NULL)
*blue = (unsigned char)(255.0f * light->Specular.b);
}
//---------------------------------------------------------------------------
// set ambient color of light source.
//---------------------------------------------------------------------------
void set_light_ambient(D3DLIGHT9* light,
unsigned char red, unsigned char green, unsigned char blue)
{
light->Ambient.r = red / 255.0f;
light->Ambient.g = green / 255.0f;
light->Ambient.b = blue / 255.0f;
}
//---------------------------------------------------------------------------
// Get ambient color of light source.
//---------------------------------------------------------------------------
void get_light_ambient(const D3DLIGHT9* light,
unsigned char* red, unsigned char* green, unsigned char* blue)
{
if(red != NULL)
*red = (unsigned char)(255.0f * light->Ambient.r);
if(green != NULL)
*green = (unsigned char)(255.0f * light->Ambient.g);
if(blue != NULL)
*blue = (unsigned char)(255.0f * light->Ambient.b);
}
//---------------------------------------------------------------------------
// set the range of light source.
//---------------------------------------------------------------------------
void set_light_range(D3DLIGHT9* light, float range)
{
light->Range = range;
}
//---------------------------------------------------------------------------
// Get the range of light source.
//---------------------------------------------------------------------------
float get_light_range(const D3DLIGHT9* light)
{
return light->Range;
}
//---------------------------------------------------------------------------
// set the fallof of light source.
//---------------------------------------------------------------------------
void set_light_falloff(D3DLIGHT9* light, float falloff)
{
light->Falloff = falloff;
}
//---------------------------------------------------------------------------
// Get the fallof of light source.
//---------------------------------------------------------------------------
float get_light_falloff(const D3DLIGHT9* light)
{
return light->Falloff;
}
//---------------------------------------------------------------------------
// set attenuation 0 of light source.
//---------------------------------------------------------------------------
void set_light_attenuation_0(D3DLIGHT9* light, float attenuation)
{
light->Attenuation0 = attenuation;
}
//---------------------------------------------------------------------------
// Get attenuation 0 of light source.
//---------------------------------------------------------------------------
float get_light_attenuation_0(const D3DLIGHT9* light)
{
return light->Attenuation0;
}
//---------------------------------------------------------------------------
// set attenuation 1 of light source.
//---------------------------------------------------------------------------
void set_light_attenuation_1(D3DLIGHT9* light, float attenuation)
{
light->Attenuation1 = attenuation;
}
//---------------------------------------------------------------------------
// Get attenuation 1 of light source.
//---------------------------------------------------------------------------
float get_light_attenuation_1(const D3DLIGHT9* light)
{
return light->Attenuation1;
}
//---------------------------------------------------------------------------
// set attenuation 2 of light source.
//---------------------------------------------------------------------------
void set_light_attenuation_2(D3DLIGHT9* light, float attenuation)
{
light->Attenuation2 = attenuation;
}
//---------------------------------------------------------------------------
// Get attenuation 2 of light source.
//---------------------------------------------------------------------------
float get_light_attenuation_2(const D3DLIGHT9* light)
{
return light->Attenuation2;
}
//---------------------------------------------------------------------------
// set angle thera of light source.
//---------------------------------------------------------------------------
void set_light_theta(D3DLIGHT9* light, float theta)
{
light->Theta = theta;
}
//---------------------------------------------------------------------------
// Get angle thera of light source.
//---------------------------------------------------------------------------
float get_light_theta(const D3DLIGHT9* light)
{
return light->Theta;
}
//---------------------------------------------------------------------------
// set angle phi of light source.
//---------------------------------------------------------------------------
void set_light_phi(D3DLIGHT9* light, float phi)
{
light->Phi = phi;
}
//---------------------------------------------------------------------------
// Get angle phi of light source.
//---------------------------------------------------------------------------
float get_light_phi(const D3DLIGHT9* light)
{
return light->Phi;
}
测试代码:
/***********************************************************************************
PURPOSE:
Test D3D light function.
***********************************************************************************/
#include <windows.h>
#include "core_framework.h"
#include "core_graphics.h"
#include "core_tool.h"
// The 3D vertex format and descriptor
typedef struct
{
float x, y, z; // 3D coordinates
float nx, ny, nz; // normals
D3DCOLOR diffuse; // color
} VERTEX;
#define VERTEX_FVF (D3DFVF_XYZ | D3DFVF_NORMAL | D3DFVF_DIFFUSE)
IDirect3DVertexBuffer9* g_vertex_buffer;
//--------------------------------------------------------------------------------
// Initialize data for game.
