渲染顶点缓冲区图形
void render()
{
g_device->Clear(0, NULL, D3DCLEAR_TARGET, D3DCOLOR_X#050505, 1.0f, 0);
g_device->BeginScene();
g_device->SetStreamSource(0, g_vertex_buffer, 0, sizeof(sCustomVertex));
g_device->SetFVF(D3DFVF_CUSTOM_VERTEX);
g_device->DrawPrimitive(D3DPT_TRIANGLELIST, 0, 1);
g_device->EndScene();
g_device->Present(NULL, NULL, NULL, NULL);
}
函数IDirect3DDevice9::SetStreamSource()将顶点缓冲区和渲染数据流链接。
Binds a vertex buffer to a device data stream. For more
information, see Setting the Stream Source (Direct3D 9).
HRESULT SetStreamSource(
UINT StreamNumber,
IDirect3DVertexBuffer9 * pStreamData,
UINT OffsetInBytes,
UINT Stride
);
Parameters
- StreamNumber
- [in] Specifies the data stream, in the range from
0 to the maximum number of streams -1.
- pStreamData
- [in] Pointer to an IDirect3DVertexBuffer9
interface, representing the vertex buffer to bind to the specified data
stream.
- OffsetInBytes
- [in] Offset from the beginning of the stream to
the beginning of the vertex data, in bytes. To find out if the device
supports stream offsets, see the D3DDEVCAPS2_STREAMOFFSET constant in
D3DDEVCAPS2.
- Stride
- [in] Stride of the component, in bytes. See
Remarks.
Return Values
If the method succeeds, the return value is D3D_OK. If
the method fails, the return value can be D3DERR_INVALIDCALL.
Remarks
When a FVF vertex shader is used, the stride of the
vertex stream must match the vertex size, computed from the FVF. When a
declaration is used, the stride should be greater than or equal to the stream
size computed from the declaration.
When calling SetStreamSource, the stride is normally
required to be equal to the vertex size. However, there are times when you may
want to draw multiple instances of the same or similar geometry (such as when
using instancing to draw). For this case, use a zero stride to tell the runtime
not to increment the vertex buffer offset (ie: use the same vertex data for all
instances). For more information about instancing, see Efficiently Drawing
Multiple Instances of Geometry (Direct3D 9).
函数IDirect3DDevice9::SetFVF()的作用是声明当前的渲染数据流中的灵活顶点格式。
Sets the current vertex stream declaration.
HRESULT SetFVF(
DWORD FVF
);
Parameters
- FVF
- [in] DWORD containing the fixed function vertex
type. For more information, see D3DFVF.
Return Values
If the method succeeds, the return value is D3D_OK. If
the method fails, the return value can be: D3DERR_INVALIDCALL.
Remarks
Here are the steps necessary to initialize and use
vertices that have a position, diffuse and specular color, and texture
coordinates:
- Define the custom vertex type and FVF code.
struct LVertex
{
FLOAT x, y, z;
D3DCOLOR specular, diffuse;
FLOAT tu, tv;
};
const DWORD VertexFVF = (D3DFVF_XYZ | D3DFVF_DIFFUSE |
D3DFVF_SPECULAR | D3DFVF_TEX1 );
- Create a vertex buffer with enough room for four
vertices using IDirect3DDevice9::CreateVertexBuffer.
g_d3dDevice->CreateVertexBuffer( 4*sizeof(LVertex),
D3DUSAGE_WRITEONLY, VertexFVF, D3DPOOL_DEFAULT, &pBigSquareVB, NULL );
- Set the values for each vertex.
