ID3DXMesh接口的主要功能继承自ID3DXBaseMesh父接口,了解这些是很重要的,其它一些mesh接口如ID3DXPMesh也是继承自ID3DXBaseMesh。
ID3DXBaseMesh接口包含一个用来存储网格顶点的顶点缓存和一个用来定义这些顶点怎样连接在一起组成网格三角形的索引缓存。我们使用下面的方法来得到这些缓存的指针:
HRESULT
ID3DXMesh::GetVertexBuffer(LPDIRECT3DVERTEXBUFFER9* ppVB);
HRESULT
ID3DXMesh::GetIndexBuffer(LPDIRECT3DINDEXBUFFER9* ppIB);
|
这里有一些使用这些方法的例子:
IDirect3DVertexBuffer9* vb
= 0;
Mesh->GetVertexBuffer( &vb
);
IDirect3DIndexBuffer9* ib =
0;
Mesh->GetIndexBuffer( &ib
);
|
假如想锁定这些缓存来读写数据,那么我们能够使用下面的方法。注意这些方法锁定整个顶点/索引缓存。
Locks a vertex buffer and obtains a pointer to the
vertex buffer memory.
HRESULT LockVertexBuffer(
DWORD Flags,
LPVOID * ppData
);
Parameters
- Flags
Combination of zero or more locking flags that
describe the type of lock to perform. For this method, the valid flags are:
- D3DLOCK_DISCARD
- D3DLOCK_NO_DIRTY_UPDATE
- D3DLOCK_NOSYSLOCK
- D3DLOCK_READONLY
- D3DLOCK_NOOVERWRITE
- [in] For a description of the flags, see D3DLOCK.
- ppData
- [out, retval] VOID* pointer to a buffer containing
the vertex data.
Return Values
If the method succeeds, the return value is D3D_OK. If
the method fails, the return value can be D3DERR_INVALIDCALL.
Remarks
When working with vertex buffers, you are allowed to
make multiple lock calls; however, you must ensure that the number of lock calls
match the number of unlock calls. DrawPrimitive calls will not succeed with any
outstanding lock count on any currently set vertex buffer.
Locks an index buffer and obtains a pointer to the
index buffer memory.
HRESULT LockIndexBuffer(
DWORD Flags,
LPVOID * ppData
);
Parameters
- Flags
Combination of zero or more locking flags that
describe the type of lock to perform. For this method, the valid flags are:
- D3DLOCK_DISCARD
- D3DLOCK_NO_DIRTY_UPDATE
- D3DLOCK_NOSYSLOCK
- D3DLOCK_READONLY
- [in] For a description of the flags, see D3DLOCK.
- ppData
- [out, retval] VOID* pointer to a buffer containing
the index data. The count of indices in this buffer will be equal to
ID3DXBaseMesh::GetNumFaces * 3.
Return Values
If the method succeeds, the return value is D3D_OK. If
the method fails, the return value can be D3DERR_INVALIDCALL.
Remarks
When working with index buffers, you are allowed to
make multiple lock calls. However, you must ensure that the number of lock calls
match the number of unlock calls. DrawPrimitive calls will not succeed with any
outstanding lock count on any currently set index buffer.
D3DLOCK
A combination of zero or more locking options that
describe the type of lock to perform.
