Updating the Skinned Mesh
When your skeletal structure is in the pose you desire, it's time to update
(or rebuild) the skinned mesh to match. Before you rebuild the skinned mesh, you
must make sure you have constructed the secondary mesh container and updated the
frame hierarchy. To review how to construct the mesh container, consult the
"Creating a Secondary Mesh Container" section earlier in this chapter. To
refresh your memory about how to update the frame hierarchy, review the
"Updating the Hierarchy" section earlier in this chapter. After you're sure of
these two things, you can continue.
To update the skinned mesh, you must first lock the vertex buffers of the
skinned mesh and the secondary mesh. This is critical because DirectX will pull
the vertex data from the skinned mesh object, apply the bone transformations,
and write the resulting vertex data to the secondary mesh object.
First, though, you need to copy the transformations from the frames to the
array of matrices(pBoneMatrices) stored in the mesh container. At the same time,
you have to combine the transformations with the bones' inversed
transformations. The inversed bone transformations are responsible for moving
the mesh's vertices to the origin of the mesh before you apply the actual
transformation. To better understand this, take a look at Figure 4.4
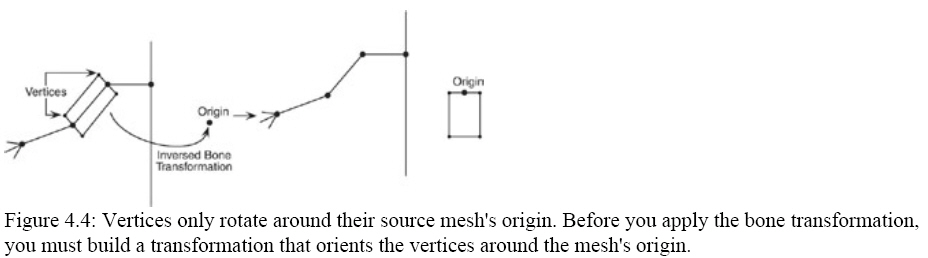
The mesh in Figure 4.4 is composed of three bones (frames) and a number of
vertices. To apply a transformation to any frame, you must move the vertices
belonging to the frame to the origin and then apply the transformations.
You move the vertices around the origin of the mesh before you apply a
transformation because a rotation matrix simply rotates vertices around an
origin. If you were to rotate a vertex belonging to any bone, the vertex would
rotate around the origin of the mesh instead of the bone's joint. For example,
if your body was a mesh and you bent your elbow, the vertices constructing your
arm's mesh would rotate around your elbow, not the center of your body. After
the vertices are moved to the center of the mesh, the transformation is applied
(thus rotating the vertices to match the rotation of the bone) and finally
translated into position.
Normally, these inversed bone transformations are stored in the .X file by
the 3D modeler used to create the meshes. If you don't have access to this
information from an .X file, you can compute it yourself by first updating the
frame hierarchy, and then inverting each frame's combined transformation using
the D3DXMatrixInverse function. Here's a quick example.
// pRoot = root D3DXFRAME_EX object
// pMesh = D3DXMESHCONTAINER_EX object w/mesh data
// Update the frame hierarchy
pRoot−>UpdateHierarchy();
// Go through each bone and calculate the inverse
for(DWORD i=0;i<NumBones;i++)
{
// Grab the transformation using the bone matrix
D3DXMATRIX matBone = (*pMesh−>ppFrameMatrices);
// Invert the matrix
D3DXMatrixInverse(&matBone, NULL, &matBone);
// Store the inversed bone transformation somewhere
}
Instead of going through all the trouble of calculating the inversed bone
transformations yourself, however, you can rely on the skinned mesh object to
supply that information. By calling ID3DXSkinInfo::GetBoneOffsetMatrix, you'll
get the inversed bone transformation matrix pointer. Multiply this matrix by a
frame transformation matrix, and you're set!
Using what you just learned, iterate through all the bones, grab the inversed
bone transformation, combine it with the frame transformation, and store the
result in the pBoneMatrices array.
for(DWORD i=0;i<pSkinInfo−>GetNumBones();i++)
{
// Set the inversed bone transformation
pMesh−>pBoneMatrices[i]=(*pSkinInfo−>GetBoneOffsetMatrix(i));
// Apply frame transformation
if(pMesh−>ppFrameMatrices[i])
pMesh−>pBoneMatrices[i] *= (*pMesh−>ppFrameMatrices[i]);
}
Now that you've copied the bones' transformations into the pBoneMatrices
array, you can move on to updating the skinned mesh by first locking the vertex
buffers for the skinned mesh and the secondary mesh.
// pSkinMesh = skinned mesh container
// pMesh = secondary mesh container
// Lock the meshes' vertex buffers
void *SrcPtr, *DestPtr;
pSkinMesh−>LockVertexBuffer(D3DLOCK_READONLY,(void**)&SrcPtr);
pMesh−>LockVertexBuffer(0, (void**)&DestPtr);
After you lock the vertex buffers, you need to perform a call to
ID3DXSkinInfo::UpdateSkinnedMesh to apply all the bones' transformations to the
vertices and write the resulting data to the secondary mesh container.
Applies software skinning to the target vertices based
on the current matrices.
HRESULT UpdateSkinnedMesh(
CONST D3DXMATRIX * pBoneTransforms,
CONST D3DXMATRIX * pBoneInvTransposeTransforms,
LPCVOID pVerticesSrc,
PVOID pVerticesDst
);
Parameters
- pBoneTransforms
- [in] Bone transform matrix.
- pBoneInvTransposeTransforms
- [in] Inverse transpose of the bone transform
matrix.
- pVerticesSrc
- [in] Pointer to the buffer containing the source
vertices.
- pVerticesDst
- [in] Pointer to the buffer containing the
destination vertices.
Return Values
If the method succeeds, the return value is D3D_OK. If
the method fails, the return value can be D3DERR_INVALIDCALL.
Remarks
When used to skin vertices with two position elements,
this method skins the second position element with the inverse of the bone
instead of the bone itself.
To finish, you simply unlock the vertex buffers, and you're ready to render!
// pSkinInfo = skinned mesh info object
// Update the skinned mesh using provided transformations
pSkinInfo−>UpdateSkinnedMesh(pBoneMatrices, NULL, SrcPtr, DestPtr);
// Unlock the meshes vertex buffers
pSkinMesh−>UnlockVertexBuffer();
pMesh−>UnlockVertexBuffer();
Rendering the Skinned Mesh
Now comes the good part−rendering your secondary mesh and showing the world
what it's like to play with powerthe power of skeletal animation and skinned
meshes, that is. You only need to depend on the typical mesh−rendering functions
to render the secondary mesh. Loop through each material, set the material and
texture, and call the ID3DXMesh::DrawSubset function. Loop and continue until
all of the subsets have been drawn.
// pMesh = D3DXMESHCONTAINER_EX object with material data
// pMeshToDraw = secondary mesh pointer to render
for(DWORD i=0;i<pMesh−>NumMaterials;i++)
{
// Set material and texture
pD3DDevice−>SetMaterial(&pMesh−>pMaterials[i].MatD3D);
pD3DDevice−>SetTexture(0, pMesh−>pTextures[i]);
// Draw the mesh subset
pMeshToDraw−>DrawSubset(i);
}