//--------------------------------------------------------------------------------
BOOL game_init()
{
// initialize vertex data
VERTEX _verts[] = {
{ -100.0f, 100.0f, -100.0f, 0.0f,0.0f,-1.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ 100.0f, 100.0f, -100.0f, 0.0f,0.0f,-1.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ -100.0f, -100.0f, -100.0f, 0.0f,0.0f,-1.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ 100.0f, -100.0f, -100.0f, 0.0f,0.0f,-1.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ 100.0f, 100.0f, -100.0f, 1.0f,0.0f,0.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ 100.0f, 100.0f, 100.0f, 1.0f,0.0f,0.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ 100.0f, -100.0f, -100.0f, 1.0f,0.0f,0.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ 100.0f, -100.0f, 100.0f, 1.0f,0.0f,0.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ 100.0f, 100.0f, 100.0f, 0.0f,0.0f,1.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ -100.0f, 100.0f, 100.0f, 0.0f,0.0f,1.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ 100.0f, -100.0f, 100.0f, 0.0f,0.0f,1.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ -100.0f, -100.0f, 100.0f, 0.0f,0.0f,1.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ -100.0f, 100.0f, 100.0f, -1.0f,0.0f,0.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ -100.0f, 100.0f, -100.0f, -1.0f,0.0f,0.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ -100.0f, -100.0f, 100.0f, -1.0f,0.0f,0.0f, D3DCOLOR_RGBA(255,255,255,255) },
{ -100.0f, -100.0f, -100.0f, -1.0f,0.0f,0.0f, D3DCOLOR_RGBA(255,255,255,255) }
};
// Create Direct3D and Direct3DDevice object
if(! create_display(g_hwnd, get_window_width(g_hwnd), get_window_height(g_hwnd), 16, TRUE, FALSE))
return FALSE;
// builds a left-handed perspective projection matrix based on a field of view
set_perspective(D3DX_PI/4.0, 1.33333f, 1.0f, 1000.0f);
D3DXMATRIX _mat_view;
// create and set the view matrix
D3DXMatrixLookAtLH(&_mat_view,
&D3DXVECTOR3(0.0f, 0.0f, -500.0f),
&D3DXVECTOR3(0.0f, 0.0f, 0.0f),
&D3DXVECTOR3(0.0f, 1.0f, 0.0f));
g_d3d_device->SetTransform(D3DTS_VIEW, &_mat_view);
BYTE* _vertex_ptr;
// create the vertex buffer and set data
g_d3d_device->CreateVertexBuffer(sizeof(_verts), 0, VERTEX_FVF, D3DPOOL_DEFAULT, &g_vertex_buffer, NULL);
// locks a range of vertex data and obtains a pointer to the vertex buffer memory
g_vertex_buffer->Lock(0, 0, (void**)&_vertex_ptr, 0);
memcpy(_vertex_ptr, _verts, sizeof(_verts));
// unlocks vertex data
g_vertex_buffer->Unlock();
// enable d3d lighting
g_d3d_device->SetRenderState(D3DRS_LIGHTING, TRUE);
D3DLIGHT9 _light;
memset(&_light, 0, sizeof(_light));
// set light
set_light_type(&_light, D3DLIGHT_POINT);
set_light_diffuse(&_light, 128, 128, 0);
set_light_range(&_light, 1000.0);
set_light_attenuation_0(&_light, 0.5);
move_light(&_light, 300.0, 0.0, -600.0);
// attach light to d3d device and enable _light
g_d3d_device->SetLight(0, &_light);
// enale this light
g_d3d_device->LightEnable(0, TRUE);
return TRUE;
}
//--------------------------------------------------------------------------------
// Render every game frame.
//--------------------------------------------------------------------------------
BOOL game_frame()
{
clear_display_buffer(D3DCOLOR_RGBA(0, 0, 0, 0));
if(SUCCEEDED(g_d3d_device->BeginScene()))
{
D3DXMATRIX _mat_world;
// create and set the world transformation matrix
// rotate object along y-axis
D3DXMatrixRotationY(&_mat_world, (float) (timeGetTime() / 1000.0));
g_d3d_device->SetTransform(D3DTS_WORLD, &_mat_world);
// set the vertex stream, shader, and texture.
// binds a vertex buffer to a device data stream
g_d3d_device->SetStreamSource(0, g_vertex_buffer, 0, sizeof(VERTEX));
// set the current vertex stream declation
g_d3d_device->SetFVF(VERTEX_FVF);
// renders a sequence of noindexed, geometric primitives of the specified type from the current set
// of data input stream.
for(short i = 0; i < 4; i++)
g_d3d_device->DrawPrimitive(D3DPT_TRIANGLESTRIP, i * 4, 2);
g_d3d_device->EndScene();
}
present_display();
return TRUE;
}
//--------------------------------------------------------------------------------
// Release all game resources.
//--------------------------------------------------------------------------------
BOOL game_shutdown()
{
release_com(g_vertex_buffer);
release_com(g_d3d_device);
release_com(g_d3d);
return TRUE;
}
//--------------------------------------------------------------------------------
// Main function, routine entry.
//--------------------------------------------------------------------------------
int WINAPI WinMain(HINSTANCE inst, HINSTANCE pre_inst, LPSTR cmd_line, int cmd_show)
{
if(! build_window(inst, "MainClass", "MainWindow", WS_OVERLAPPEDWINDOW, 0, 0, 800, 600))
return FALSE;
run_game(game_init, game_frame, game_shutdown);
return 0;
}
点击下载源码和工程
程序截图:
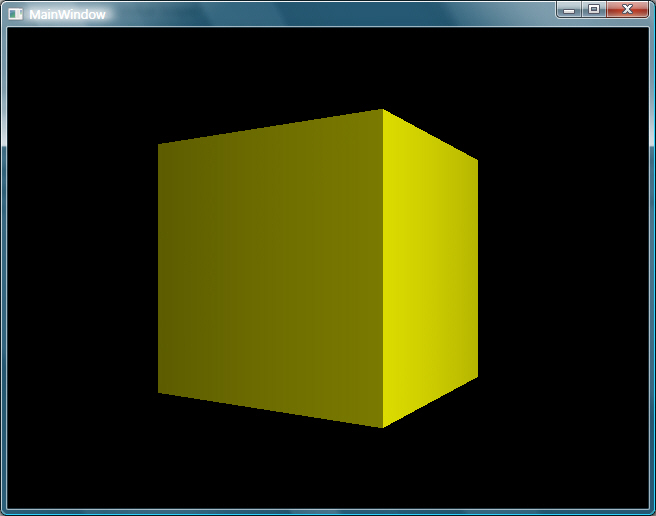