新建网页 1
SetMatrixTranspose—Used to set a transposed 4 × 4 matrix. Sample call:
D3DXMATRIX M(…);
D3DXMatrixTranspose(&M, &M);
ConstTable->SetMatrixTranspose(Device, handle, &M);
|
Sets a transposed matrix.
HRESULT SetMatrixTranspose( LPDIRECT3DDEVICE9 pDevice, D3DXHANDLE hConstant, CONST D3DXMATRIX* pMatrix);
Parameters
- pDevice
- [in] Pointer to an IDirect3DDevice9 interface, representing the device associated with the constant table.
- hConstant
- [in] Unique identifier to the matrix of constants.
- pMatrix
- [in] Pointer to a transposed matrix.
Return Values
If the method succeeds, the return value is D3D_OK. If the method fails, the return value can be D3DERR_INVALIDCALL.
SetMatrixTransposeArray—Used to set an array of 4 × 4 transposed matrices. Sample call:
D3DXMATRIX M[4];
// ...Initialize matrices and transpose them.
ConstTable->SetMatrixTransposeArray(Device, handle, M, 4);
|
Sets an array of transposed matrices.
HRESULT SetMatrixTransposeArray( LPDIRECT3DDEVICE9 pDevice, D3DXHANDLE hConstant,
CONST D3DXMATRIX* pMatrix, UINT Count);
Parameters
- pDevice
- [in] Pointer to an IDirect3DDevice9 interface, representing the device associated with the constant table.
- hConstant
- [in] Unique identifier to the array of matrix constants.
- pMatrix
- [in] Array of transposed matrices.
- Count
- [in] Number of matrices in the array.
Return Values
If the method succeeds, the return value is D3D_OK. If the method fails, the return value can be D3DERR_INVALIDCALL.
SetMatrixTransposePointerArray—Used to set an array of pointers to 4 × 4 transposed matrices. Sample call:
D3DXMATRIX* M[4];
// ...Allocate,initialize matrix pointers and transpose them.
ConstTable->SetMatrixTransposePointerArray(Device, handle, M, 4);
|
Sets an array of pointers to transposed matrices.
HRESULT SetMatrixTransposePointerArray( LPDIRECT3DDEVICE9 pDevice, D3DXHANDLE hConstant,
CONST D3DXMATRIX** ppMatrix, UINT Count);
Parameters
- pDevice
- [in] Pointer to an IDirect3DDevice9 interface, representing the device associated with the constant table.
- hConstant
- [in] Unique identifier to the array of matrix constants.
- ppMatrix
- [in] Array of pointers to transposed matrices.
- Count
- [in] Number of matrices in the array.
Return Values
If the method succeeds, the return value is D3D_OK. If the method fails, the return value can be D3DERR_INVALIDCALL.
Remarks
A transposed matrix contains column-major data; that is, each vector is contained in a column.
SetVector—Used to set a variable of type D3DXVECTOR4. Sample call:
D3DXVECTOR4 v(1.0f, 2.0f, 3.0f, 4.0f);
ConstTable->SetVector(Device, handle, &v);
|
Sets a 4D vector.
HRESULT SetVector( LPDIRECT3DDEVICE9 pDevice, D3DXHANDLE hConstant, CONST D3DXVECTOR4* pVector);
Parameters
- pDevice
- [in] Pointer to an IDirect3DDevice9 interface, representing the device associated with the constant table.
- hConstant
- [in] Unique identifier to the vector constant.
- pVector
- [in] Pointer to a 4D vector.
Return Values
If the method succeeds, the return value is D3D_OK. If the method fails, the return value can be D3DERR_INVALIDCALL.
SetVectorArray—Used to set a variable that is a vector array. Sample call:
D3DXVECTOR4 v[3];
// ...Initialize vectors
ConstTable->SetVectorArray(Device, handle, v, 3);
|
Sets an array of 4D vectors.
HRESULT SetVectorArray( LPDIRECT3DDEVICE9 pDevice, D3DXHANDLE hConstant,
CONST D3DXVECTOR4* pVector, UINT Count);
Parameters
- pDevice
- [in] Pointer to an IDirect3DDevice9 interface, representing the device associated with the constant table.
- hConstant
- [in] Unique identifier to the array of vector constants.
- pVector
- [in] Array of 4D vectors.
