最后的函数是被用来创建一个3D 文字网格,图9.1显示了实例“D3DXCreateText”所绘制的3D文本网格。
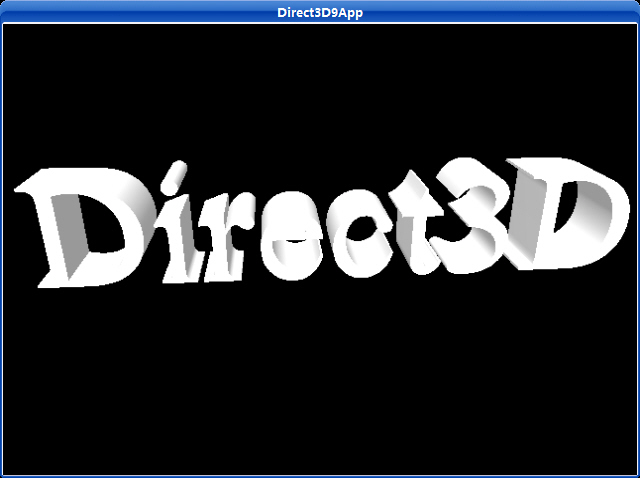
该函数的原型是:
HRESULT D3DXCreateText(
LPDIRECT3DDEVICE9 pDevice,
HDC hDC,
LPCTSTR pText,
FLOAT Deviation,
FLOAT Extrusion,
LPD3DXMESH* ppMesh,
LPD3DXBUFFER* ppAdjacency,
LPGLYPHMETRICSFLOAT pGlyphMetrics
);
|
这个函数如果调用成功则返回D3D_OK。
pDevice — 和mesh关联的device。
hDC — 我们将要用来产生mesh的包含描述字体的设备环境句柄。
pText — 指向以null结束的字符串的指针,此字符串是用来指定创建什么文字mesh。
Deviation — 字型轮廓段数间距,该值必须大于等于0。当它为0时,段数等于字体原始设计单位(该值越接近0,那么字体就越光滑)。
Extrusion — 文字在z轴方向的深度。
ppMesh — 返回创建的mesh。
ppAdjacency — 返回创建mesh的相关信息。假如你不需要它可以将其指定为null。
pGlyphMetrics — 一个指向LPGLYPHMETRICSFLOAT结构数组的指针,它包含了字型米数据。假如你不关心此数据,你可以把它设置为0。
来看一个示例,首先来看看其中用到的API函数使用说明:
The CreateCompatibleDC function creates a memory device context (DC) compatible with the specified device.
HDC CreateCompatibleDC(
HDC hdc // handle to DC
);
Parameters
- hdc
- [in] Handle to an existing DC. If this handle is NULL, the function creates a memory DC compatible with the application's current screen.
Return Values
If the function succeeds, the return value is the handle to a memory DC.
If the function fails, the return value is NULL.
Windows NT/2000/XP: To get extended error information, call GetLastError.
Remarks
A memory DC exists only in memory. When the memory DC is created, its display surface is exactly one monochrome pixel wide and one monochrome pixel high. Before an application can use a memory DC for drawing operations, it must select a bitmap of the correct width and height into the DC. To select a bitmap into a DC, use the CreateCompatibleBitmap function, specifying the height, width, and color organization required.
When a memory DC is created, all attributes are set to normal default values. The memory DC can be used as a normal DC. You can set the attributes; obtain the current settings of its attributes; and select pens, brushes, and regions.
The CreateCompatibleDC function can only be used with devices that support raster operations. An application can determine whether a device supports these operations by calling the GetDeviceCaps function.
When you no longer need the memory DC, call the DeleteDC function.
Windows 2000 and later: If hdc is NULL, the thread that calls CreateCompatibleDC owns the HDC that is created. When this thread is destroyed, the HDC is no longer valid. Thus, if you create the HDC andpass it to another thread, then exit the first thread, the second thread will not be able to use the HDC.
ICM: If the DC that is passed to this function is enabled for Independent Color Management (ICM), the DC created by the function is ICM-enabled. The source and destination color spaces are specified in the DC.
The CreateFontIndirect function creates a logical font that has the specified characteristics. The font can subsequently be selected as the current font for any device context.
HFONT CreateFontIndirect(
CONST LOGFONT* lplf // characteristics
);
Parameters
- lplf
- [in] Pointer to a LOGFONT structure that defines the characteristics of the logical font.
Return Values
If the function succeeds, the return value is a handle to a logical font.
If the function fails, the return value is NULL.
Windows NT/2000/XP: To get extended error information, call GetLastError.
Remarks
The CreateFontIndirect function creates a logical font with the characteristics specified in the LOGFONT structure. When this font is selected by using the SelectObject function, GDI's font mapper attempts to match the logical font with an existing physical font. If it fails to find an exact match, it provides an alternative whose characteristics match as many of the requested characteristics as possible.
To get the appropriate font on different language versions of the OS, call EnumFontFamiliesEx with the desired font characteristics in the LOGFONT structure, retrieve the appropriate typeface name, and create the font using CreateFont or CreateFontIndirect.
When you no longer need the font, call the DeleteObject function to delete it.
The SelectObject function selects an object into the specified device context (DC). The new object replaces the previous object of the same type.
HGDIOBJ SelectObject(
HDC hdc, // handle to DC
HGDIOBJ hgdiobj // handle to object
);
Parameters
- hdc
- [in] Handle to the DC.
- hgdiobj
- [in] Handle to the object to be selected. The specified object must have been created by using one of the following functions.
Return Values
If the selected object is not a region and the function succeeds, the return value is a handle to the object being replaced. If the selected object is a region and the function succeeds, the return value is one of the following values.