LVertex * v;
pBigSquareVB->Lock( 0, 0, (BYTE**)&v, 0 );
v[0].x = 0.0f; v[0].y = 10.0; v[0].z = 10.0f;
v[0].diffuse = 0xffff0000;
v[0].specular = 0xff00ff00;
v[0].tu = 0.0f; v[0].tv = 0.0f;
v[1].x = 0.0f; v[1].y = 0.0f; v[1].z = 10.0f;
v[1].diffuse = 0xff00ff00;
v[1].specular = 0xff00ffff;
v[1].tu = 0.0f; v[1].tv = 0.0f;
v[2].x = 10.0f; v[2].y = 10.0f; v[2].z = 10.0f;
v[2].diffuse = 0xffff00ff;
v[2].specular = 0xff000000;
v[2].tu = 0.0f; v[2].tv = 0.0f;
v[3].x = 0.0f; v[3].y = 10.0f; v[3].z = 10.0f;
v[3].diffuse = 0xffffff00;
v[3].specular = 0xffff0000;
v[3].tu = 0.0f; v[3].tv = 0.0f;
pBigSquareVB->Unlock();
- The vertex buffer has been initialized and is
ready to render. The following code example shows how to use the legacy FVF
to draw a square.
g_d3dDevice->SetFVF(VertexFVF);
g_d3dDevice->SetStreamSource(0, pBigSquareVB, 0, sizeof(LVertex));
g_d3dDevice->DrawPrimitive(D3DPT_TRIANGLESTRIP, 0 ,2);
Here are the steps necessary to initialize and use
vertices that have a position, a normal, and texture coordinates:
- Define the custom vertex type and FVF code.
struct Vertex
{
FLOAT x, y, z;
FLOAT nx, ny, nz;
FLOAT tu, tv;
};
const DWORD VertexFVF = ( D3DFVF_XYZ | D3DFVF_NORMAL | D3DFVF_TEX1 );
- Create a vertex buffer with enough room for four
vertices using IDirect3DDevice9::CreateVertexBuffer (similar to the
example above).
- Set the values for each vertex.
Vertex * v;
pBigSquareVB->Lock(0, 0, (BYTE**)&v, 0);
v[0].x = 0.0f; v[0].y = 10.0; v[0].z = 10.0f;
v[0].nx = 0.0f; v[0].ny = 1.0f; v[0].nz = 0.0f;
v[0].tu = 0.0f; v[0].tv = 0.0f;
v[1].x = 0.0f; v[1].y = 0.0f; v[1].z = 10.0f;
v[1].nx = 0.0f; v[1].ny = 1.0f; v[1].nz = 0.0f;
v[1].tu = 0.0f; v[1].tv = 0.0f;
v[2].x = 10.0f; v[2].y = 10.0f; v[2].z = 10.0f;
v[2].nx = 0.0f; v[2].ny = 1.0f; v[2].nz = 0.0f;
v[2].tu = 0.0f; v[2].tv = 0.0f;
v[3].x = 0.0f; v[3].y = 10.0f; v[3].z = 10.0f;
v[3].nx = 0.0f; v[3].ny = 1.0f; v[3].nz = 0.0f;
v[3].tu = 0.0f; v[3].tv = 0.0f;
pBigSquareVB->Unlock();
- Draw the object (similar to the example above).
函数IDirect3DDevice9::DrawPrimitive()用来绘制当前的渲染数据流中的图元。
示例程序VertexBuffer的完整源码如下:
#include <d3d9.h>
#define CLASS_NAME "GameApp"
#define release_com(p) do { if(p) { (p)->Release(); (p) = NULL; } } while(0)
IDirect3D9* g_d3d;
IDirect3DDevice9* g_device;
IDirect3DVertexBuffer9* g_vertex_buffer;
struct sCustomVertex
{
float x, y, z, rhw;
DWORD color;
};
#define D3DFVF_CUSTOM_VERTEX (D3DFVF_XYZRHW | D3DFVF_DIFFUSE)
void init_vb()
{
sCustomVertex vertices[] =