- Count
- [in] Number of vectors in the array.
Return Values
If the method succeeds, the return value is D3D_OK. If the method fails, the return value can be D3DERR_INVALIDCALL.
SetValue—Used to set an arbitrarily sized type, such as a structure. In the sample call, we use SetValue to set a D3DXMATRIX:
D3DXMATRIX M(…);
ConstTable->SetValue(Device, handle, (void*)&M, sizeof(M));
|
Sets the contents of the buffer to the constant table.
HRESULT SetValue( LPDIRECT3DDEVICE9 pDevice, D3DXHANDLE hConstant, LPCVOID pData, UINT Bytes);
Parameters
- pDevice
- [in] Pointer to an IDirect3DDevice9 interface, representing the device associated with the constant table.
- hConstant
- [in] Unique identifier to a constant.
- pData
- [in] Buffer containing data.
- Bytes
- [in] Size of the buffer, in bytes.
Return Values
If the method succeeds, the return value is D3D_OK. If the method fails, the return value can be D3DERR_INVALIDCALL.
16.2.1.3 设置常量默认值
下一个方法就是设置常量的默认值,这些默认值在声明时初始化。这个方法应该在应用程序建立(setup)期间被调用一次
(called once)。
HRESULT ID3DXConstantTable::SetDefaults(
LPDIRECT3DDEVICE9 pDevice
);
|
pDevice——关联到常量表的设备的指针。
Sets the constants to their default values. The default values are declared in the variable declarations in the shader.
HRESULT SetDefaults( LPDIRECT3DDEVICE9 pDevice);
Parameters
- pDevice
- [in] Pointer to an IDirect3DDevice9 interface, representing the device associated with the constant table.
Return Values
If the method succeeds, the return value is D3D_OK. If the method fails, the return value can be D3DERR_INVALIDCALL.
16.2.2 编译HLSL着色器
我们可以编译一个着色器——用我们已保存的着色器的文本文件——使用下列函数:
HRESULT D3DXCompileShaderFromFile(
LPCSTR pSrcFile,
CONST D3DXMACRO* pDefines,
LPD3DXINCLUDE pInclude,
LPCSTR pFunctionName,
LPCSTR pTarget,
DWORD Flags,
LPD3DXBUFFER* ppShader,
LPD3DXBUFFER* ppErrorMsgs,
LPD3DXCONSTANTTABLE* ppConstantTable
);
|
pSrcFile——要编译的包含着色器源代码的文本文件的文件名
pDefines——参数可选,这里指定为空。
pInclude——ID3DXInclude接口指针。这个接口被设计成由应用程序实现,所以我们可以重载默认include的行为。通常默认行为就可以了,而且我们可以通过将其指定为空忽略此参数。
pFunctionName——指定入口点函数名的字符串。例如,如果着色器的入口点函数叫做Main,我们可以给此参数传递“Main”。
pTarget——指定要编译成的HLSL着色器源文件的版本的字符串。有效的顶点着色器版本是:vs_1_1, vs_2_0, vs_2_sw。有效的像素着色器版本是2.0,我们可以给此参数传递vs_2_0。
备注:有编译不同版本着色器的能力,是HLSL与汇编语言比的主要优势。用HLSL我们只需为需要的目标简单的重新编译,便可快速移植着色器到不同的版本。使用汇编,我们可能需要手动移植代码。
Flags——可选的编译标记,指定为0标识没有标记。有效的选项是:
D3DXSHADER_DEBUG——通知编译器写入调试信息
D3DXSHADER_SKIPVALIDATION——通知编译器不要做任何代码检查。此项仅用于你已知着色器能够工作时。
D3DXSHADER_SKIPOPTIMIZATION——通知编译器不要执行任何代码优化。实践中,这个选项应该仅用于调试,因为这种情况下你不希望编译器以任何方式修改代码。
ppShader——返回已编译的着色器代码的ID3DXBuffer指针。这个已编译过的着色器代码将作为另一个实际创建顶点/像素着色器函数的参数。
ppErrorMsgs——返回包含错误码和错误消息字符串的ID3DXBuffer指针
ppConstantTable——返回包含此着色器常量表数据的ID3DXConstantTable指针
Compile a shader file.
