本篇是创建游戏内核(8)【接口与实现分离版】的续篇,关于该内核的细节说明请参考创建游戏内核(9),这个版本主要是按照功能划分模块的思想,并严格按照接口与实现相分离的原则来写的,没有用面向对象的思想来写,没有继承没有多态。大家可以对比两个版本,比较优劣。
接口:
BOOL load_texture(LPDIRECT3DTEXTURE9* texture, char* filename, DWORD transparent, D3DFORMAT format);
BOOL create_texture(LPDIRECT3DTEXTURE9* texture, DWORD width, DWORD height, D3DFORMAT format);
BOOL clone_texture(LPDIRECT3DTEXTURE9* dest_texture, const LPDIRECT3DTEXTURE9 src_texture);
long get_texture_width(const LPDIRECT3DTEXTURE9 texture);
long get_texture_height(const LPDIRECT3DTEXTURE9 texture);
long get_texture_format(const LPDIRECT3DTEXTURE9 texture);
BOOL draw_texture(const LPDIRECT3DTEXTURE9 texture,
long dest_x, long dest_y,
long src_x, long src_y,
long width, long height,
float x_scale, float y_scale,
D3DCOLOR color);
实现:
//-------------------------------------------------------------------
// create texture object from specified file, you can specify transparent
// value and pixel format of the texture.
//-------------------------------------------------------------------
BOOL load_texture(LPDIRECT3DTEXTURE9* texture, char* filename, DWORD transparent, D3DFORMAT format)
{
// check condition first
if(g_d3d_device == NULL || filename == NULL)
return FALSE;
// create a texture from file
if(FAILED(D3DXCreateTextureFromFileEx(g_d3d_device, filename, D3DX_DEFAULT, D3DX_DEFAULT,
D3DX_DEFAULT, 0, format, D3DPOOL_MANAGED, D3DX_FILTER_TRIANGLE, D3DX_FILTER_TRIANGLE,
transparent, NULL, NULL, texture)))
{
return FALSE;
}
return TRUE;
}
//-------------------------------------------------------------------
// Creates a texture resource.
//-------------------------------------------------------------------
BOOL create_texture(LPDIRECT3DTEXTURE9* texture, DWORD width, DWORD height, D3DFORMAT format)
{
if(FAILED(g_d3d_device->CreateTexture(width, height, 0, 0, format, D3DPOOL_MANAGED, texture, NULL)))
return FALSE;
return TRUE;
}
//-------------------------------------------------------------------
// Configure a TEXTURE class from an existing IDirect3DTexture9 object
// instance.
//-------------------------------------------------------------------
BOOL clone_texture(LPDIRECT3DTEXTURE9* dest_texture, const LPDIRECT3DTEXTURE9 src_texture)
{
D3DLOCKED_RECT _src_rect, _dest_rect;
D3DSURFACE_DESC _surface_desc;
if(src_texture == NULL)
return FALSE;
// copy texture voer, from source to dest.
if(FAILED(src_texture->GetLevelDesc(0, &_surface_desc)))
return FALSE;
g_d3d_device->CreateTexture(_surface_desc.Width, _surface_desc.Height, 0, 0,
_surface_desc.Format, D3DPOOL_MANAGED, dest_texture, NULL);
// locks a rectangle on a texture resource
src_texture->LockRect(0, &_src_rect, NULL, D3DLOCK_READONLY);
(*dest_texture)->LockRect(0, &_dest_rect, NULL, 0);
memcpy(_dest_rect.pBits, _src_rect.pBits, _src_rect.Pitch * _surface_desc.Height);
// unlocks a rectangle on a texture resource
src_texture->UnlockRect(0);
(*dest_texture)->UnlockRect(0);
return TRUE;
}
//-------------------------------------------------------------------
// Get width of the texture.
//-------------------------------------------------------------------
long get_texture_width(const LPDIRECT3DTEXTURE9 texture)
{
D3DSURFACE_DESC _surface_desc;
if(texture == NULL)
return 0;
if(FAILED(texture->GetLevelDesc(0, &_surface_desc)))
return 0;
return _surface_desc.Width;
}
//-------------------------------------------------------------------
// Get height of the texture.