{
{ 100.0f, 400.0f, 1.0f, 1.0f, 0xffffff00, },
{ 300.0f, 50.0f, 1.0f, 1.0f, 0xff00ff00, },
{ 500.0f, 400.0f, 1.0f, 1.0f, 0xffff00ff, },
};
g_device->CreateVertexBuffer(sizeof(vertices), 0, D3DFVF_CUSTOM_VERTEX, D3DPOOL_DEFAULT, &g_vertex_buffer, NULL);
void* ptr;
g_vertex_buffer->Lock(0, sizeof(vertices), (void**)&ptr, 0);
memcpy(ptr, vertices, sizeof(vertices));
g_vertex_buffer->Unlock();
}
bool init_d3d(HWND hwnd)
{
g_d3d = Direct3DCreate9(D3D_SDK_VERSION);
if(g_d3d == NULL)
return false;
D3DPRESENT_PARAMETERS d3dpp;
ZeroMemory(&d3dpp, sizeof(d3dpp));
d3dpp.Windowed = TRUE;
d3dpp.SwapEffect = D3DSWAPEFFECT_DISCARD;
d3dpp.BackBufferFormat = D3DFMT_UNKNOWN;
if(FAILED(g_d3d->CreateDevice(D3DADAPTER_DEFAULT, D3DDEVTYPE_HAL, hwnd, D3DCREATE_SOFTWARE_VERTEXPROCESSING,
&d3dpp, &g_device)))
{
return false;
}
init_vb();
return true;
}
void cleanup()
{
release_com(g_vertex_buffer);
release_com(g_device);
release_com(g_d3d);
}
void render()
{
g_device->Clear(0, NULL, D3DCLEAR_TARGET, D3DCOLOR_XRGB(5, 5, 5), 1.0f, 0);
g_device->BeginScene();
g_device->SetStreamSource(0, g_vertex_buffer, 0, sizeof(sCustomVertex));
g_device->SetFVF(D3DFVF_CUSTOM_VERTEX);
g_device->DrawPrimitive(D3DPT_TRIANGLELIST, 0, 1);
g_device->EndScene();
g_device->Present(NULL, NULL, NULL, NULL);
}
LRESULT WINAPI WinProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch(msg)
{
case WM_KEYDOWN:
if(wParam == VK_ESCAPE)
DestroyWindow(hwnd);
break;
case WM_DESTROY:
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, msg, wParam, lParam);
}
int WINAPI WinMain(HINSTANCE inst, HINSTANCE, LPSTR, INT)
{
WNDCLASSEX wc;
wc.cbSize = sizeof(WNDCLASSEX);
wc.style = CS_CLASSDC;
wc.lpfnWndProc = WinProc;
wc.cbClsExtra = 0;
wc.cbWndExtra = 0;
wc.hInstance = inst;
wc.hIcon = NULL;
wc.hCursor = NULL;
wc.hbrBackground = NULL;
wc.lpszMenuName = NULL;
wc.lpszClassName = CLASS_NAME;
wc.hIconSm = NULL;
if(! RegisterClassEx(&wc))
return -1;
HWND hwnd = CreateWindow(CLASS_NAME, "Direct3D App", WS_OVERLAPPEDWINDOW, 200, 100, 600, 500,
NULL, NULL, wc.hInstance, NULL);
if(hwnd == NULL)
return -1;
if(init_d3d(hwnd))
{
ShowWindow(hwnd, SW_SHOWDEFAULT);
UpdateWindow(hwnd);
MSG msg;
ZeroMemory(&msg, sizeof(msg));
while(msg.message != WM_QUIT)
{
if(PeekMessage(&msg, NULL, 0, 0, PM_REMOVE))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
render();
}
}
cleanup();
UnregisterClass(CLASS_NAME, wc.hInstance);
return 0;
}
运行截图:
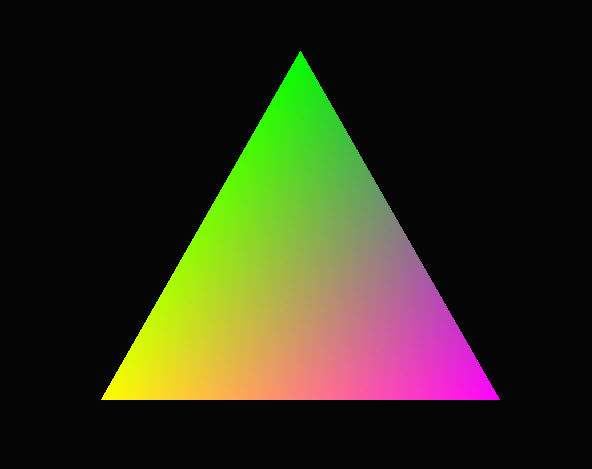