HRESULT D3DXCompileShaderFromFile( LPCSTR pSrcFile, CONST D3DXMACRO* pDefines, LPD3DXINCLUDE pInclude,
LPCSTR pFunctionName, LPCSTR pProfile, DWORD Flags, LPD3DXBUFFER* ppShader,
LPD3DXBUFFER * ppErrorMsgs, LPD3DXCONSTANTTABLE * ppConstantTable);
Parameters
- pSrcFile
- [in] Pointer to a string that specifies the filename.
- pDefines
- [in] An optional NULL terminated array of D3DXMACRO structures. This value may be NULL.
- pInclude
- [in] Optional interface pointer, ID3DXInclude, to use for handling #include directives. If this value is NULL, #includes will either be honored when compiling from a file or will cause an error when compiled from a resource or memory.
- pFunctionName
- [in] Pointer to the shader entry point function where execution begins.
- pProfile
- [in] Pointer to a shader profile which determines the shader instruction set. See D3DXGetVertexShaderProfile or D3DXGetPixelShaderProfile for a list of the profiles available.
- Flags
- [in] Compile options identified by various flags. The Direct3D 10 HLSL compiler is now the default. See D3DXSHADER Flags for details.
- ppShader
- [out] Returns a buffer containing the created shader. This buffer contains the compiled shader code, as well as any embedded debug and symbol table information.
- ppErrorMsgs
- [out] Returns a buffer containing a listing of errors and warnings that were encountered during the compile. These are the same messages the debugger displays when running in debug mode. This value may be NULL.
- ppConstantTable
- [out] Returns an ID3DXConstantTable interface, which can be used to access shader constants. This value may be NULL.
Return Values
If the function succeeds, the return value is D3D_OK. If the function fails, the return value can be one of the following: D3DERR_INVALIDCALL, D3DXERR_INVALIDDATA, E_OUTOFMEMORY.
这里是一个调用D3DXCompileShaderFromFile的例子:
// compile shader
ID3DXBuffer* shader_buffer;
ID3DXBuffer* error_buffer;
HRESULT hr = D3DXCompileShaderFromFile("VertexShader.cxx", NULL, NULL, "main", "vs_1_1", D3DXSHADER_DEBUG,
&shader_buffer, &error_buffer, &g_constant_table);
// output any error messages
if(error_buffer)
{
MessageBox(NULL, (char*)error_buffer->GetBufferPointer(), "ERROR", MB_OK);
safe_release<ID3DXBuffer*>(error_buffer);
}
if(FAILED(hr))
{
MessageBox(NULL, "D3DXCreateEffectFromFile() - FAILED", "ERROR", MB_OK);
return false;
}
示例截图:
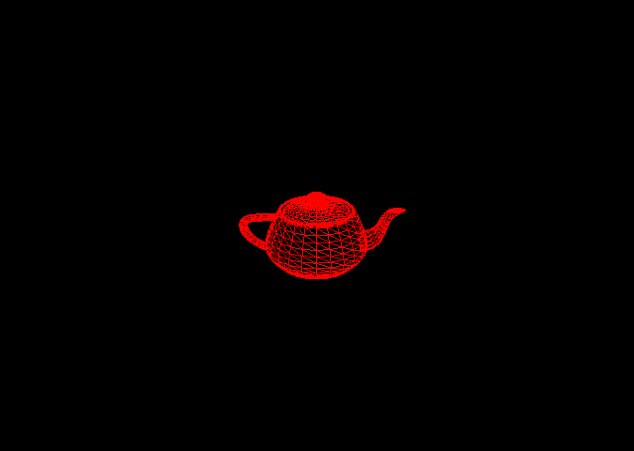
执行程序:
/**************************************************************************************************
Demonstrates transforming an object by the view and projection matrices, and setting
the vertex color using a vertex shader.
You will have to switch to the REF device if your hardware does not support shaders.
Or you can use software vertex processing: D3DCREATE_SOFTWARE_VERTEXPROCESSING.