#define |
Description |
D3DLOCK_DISCARD |
The application discards all memory within the
locked region. For vertex and index buffers, the entire buffer will be
discarded. This option is only valid when the resource is created with
dynamic usage (see D3DUSAGE). |
D3DLOCK_DONOTWAIT |
Allows an application to gain back CPU cycles
if the driver cannot lock the surface immediately. If this flag is set
and the driver cannot lock the surface immediately, the lock call will
return D3DERR_WASSTILLDRAWING. This flag can only be used when locking a
surface created using IDirect3DDevice9::CreateOffscreenPlainSurface,
IDirect3DDevice9::CreateRenderTarget, or
IDirect3DDevice9::CreateDepthStencilSurface. This flag can also be used
with a back buffer. |
D3DLOCK_NO_DIRTY_UPDATE |
By default, a lock on a resource adds a dirty
region to that resource. This option prevents any changes to the dirty
state of the resource. Applications should use this option when they
have additional information about the set of regions changed during the
lock operation. |
D3DLOCK_NOOVERWRITE |
Indicates that memory that was referred to in
a drawing call since the last lock without this flag will not be
modified during the lock. This can enable optimizations when the
application is appending data to a resource. Specifying this flag
enables the driver to return immediately if the resource is in use,
otherwise, the driver must finish using the resource before returning
from locking. |
D3DLOCK_NOSYSLOCK |
The default behavior of a video memory lock is
to reserve a system-wide critical section, guaranteeing that no display
mode changes will occur for the duration of the lock. This option causes
the system-wide critical section not to be held for the duration of the
lock.
The lock operation is time
consuming, but can enable the system to perform other duties, such as
moving the mouse cursor. This option is useful for long-duration locks,
such as the lock of the back buffer for software rendering that would
otherwise adversely affect system responsiveness.
|
D3DLOCK_READONLY |
The application will not write to the buffer.
This enables resources stored in non-native formats to save the
recompression step when unlocking. |
当然在你锁定以后一定要记得解锁:
HRESULT
ID3DXMesh::UnlockVertexBuffer();
HRESULT
ID3DXMesh::UnlockIndexBuffer();
|
下面是另外一些与mesh几何结构有关的ID3DXMesh接口方法:
DWORD
GetFVF() — 返回顶点的格式
DWORD
GetNumVertices() —
返回顶点缓存中的顶点数
DWORD
GetNumBytesPerVertex() —
返回一个顶点所占的字节数
DWORD GetNumFaces()
— 返回在mesh中的面(三角形)数
一个mesh由一个或数个子集组成。一个子集(subset)是在mesh中的使用相同属性渲染的一组三角形。这里的属性是指材质,纹理和渲染状态。图10.1显示了一座房子mesh可能被分成的几个子集。
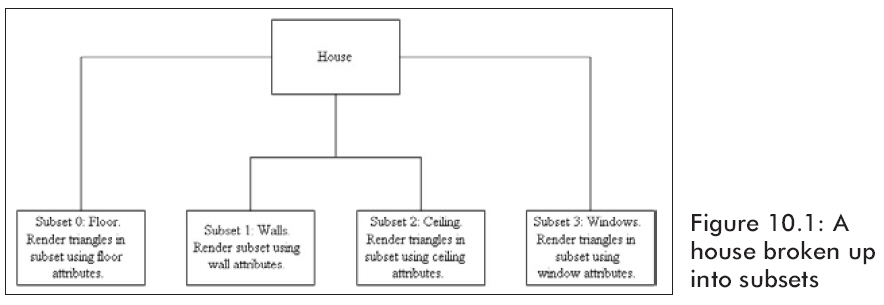
