视区变换
视区(视口)变换是Direct3D顶点变换流水线的最后一步,它通过定义视区信息(屏幕显示区域的实际宽和高等参数),完成顶点裁剪以及将顶点坐标从投影坐标变换为最终显示的以像素为单位的屏幕坐标等操作。裁剪过程保证不渲染完全在观察平截面以外的对象,还确保对于与观察平截面相交的对象,可以如下方式进行渲染:即在视口指定范围以外的部分不绘制像素。
1、定义视区
视区结构D3DVIEWPORT9定义了Direct3D用以进行视区变换的各项参数:
Defines the window dimensions of a render-target
surface onto which a 3D volume projects.
typedef struct D3DVIEWPORT9 {
DWORD X;
DWORD Y;
DWORD Width;
DWORD Height;
float MinZ;
float MaxZ;
} D3DVIEWPORT9, *LPD3DVIEWPORT9;
Members
- X
- Pixel coordinate of the upper-left corner of the
viewport on the render-target surface. Unless you want to render to a subset
of the surface, this member can be set to 0.
- Y
- Pixel coordinate of the upper-left corner of the
viewport on the render-target surface. Unless you want to render to a subset
of the surface, this member can be set to 0.
- Width
- Width dimension of the clip volume, in pixels.
Unless you are rendering only to a subset of the surface, this member should
be set to the width dimension of the render-target surface.
- Height
- Height dimension of the clip volume, in pixels.
Unless you are rendering only to a subset of the surface, this member should
be set to the height dimension of the render-target surface.
- MinZ
- Together with MaxZ, value describing the range of
depth values into which a scene is to be rendered, the minimum and maximum
values of the clip volume. Most applications set this value to 0.0. Clipping
is performed after applying the projection matrix.
- MaxZ
- Together with MinZ, value describing the range of
depth values into which a scene is to be rendered, the minimum and maximum
values of the clip volume. Most applications set this value to 1.0. Clipping
is performed after applying the projection matrix.
Remarks
The X, Y, Width, and Height members describe the
position and dimensions of the viewport on the render-target surface. Usually,
applications render to the entire target surface; when rendering on a 640 x 480
surface, these members should be 0, 0, 640, and 480, respectively. The MinZ and
MaxZ are typically set to 0.0 and 1.0 but can be set to other values to achieve
specific effects. For example, you might set them both to 0.0 to force the
system to render objects to the foreground of a scene, or both to 1.0 to force
the objects into the background.
When the viewport parameters for a device change
(because of a call to the IDirect3DDevice9::SetViewport method), the driver
builds a new transformation matrix.
2、视区设置
使用函数IDirect3DDevice9::SetViewport()设置Direct3D的视区,其声明如下:
HRESULT SetViewport(
CONST D3DVIEWPORT9 * pViewport
);
SetViewport()的作用相当于把投影空间的顶点P(x, y, z, 1)乘以下面的矩阵:
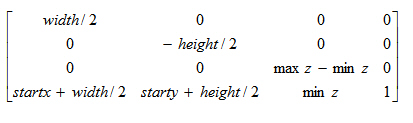
因此,屏幕上的二维坐标P'(x', y')的坐标等于:
x' = x * width/2 + startx + width/2
y' = y * (-height/2) + starty + height/2
z' = z * (maxz - minz) + minz
这些坐标被Direct3D用来进行裁剪。
与SetViewport()相对应,可以通过函数IDirect3DDevice9::GetViewport()获得当前视区的相关信息,该函数声明如下:
Retrieves the viewport parameters currently set for the
device.
HRESULT GetViewport(
D3DVIEWPORT9 * pViewport
);
Parameters
- pViewport
- [out] Pointer to a D3DVIEWPORT9 structure,
representing the returned viewport parameters.
Return Values
If the method succeeds, the return value is D3D_OK.
D3DERR_INVALIDCALL is returned if the pViewport parameter is invalid.
Remarks
Typically, methods that return state will not work on a
device that is created using D3DCREATE_PUREDEVICE. This method however, will
work even on a pure device.
3、清空视区
一般情况下,在绘制每一帧图形前都要先清空视区,即清空渲染目标表面上的视区矩形的内容:颜色缓冲区、深度缓冲区或者模板缓冲区。使用函数IDirect3DDevice9::Clear()来清空视区,该函数声明如下:
Clears one or more surfaces such as a render target,
multiple render targets, a stencil buffer, and a depth buffer.
HRESULT Clear(
DWORD Count,
CONST D3DRECT * pRects,
DWORD Flags,
D3DCOLOR Color,
float Z,
DWORD Stencil
);
Parameters
- Count
- [in] Number of rectangles in the array at pRects.
Must be set to 0 if pRects is NULL. May not be 0 if pRects is a valid
pointer.
- pRects
- [in] Pointer to an array of D3DRECT structures
that describe the rectangles to clear. Set a rectangle to the dimensions of
the rendering target to clear the entire surface. Each rectangle uses screen
coordinates that correspond to points on the render target. Coordinates are
clipped to the bounds of the viewport rectangle. To indicate that the entire
viewport rectangle is to be cleared, set this parameter to NULL and Count to
0.
- Flags
- [in] Combination of one or more D3DCLEAR flags
that specify the surface(s) that will be cleared.
- Color
- [in] Clear a render target to this ARGB color.
- Z
- [in] Clear the depth buffer to this new z value
which ranges from 0 to 1. See remarks.
- Stencil
- [in] Clear the stencil buffer to this new value
which ranges from 0 to 2n - 1 (n is the bit depth of the stencil
buffer). See remarks.
Return Values
If the method succeeds, the return value is D3D_OK. If
the method fails, the return value can be: D3DERR_INVALIDCALL.
Remarks
Use this method to clear a surface including: a render
target, all render targets in an MRT, a stencil buffer, or a depth buffer. Flags
determines how many surfaces are cleared. Use pRects to clear a subset of a
surface defined by an array of rectangles.
IDirect3DDevice9::Clear will fail if you:
- Try to clear either the depth buffer or the
stencil buffer of a render target that does not have an attached depth
buffer.
- Try to clear the stencil buffer when the depth
buffer does not contain stencil data.
D3DCLEAR
These flags identify a surface to reset when calling
IDirect3DDevice9::Clear.
#define |
Description |
D3DCLEAR_STENCIL |
Clear the
stencil buffer. |
D3DCLEAR_TARGET |
Clear a render
target, or all targets in a multiple render target. See Multiple Render
Targets (Direct3D 9). |
D3DCLEAR_ZBUFFER |
Clear the depth
buffer. |
获取Direct3D坐标变换矩阵
在Direct3D中,可以通过IDirect3DDevice9::GetTransform()获取当前的世界变换矩阵、观察变换矩阵以及投影变换矩阵,该函数声明如下:
Retrieves a matrix describing a transformation state.
HRESULT GetTransform(
D3DTRANSFORMSTATETYPE State,
D3DMATRIX * pMatrix
);
Parameters
- State
- [in] Device state variable that is being modified.
This parameter can be any member of the D3DTRANSFORMSTATETYPE enumerated
type, or the D3DTS_WORLDMATRIX macro.
- pMatrix
- [out] Pointer to a D3DMATRIX structure, describing
the returned transformation state.
Return Values
If the method succeeds, the return value is D3D_OK.
D3DERR_INVALIDCALL if one of the arguments is invalid.
Remarks
This method will not return device state for a device
that is created using D3DCREATE_PUREDEVICE. If you want to use this method, you
must create your device with any of the other flag values in D3DCREATE.