**************************************************************************************************/
#include "d3dUtility.h"
#pragma warning(disable : 4100)
const int WIDTH = 640;
const int HEIGHT = 480;
IDirect3DDevice9* g_device;
ID3DXMesh* g_teapot;
IDirect3DVertexShader9* g_vertex_shader;
ID3DXConstantTable* g_constant_table;
D3DXHANDLE g_view_proj_handle;
D3DXMATRIX g_proj_matrix;
////////////////////////////////////////////////////////////////////////////////////////////////////
bool setup()
{
D3DXCreateTeapot(g_device, &g_teapot, NULL);
// compile shader
ID3DXBuffer* shader_buffer;
ID3DXBuffer* error_buffer;
HRESULT hr = D3DXCompileShaderFromFile("VertexShader.cxx", NULL, NULL, "main", "vs_1_1", D3DXSHADER_DEBUG,
&shader_buffer, &error_buffer, &g_constant_table);
// output any error messages
if(error_buffer)
{
MessageBox(NULL, (char*)error_buffer->GetBufferPointer(), "ERROR", MB_OK);
safe_release<ID3DXBuffer*>(error_buffer);
}
if(FAILED(hr))
{
MessageBox(NULL, "D3DXCreateEffectFromFile() - FAILED", "ERROR", MB_OK);
return false;
}
hr = g_device->CreateVertexShader((DWORD*) shader_buffer->GetBufferPointer(), &g_vertex_shader);
if(FAILED(hr))
{
MessageBox(NULL, "CreateVertexShader - FAILED", "ERROR", MB_OK);
return false;
}
safe_release<ID3DXBuffer*>(shader_buffer);
g_view_proj_handle = g_constant_table->GetConstantByName(NULL, "g_view_proj_matrix");
g_constant_table->SetDefaults(g_device);
// set the projection matrix
D3DXMatrixPerspectiveFovLH(&g_proj_matrix, D3DX_PI/4.0f, (float)WIDTH/HEIGHT, 1.0f, 1000.0f);
g_device->SetRenderState(D3DRS_FILLMODE, D3DFILL_WIREFRAME);
return true;
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
void cleanup()
{
safe_release<ID3DXMesh*>(g_teapot);
safe_release<IDirect3DVertexShader9*>(g_vertex_shader);
safe_release<ID3DXConstantTable*>(g_constant_table);
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
bool display(float time_delta)
{
static float angle = (3.0f * D3DX_PI) / 2.0f;
static float height = 5.0f;
if(GetAsyncKeyState(VK_LEFT) & 0x8000f)
angle -= 0.5f * time_delta;
if(GetAsyncKeyState(VK_RIGHT) & 0x8000f)
angle += 0.5f * time_delta;
if(GetAsyncKeyState(VK_UP) & 0x8000f)
height += 5.0f * time_delta;
if(GetAsyncKeyState(VK_DOWN) & 0x8000f)
height -= 5.0f * time_delta;
D3DXVECTOR3 position(cosf(angle) * 10.0f, height, sinf(angle) * 10.0f);
D3DXVECTOR3 target(0.0f, 0.0f, 0.0f);
D3DXVECTOR3 up(0.0f, 1.0f, 0.0f);
D3DXMATRIX view_matrix;
D3DXMatrixLookAtLH(&view_matrix, &position, &target, &up);
D3DXMATRIX view_proj_matrix = view_matrix * g_proj_matrix;
g_constant_table->SetMatrix(g_device, g_view_proj_handle, &view_proj_matrix);
// render now
g_device->Clear(0, NULL, D3DCLEAR_TARGET | D3DCLEAR_ZBUFFER, 0x00000000, 1.0f, 0);
g_device->BeginScene();
g_device->SetVertexShader(g_vertex_shader);
g_teapot->DrawSubset(0);
g_device->EndScene();
g_device->Present(NULL, NULL, NULL, NULL);
return true;
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
LRESULT CALLBACK wnd_proc(HWND hwnd, UINT msg, WPARAM word_param, LPARAM long_param)
{
switch(msg)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
case WM_KEYDOWN:
if(word_param == VK_ESCAPE)
DestroyWindow(hwnd);
break;
}
return DefWindowProc(hwnd, msg, word_param, long_param);
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
int WINAPI WinMain(HINSTANCE inst, HINSTANCE, PSTR cmd_line, int cmd_show)
{
if(! init_d3d(inst, WIDTH, HEIGHT, true, D3DDEVTYPE_HAL, &g_device))
{
MessageBox(NULL, "init_d3d() - failed.", 0, MB_OK);
return 0;
}
if(! setup())
{
MessageBox(NULL, "Steup() - failed.", 0, MB_OK);
return 0;
}
enter_msg_loop(display);
cleanup();
g_device->Release();
return 0;
}
下载源程序