在D3DX库中提供了一个ID3DXFont接口,它能被用于在Direct3D应用程序中绘制文字。
9.1.1创建一个ID3DXFont
我们能够使用D3DXCreateFontIndirect函数来创建一个ID3DXFont接口。
Creates a font object indirectly for both a device and a font.
HRESULT D3DXCreateFontIndirect(
LPDIRECT3DDEVICE9 pDevice,
CONST D3DXFONT_DESC * pDesc,
LPD3DXFONT * ppFont
);
Parameters
- pDevice
- [in] Pointer to an IDirect3DDevice9 interface, the device to be associated with the font object.
- pDesc
- [in] Pointer to a D3DXFONT_DESC structure, describing the attributes of the font object to create. If the compiler settings require Unicode, the data type D3DXFONT_DESC resolves to D3DXFONT_DESCW; otherwise, the data type resolves to D3DXFONT_DESCA. See Remarks.
- ppFont
- [out] Returns a pointer to an ID3DXFont interface, representing the created font object.
Return Values
If the function succeeds, the return value is D3D_OK. If the function fails, the return value can be one of the following: D3DERR_INVALIDCALL, E_OUTOFMEMORY.
Remarks
The compiler setting also determines the function version. If Unicode is defined, the function call resolves to D3DXCreateFontIndirectW. Otherwise, the function call resolves to D3DXCreateFontIndirectA because ANSI strings are being used.
Defines the attributes of a font.
typedef struct D3DXFONT_DESC {
INT Height;
UINT Width;
UINT Weight;
UINT MipLevels;
BOOL Italic;
BYTE CharSet;
BYTE OutputPrecision;
BYTE Quality;
BYTE PitchAndFamily;
TCHAR FaceName[LF_FACESIZE];
} D3DXFONT_DESC, *LPD3DXFONT_DESC;
Members
- Height
- Height, in logical units, of the font's character cell or character.
- Width
- Width, in logical units, of characters in the font.
- Weight
- Weight of the font in the range from 0 through 1000.
- MipLevels
- Number of mip levels requested. If this value is zero or D3DX_DEFAULT, a complete mipmap chain is created. If the value is 1, the texture space is mapped identically to the screen space.
- Italic
- Set to TRUE for an Italic font.
- CharSet
- Character set.
- OutputPrecision
- Output precision. The output precision defines how closely the output must match the requested font height, width, character orientation, escapement, pitch, and font type.
- Quality
- Output quality.
- PitchAndFamily
- Pitch and family of the font.
- FaceName
- A null-terminated string or characters that specifies the typeface name of the font. The length of the string must not exceed 32 characters, including the terminating null character. If FaceName is an empty string, the first font that matches the other specified attributes will be used. If the compiler settings require Unicode, the data type TCHAR resolves to WCHAR; otherwise, the data type resolves to CHAR. See Remarks.
Remarks
The compiler setting also determines the structure type. If Unicode is defined, the D3DXFONT_DESC structure type resolves to a D3DXFONT_DESCW; otherwise the structure type resolves to a D3DXFONT_DESCA.
Possible values of the above members are given in the GDI LOGFONT structure.
下面的代码片段显示了怎样使用这个函数:
D3DXFONT_DESC font_desc;
ZeroMemory(&font_desc, sizeof(font_desc));
font_desc.Height = 25; // in logical units
font_desc.Width = 12; // in logical units
font_desc.Weight = 500; // boldness, range 0(light) - 1000(bold)
font_desc.Italic = FALSE;
font_desc.CharSet = DEFAULT_CHARSET;
font_desc.OutputPrecision = 0;
font_desc.Quality = 0;
font_desc.PitchAndFamily = 0;
strcpy(font_desc.FaceName, "Times New Roman");
if(FAILED(D3DXCreateFontIndirect(g_d3d_device, &font_desc, &g_d3d_font)))
{
MessageBox(NULL, "D3DXCreateFontIndirect() - FAILED", NULL, MB_OK);
PostQuitMessage(0);
}
注意:你也能够使用D3DXCreateFont函数来获得一个ID3DXFont接口指针。
9.1.2绘制文本
一旦我们获得了ID3DXFont接口指针,绘制文本就是很简单的事情了,我们只要调用ID3DXFont::DrawText方法就可以实现了。
Draws formatted text. This method supports ANSI and Unicode strings.