//-------------------------------------------------------------------
long get_texture_height(const LPDIRECT3DTEXTURE9 texture)
{
D3DSURFACE_DESC _surface_desc;
if(texture == NULL)
return 0;
if(FAILED(texture->GetLevelDesc(0, &_surface_desc)))
return 0;
return _surface_desc.Height;
}
//-------------------------------------------------------------------
// Get texture storage format.
//-------------------------------------------------------------------
long get_texture_format(const LPDIRECT3DTEXTURE9 texture)
{
D3DSURFACE_DESC _surface_desc;
if(texture == NULL)
return D3DFMT_UNKNOWN;
if(FAILED(texture->GetLevelDesc(0, &_surface_desc)))
return D3DFMT_UNKNOWN;
return _surface_desc.Format;
}
//-------------------------------------------------------------------
// Draw a 2D portion of texture to device.
//-------------------------------------------------------------------
BOOL draw_texture(const LPDIRECT3DTEXTURE9 texture,
long dest_x, long dest_y,
long src_x, long src_y,
long width, long height,
float x_scale, float y_scale,
D3DCOLOR color)
{
// check condition
if(texture == NULL)
return FALSE;
LPD3DXSPRITE _sprite;
if(FAILED(D3DXCreateSprite(g_d3d_device, &_sprite)))
return FALSE;
// If no specify width and height, set with texture width and height.
if(width == 0)
width = get_texture_width(texture);
if(height == 0)
height = get_texture_height(texture);
// set the portion of the source texture
RECT _rect;
_rect.left = src_x;
_rect.top = src_y;
_rect.right = src_x + width;
_rect.bottom = src_y + height;
D3DXMATRIX _transform_matrix(x_scale, 0, 0, 0,
0, y_scale, 0, 0,
0, 0, 0, 0,
(float)dest_x, (float)dest_y, 0, 0);
// sets the sprite transforma
_sprite->SetTransform(&_transform_matrix);
// adds a sprite to the list of batched sprites
if(FAILED(_sprite->Draw(texture, &_rect, NULL, NULL, color)))
return FALSE;
return TRUE;
}
测试代码:
/***********************************************************************************
PURPOSE:
Test D3D texture function.
***********************************************************************************/
#include <windows.h>
#include "core_framework.h"
#include "core_graphics.h"
#include "core_tool.h"
// The 3D vertex format and descriptor
typedef struct
{
float x, y, z; // 3D coordinates
float u, v; // texture coordinates
} VERTEX;
#define VERTEX_FVF (D3DFVF_XYZ | D3DFVF_TEX1)
IDirect3DVertexBuffer9* g_vertex_buffer;
IDirect3DTexture9* g_texture;
//--------------------------------------------------------------------------------
// Initialize data for game.
//--------------------------------------------------------------------------------
BOOL game_init()
{
// initialize vertex data
VERTEX _verts[] = {
{ -150.0f, 150.0f, 0.0f, 0.0f, 0.0f },
{ 150.0f, 150.0f, 0.0f, 1.0f, 0.0f },
{ -150.0f, -150.0f, 0.0f, 0.0f, 1.0f },
{ 150.0f, -150.0f, 0.0f, 1.0f, 1.0f }
};
// Create Direct3D and Direct3DDevice object
if(! create_display(g_hwnd, get_window_width(g_hwnd), get_window_height(g_hwnd), 16, TRUE, FALSE))
return FALSE;
// set perspective projection transform matrix
set_perspective(D3DX_PI/4.0f, 1.33333f, 1.0f, 1000.0f);
D3DXMATRIX _mat_view;
// create and set the view matrix
D3DXMatrixLookAtLH(&_mat_view,
&D3DXVECTOR3(0.0, 0.0, -500.0),
&D3DXVECTOR3(0.0f, 0.0f, 0.0f),
&D3DXVECTOR3(0.0f, 1.0f, 0.0f));
g_d3d_device->SetTransform(D3DTS_VIEW, &_mat_view);
BYTE* _vertex_ptr;
// create the vertex buffer and set data
g_d3d_device->CreateVertexBuffer(sizeof(_verts), 0, VERTEX_FVF, D3DPOOL_DEFAULT, &g_vertex_buffer, NULL);
// locks a range of vertex data and obtains a pointer to the vertex buffer memory
g_vertex_buffer->Lock(0, 0, (void**)&_vertex_ptr, 0);
memcpy(_vertex_ptr, _verts, sizeof(_verts));
// unlocks vertex data
g_vertex_buffer->Unlock();
// load the texture map
if(! load_texture(&g_texture, "texture.jpg", 0, D3DFMT_UNKNOWN))
return FALSE;
return TRUE;
}
//--------------------------------------------------------------------------------
// Render every game frame.