Value | Meaning |
SIMPLEREGION |
Region consists of a single rectangle. |
COMPLEXREGION |
Region consists of more than one rectangle. |
NULLREGION |
Region is empty. |
If an error occurs and the selected object is not a region, the return value is NULL. Otherwise, it is HGDI_ERROR.
Remarks
This function returns the previously selected object of the specified type. An application should always replace a new object with the original, default object after it has finished drawing with the new object.
An application cannot select a bitmap into more than one DC at a time.
ICM: If the object being selected is a brush or a pen, color management is performed.
主程序:
//////////////////////////////////////////////////////////////////////////////////////////////////
// Demonstrates how to create and render 3D text using D3DXCreateText.
//////////////////////////////////////////////////////////////////////////////////////////////////
#include "d3dUtility.h"
#pragma warning(disable : 4996)
const int WIDTH = 640;
const int HEIGHT = 480;
IDirect3DDevice9* g_d3d_device;
ID3DXMesh* g_text_mesh;
////////////////////////////////////////////////////////////////////////////////////////////////
bool Setup()
{
// Get a handle to a device context
HDC hdc = CreateCompatibleDC(NULL);
// Describe the font we want.
LOGFONT lf;
ZeroMemory(&lf, sizeof(LOGFONT));
lf.lfHeight = 25; // in logical units
lf.lfWidth = 12; // in logical units
lf.lfEscapement = 0;
lf.lfOrientation = 0;
lf.lfWeight = 500; // boldness, range 0(light) - 1000(bold)
lf.lfItalic = FALSE;
lf.lfUnderline = FALSE;
lf.lfStrikeOut = FALSE;
lf.lfCharSet = DEFAULT_CHARSET;
lf.lfOutPrecision = 0;
lf.lfClipPrecision = 0;
lf.lfQuality = 0;
lf.lfPitchAndFamily = 0;
strcpy(lf.lfFaceName, "Times New Roman"); // font style
// Create the font and select it with the device context.
HFONT hFont = CreateFontIndirect(&lf);
HFONT hFontOld = (HFONT)SelectObject(hdc, hFont);
// Create the text mesh based on the selected font in the HDC.
D3DXCreateText(g_d3d_device, hdc, "Direct3D", 0.001f, 0.4f, &g_text_mesh, NULL, NULL);
// Restore the old font and free the acquired HDC.
SelectObject(hdc, hFontOld);
DeleteObject(hFont);
DeleteDC(hdc);
// Lights
D3DXVECTOR3 dir(0.0f, -0.5f, 1.0f);
D3DXCOLOR col = WHITE;
D3DLIGHT9 light = InitDirectionalLight(&dir, &col);
g_d3d_device->SetLight(0, &light);
g_d3d_device->LightEnable(0, TRUE);
g_d3d_device->SetRenderState(D3DRS_NORMALIZENORMALS, TRUE);
g_d3d_device->SetRenderState(D3DRS_SPECULARENABLE, TRUE);
// Set camera
D3DXVECTOR3 pos(0.0f, 1.5f, -3.3f);
D3DXVECTOR3 target(0.0f, 0.0f, 0.0f);
D3DXVECTOR3 up(0.0f, 1.0f, 0.0f);
D3DXMATRIX V;
D3DXMatrixLookAtLH(&V, &pos, &target, &up);
g_d3d_device->SetTransform(D3DTS_VIEW, &V);
// Set projection matrix.
D3DXMATRIX proj;
D3DXMatrixPerspectiveFovLH(&proj, D3DX_PI * 0.25f, (float)WIDTH / HEIGHT, 0.01f, 1000.0f);
g_d3d_device->SetTransform(D3DTS_PROJECTION, &proj);
return true;
}
void Cleanup()
{
Release<ID3DXMesh*>(g_text_mesh);
}
bool Display(float timeDelta)
{
// Update: Spin the 3D text.
D3DXMATRIX y_rot_matrix, tran_matrix;
static float y = 0.0f;
D3DXMatrixRotationY(&y_rot_matrix, y);
y += timeDelta;
if(y >= 6.28f)
y = 0.0f;
D3DXMatrixTranslation(&tran_matrix, -1.6f, 0.0f, 0.0f);
tran_matrix *= y_rot_matrix;
g_d3d_device->SetTransform(D3DTS_WORLD, &tran_matrix);
// Render
g_d3d_device->Clear(0, NULL, D3DCLEAR_TARGET | D3DCLEAR_ZBUFFER, 0x00000000, 1.0f, 0);
g_d3d_device->BeginScene();
g_d3d_device->SetMaterial(&WHITE_MTRL);
g_text_mesh->DrawSubset(0);
g_d3d_device->EndScene();
g_d3d_device->Present(NULL, NULL, NULL, NULL);
return true;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch( msg )
{
case WM_DESTROY:
PostQuitMessage(0);
break;
case WM_KEYDOWN:
if( wParam == VK_ESCAPE )
DestroyWindow(hwnd);
break;
}
return DefWindowProc(hwnd, msg, wParam, lParam);
}
int WINAPI WinMain(HINSTANCE hinstance, HINSTANCE prevInstance, PSTR cmdLine, int showCmd)
{
if(!InitD3D(hinstance, WIDTH, HEIGHT, true, D3DDEVTYPE_HAL, &g_d3d_device))
{
MessageBox(0, "InitD3D() - FAILED", 0, 0);
return 0;
}
if(!Setup())
{
MessageBox(0, "Setup() - FAILED", 0, 0);
return 0;
}
EnterMsgLoop( Display );
Cleanup();
g_d3d_device->Release();
return 0;
}
下载源程序