INT DrawText(
LPD3DXSPRITE pSprite,
LPCTSTR pString,
INT Count,
LPRECT pRect,
DWORD Format,
D3DCOLOR Color
);
Parameters
- pSprite
- [in] Pointer to an ID3DXSprite object that contains the string. Can be NULL, in which case Direct3D will render the string with its own sprite object. To improve efficiency, a sprite object should be specified if ID3DXFont::DrawText is to be called more than once in a row.
- pString
- [in] Pointer to a string to draw.If the Count parameter is -1, the string must be null-terminated.
- Count
- [in] Specifies the number of characters in the string. If Count is -1, then the pString parameter is assumed to be a pointer to a null-terminated string and ID3DXFont::DrawText computes the character count automatically.
- pRect
- [in] Pointer to a RECT structure that contains the rectangle, in logical coordinates, in which the text is to be formatted. As with any RECT object, the coordinate value of the rectangle's right side must be greater than that of its left side. Likewise, the coordinate value of the bottom must be greater than that of the top.
- Format
- [in] Specifies the method of formatting the text. It can be any combination of the following values:
- DT_BOTTOM
- Justifies the text to the bottom of the rectangle. This value must be combined with DT_SINGLELINE.
- DT_CALCRECT
- Determines the width and height of the rectangle. If there are multiple lines of text, ID3DXFont::DrawText uses the width of the rectangle pointed to by the pRect parameter and extends the base of the rectangle to bound the last line of text. If there is only one line of text, ID3DXFont::DrawText modifies the right side of the rectangle so that it bounds the last character in the line. In either case, ID3DXFont::DrawText returns the height of the formatted text but does not draw the text.
- DT_CENTER
- Centers text horizontally in the rectangle.
- DT_EXPANDTABS
- Expands tab characters. The default number of characters per tab is eight.
- DT_LEFT
- Aligns text to the left.
- DT_NOCLIP
- Draws without clipping. ID3DXFont::DrawText is somewhat faster when DT_NOCLIP is used.
- DT_RIGHT
- Aligns text to the right.
- DT_RTLREADING
- Displays text in right-to-left reading order for bidirectional text when a Hebrew or Arabic font is selected. The default reading order for all text is left-to-right.
- DT_SINGLELINE
- Displays text on a single line only. Carriage returns and line feeds do not break the line.
- DT_TOP
- Top-justifies text.
- DT_VCENTER
- Centers text vertically (single line only).
- DT_WORDBREAK
- Breaks words. Lines are automatically broken between words if a word would extend past the edge of the rectangle specified by the pRect parameter. A carriage return/line feed sequence also breaks the line.
- Color
- [in] Color of the text. For more information, see D3DCOLOR.
Return Values
If the function succeeds, the return value is the height of the text in logical units. If DT_VCENTER or DT_BOTTOM is specified, the return value is the offset from pRect (top to the bottom) of the drawn text. If the function fails, the return value is zero.
Remarks
The parameters of this method are very similar to those of the GDI DrawText function.
This method supports both ANSI and Unicode strings.
This method must be called inside a IDirect3DDevice9::BeginScene ... IDirect3DDevice9::EndScene block. The only exception is when an application calls ID3DXFont::DrawText with DT_CALCRECT to calculate the size of a given block of text.
Unless the DT_NOCLIP format is used, this method clips the text so that it does not appear outside the specified rectangle. All formatting is assumed to have multiple lines unless the DT_SINGLELINE format is specified.
If the selected font is too large for the rectangle, this method does not attempt to substitute a smaller font.
This method supports only fonts whose escapement and orientation are both zero.