//--------------------------------------------------------------------------------
BOOL game_frame()
{
clear_display_buffer(D3DCOLOR_RGBA(0, 0, 0, 255));
if(SUCCEEDED(g_d3d_device->BeginScene()))
{
// set world tranformation
D3DXMATRIX _mat_world;
D3DXMatrixRotationZ(&_mat_world, (float) (timeGetTime() / 1000.0));
g_d3d_device->SetTransform(D3DTS_WORLD, &_mat_world);
// set the vertex stream, shader, and texture.
// binds a vertex buffer to a device data stream
g_d3d_device->SetStreamSource(0, g_vertex_buffer, 0, sizeof(VERTEX));
// set the current vertex stream declation
g_d3d_device->SetFVF(VERTEX_FVF);
// assigns a texture to a stage for a device
set_texture(0, g_texture);
// renders a sequence of noindexed, geometric primitives of the specified type from the current set
// of data input stream.
g_d3d_device->DrawPrimitive(D3DPT_TRIANGLESTRIP, 0, 2);
// release texture
set_texture(0, NULL);
g_d3d_device->EndScene();
}
present_display();
return TRUE;
}
//--------------------------------------------------------------------------------
// Release all game resources.
//--------------------------------------------------------------------------------
BOOL game_shutdown()
{
release_com(g_vertex_buffer);
release_com(g_texture);
release_com(g_d3d_device);
release_com(g_d3d);
return TRUE;
}
//--------------------------------------------------------------------------------
// Main function, routine entry.
//--------------------------------------------------------------------------------
int WINAPI WinMain(HINSTANCE inst, HINSTANCE pre_inst, LPSTR cmd_line, int cmd_show)
{
if(! build_window(inst, "MainClass", "MainWindow", WS_OVERLAPPEDWINDOW, 0, 0, 640, 480))
return FALSE;
run_game(game_init, game_frame, game_shutdown);
return 0;
}
点击下载源码和工程
程序截图:
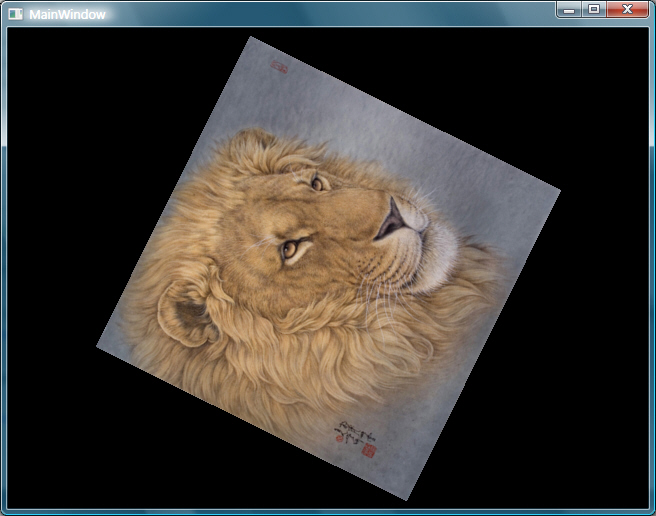