这个程序使用D3DXCreateTeapot函数创建并用DrawSubset函数渲染一个纺纱茶壶。
Uses a left-handed coordinate system to create a mesh
containing a teapot.
HRESULT D3DXCreateTeapot(
LPDIRECT3DDEVICE9 pDevice,
LPD3DXMESH * ppMesh,
LPD3DXBUFFER * ppAdjacency
);
Parameters
- pDevice
- [in] Pointer to an IDirect3DDevice9 interface,
representing the device associated with the created teapot mesh.
- ppMesh
- [out] Address of a pointer to the output shape, an
ID3DXMesh interface.
- ppAdjacency
- [out] Address of a pointer to an ID3DXBuffer
interface. When the method returns, this parameter is filled with an array
of three DWORDs per face that specify the three neighbors for each face in
the mesh. NULL can be specified.
Return Values
If the function succeeds, the return value is D3D_OK.
If the function fails, the return value can be one of the following:
D3DERR_INVALIDCALL, D3DXERR_INVALIDDATA, E_OUTOFMEMORY.
Remarks
This function creates a mesh with the D3DXMESH_MANAGED
creation option and D3DFVF_XYZ | D3DFVF_NORMAL flexible vertex format (FVF).
Draws a subset of a mesh.
HRESULT DrawSubset(
DWORD AttribId
);
Parameters
- AttribId
- [in] DWORD that specifies which subset of the mesh
to draw. This value is used to differentiate faces in a mesh as belonging to
one or more attribute groups.
Return Values
If the method succeeds, the return value is D3D_OK. If
the method fails, the return value can be D3DERR_INVALIDCALL.
Remarks
The subset that is specified by AttribId will be
rendered by the IDirect3DDevice9::DrawIndexedPrimitive method, using the
D3DPT_TRIANGLELIST primitive type, so an index buffer must be properly
initialized.
An attribute table is used to identify areas of the
mesh that need to be drawn with different textures, render states, materials,
and so on. In addition, the application can use the attribute table to hide
portions of a mesh by not drawing a given attribute identifier (AttribId)
when drawing the frame.
截图:
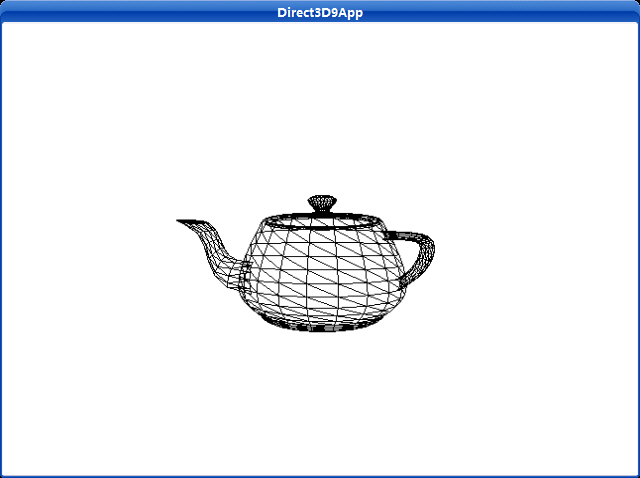
源程序:
/**************************************************************************************
Renders a teapot in wireframe mode. Shows how to create a teapot using the
D3DXCreateTeapot function and how to render the teapot using the ID3DXMesh::DrawSubset
method.
**************************************************************************************/
#include "d3dUtility.h"
#pragma warning(disable : 4100)
const int WIDTH = 640;
const int HEIGHT = 480;
IDirect3DDevice9* g_d3d_device = NULL;
// mesh interface that will store the teapot data and contains method to render the teapot data
ID3DXMesh* g_teapot_mesh = NULL;
////////////////////////////////////////////////////////////////////////////////////////////////////
bool setup()
{
// create the teapot geometry
D3DXCreateTeapot(g_d3d_device, &g_teapot_mesh, NULL);
// position and aim the camera
D3DXVECTOR3 position(0.0f, 0.0f, -3.0f);
D3DXVECTOR3 target(0.0f, 0.0f, 0.0f);
D3DXVECTOR3 up(0.0f, 1.0f, 0.0f);
D3DXMATRIX view_matrix;
D3DXMatrixLookAtLH(&view_matrix, &position, &target, &up);
g_d3d_device->SetTransform(D3DTS_VIEW, &view_matrix);
// set the projection matrix
D3DXMATRIX proj;
D3DXMatrixPerspectiveFovLH(&proj, D3DX_PI * 0.5f, (float)WIDTH/HEIGHT, 1.0f, 1000.0f);
g_d3d_device->SetTransform(D3DTS_PROJECTION, &proj);
// set wireframe mode render state
g_d3d_device->SetRenderState(D3DRS_FILLMODE, D3DFILL_WIREFRAME);
return true;
}
void cleanup()
{
safe_release<ID3DXMesh*>(g_teapot_mesh);
}
bool display(float time_delta)
{
// spin the teapot
D3DXMATRIX ry;
static float y = 0.0f;
D3DXMatrixRotationY(&ry, y);
// increment y-rotation angle each frame
y += time_delta;
// reset angle to zero when angle reaches 2*PI
if(y >= 6.28f)
y = 0.0f;
g_d3d_device->SetTransform(D3DTS_WORLD, &ry);
// draw the scene
g_d3d_device->Clear(0, NULL, D3DCLEAR_TARGET | D3DCLEAR_ZBUFFER, 0xffffffff, 1.0f, 0);
g_d3d_device->BeginScene();
// draw teapot using DrawSubset method with 0 as the argument
g_teapot_mesh->DrawSubset(0);
g_d3d_device->EndScene();
g_d3d_device->Present(NULL, NULL, NULL, NULL);
return true;
}
LRESULT CALLBACK wnd_proc(HWND hwnd, UINT msg, WPARAM word_param, LPARAM long_param)
{
switch(msg)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
case WM_KEYDOWN:
if(word_param == VK_ESCAPE)
DestroyWindow(hwnd);
break;
}
return DefWindowProc(hwnd, msg, word_param, long_param);
}
int WINAPI WinMain(HINSTANCE inst, HINSTANCE, PSTR cmd_line, int cmd_show)
{
if(! init_d3d(inst, WIDTH, HEIGHT, true, D3DDEVTYPE_HAL, &g_d3d_device))
{
MessageBox(NULL, "init_d3d() - failed.", 0, MB_OK);
return 0;
}
if(! setup())
{
MessageBox(NULL, "Steup() - failed.", 0, MB_OK);
return 0;
}
enter_msg_loop(display);
cleanup();
g_d3d_device->Release();
return 0;
}
下载茶壶源程序