运行截图:
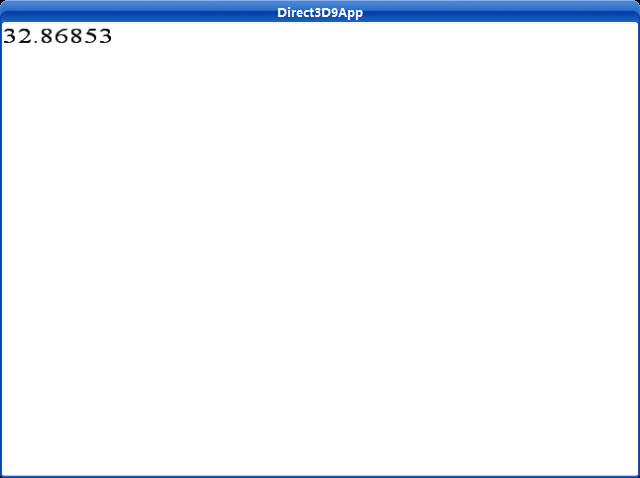
主程序:
/**************************************************************************************
Shows how to calculate the number of frames rendered per second and demonstrates
how to render text with the ID3DXFont interface.
**************************************************************************************/
#include <stdio.h>
#include "d3dUtility.h"
#pragma warning(disable : 4100 4996)
const int WIDTH = 640;
const int HEIGHT = 480;
IDirect3DDevice9* g_d3d_device;
ID3DXFont* g_d3d_font;
DWORD g_frame_count;
float g_time_elapsed;
float g_fps;
char g_fps_string[9];
////////////////////////////////////////////////////////////////////////////////////////////////////
bool setup()
{
D3DXFONT_DESC font_desc;
ZeroMemory(&font_desc, sizeof(font_desc));
font_desc.Height = 25; // in logical units
font_desc.Width = 12; // in logical units
font_desc.Weight = 500; // boldness, range 0(light) - 1000(bold)
font_desc.Italic = FALSE;
font_desc.CharSet = DEFAULT_CHARSET;
font_desc.OutputPrecision = 0;
font_desc.Quality = 0;
font_desc.PitchAndFamily = 0;
strcpy(font_desc.FaceName, "Times New Roman");
if(FAILED(D3DXCreateFontIndirect(g_d3d_device, &font_desc, &g_d3d_font)))
{
MessageBox(NULL, "D3DXCreateFontIndirect() - FAILED", NULL, MB_OK);
PostQuitMessage(0);
}
return true;
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
void cleanup()
{
safe_release<ID3DXFont*>(g_d3d_font);
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
bool display(float time_delta)
{
// update: compute the frames per second.
g_frame_count++;
g_time_elapsed += time_delta;
if(g_time_elapsed >= 1.0f)
{
g_fps = g_frame_count / g_time_elapsed;
sprintf(g_fps_string, "%f", g_fps);
g_fps_string[8] = '\0'; // mark end of string
g_time_elapsed = 0.0f;
g_frame_count = 0;
}
// render
g_d3d_device->Clear(0, NULL, D3DCLEAR_TARGET | D3DCLEAR_ZBUFFER, 0xffffffff, 1.0f, 0);
g_d3d_device->BeginScene();
RECT rect = {0, 0, WIDTH, HEIGHT};
g_d3d_font->DrawText(NULL, g_fps_string, -1, &rect, DT_TOP | DT_LEFT, 0xff000000);
g_d3d_device->EndScene();
g_d3d_device->Present(NULL, NULL, NULL, NULL);
return true;
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
LRESULT CALLBACK wnd_proc(HWND hwnd, UINT msg, WPARAM word_param, LPARAM long_param)
{
switch(msg)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
case WM_KEYDOWN:
if(word_param == VK_ESCAPE)
DestroyWindow(hwnd);
break;
}
return DefWindowProc(hwnd, msg, word_param, long_param);
}
///////////////////////////////////////////////////////////////////////////////////////////////////////
int WINAPI WinMain(HINSTANCE inst, HINSTANCE, PSTR cmd_line, int cmd_show)
{
if(! init_d3d(inst, WIDTH, HEIGHT, true, D3DDEVTYPE_HAL, &g_d3d_device))
{
MessageBox(NULL, "init_d3d() - failed.", 0, MB_OK);
return 0;
}
if(! setup())
{
MessageBox(NULL, "Steup() - failed.", 0, MB_OK);
return 0;
}
enter_msg_loop(display);
cleanup();
g_d3d_device->Release();
return 0;
}
下载源程序