在mesh中的每个三角形都与一个属性ID相关联,表示该三角形属于该子集。例如,图10.1中组成地板的三角形具有属性ID0,它表示这些三角形属于子集0。同样,组成墙的三角形具有属性ID1,它表示这些三角形属于子集1。
三角形的属性ID存储在mesh的属性缓存中,它是一个DWORD数组。因为每个面对应属性缓存中的一项,所以属性缓存中的项目数等于mesh中的面的个数。属性缓存中的项目和索引缓存中定义的三角形一一对应。即,属性缓存中的第i项和索引缓存中的第i个三角形相对应。三角形i由下面三个索引缓存中的索引项定义:
A = i * 3
B = i * 3 + 1
C = i * 3 + 2
图10.2显示了这个对应关系:
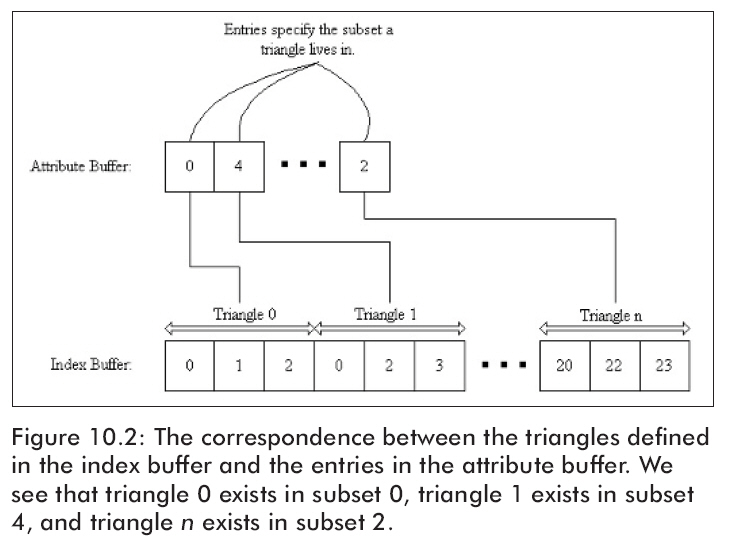
我们可以通过LockAttributeBuffer()锁定属性缓存:
Locks the mesh buffer that contains the mesh attribute
data, and returns a pointer to it.
HRESULT LockAttributeBuffer(
DWORD Flags,
DWORD ** ppData
);
Parameters
- Flags
Combination of zero or more locking flags that
describe the type of lock to perform. For this method, the valid flags are:
- D3DLOCK_DISCARD
- D3DLOCK_NO_DIRTY_UPDATE
- D3DLOCK_NOSYSLOCK
- D3DLOCK_READONLY
- [in] For a description of the flags, see D3DLOCK.
- ppData
- [out] Address of a pointer to a buffer containing
a DWORD for each face in the mesh.
Return Values
If the method succeeds, the return value is D3D_OK. If
the method fails, the return value can be D3DERR_INVALIDCALL.
Remarks
If ID3DXMesh::Optimize has been called, the mesh will
also have an attribute table that can be accessed using the
ID3DXBaseMesh::GetAttributeTable method.
就象下面的代码片段:
DWORD* buffer = 0;
Mesh->LockAttributeBuffer(lockingFlags, &buffer);
// Read or
write to attribute buffer...
Mesh->UnlockAttributeBuffer();
|
ID3DXMesh接口提供了DrawSubset(DWORD
AttribId)方法来绘制AttribId指示的子集中的各个三角形。
Draws a subset of a mesh.
HRESULT DrawSubset(
DWORD AttribId
);
Parameters
- AttribId
- [in] DWORD that specifies which subset of the mesh
to draw. This value is used to differentiate faces in a mesh as belonging to
one or more attribute groups.
Return Values
If the method succeeds, the return value is D3D_OK. If
the method fails, the return value can be D3DERR_INVALIDCALL.
Remarks
The subset that is specified by AttribId will be
rendered by the IDirect3DDevice9::DrawIndexedPrimitive method, using the
D3DPT_TRIANGLELIST primitive type, so an index buffer must be properly
initialized.
An attribute table is used to identify areas of the
mesh that need to be drawn with different textures, render states, materials,
and so on. In addition, the application can use the attribute table to hide
portions of a mesh by not drawing a given attribute identifier (AttribId)
when drawing the frame.
例如,要绘制子集0中的所有三角形,我们将这样写:
为了绘制整个mesh,我们必须绘制mesh的所有子集。这是非常方便的用0,1,2,…,n-1来标识子集,这里的n是子集的总数。且有一个相对应的材质和纹理数组,即子集i与材质和纹理数组的第i项对应。这就使我们能够简单的用循环来渲染mesh:
for(int
i = 0; i < numSubsets; i++)
{
Device->SetMaterial(
mtrls[i] );
Device->SetTexture(
0, textures[i] );
Mesh->DrawSubset(i);
}
|