来源:http://www.flounder.com/wm_copydata.htm
I have experienced several problems, not of my own creation, in using WM_COPYDATA. Now, when I need to use it, I use a mechanism that protects me against the sort of problems I experienced. This essay describes those techniques for creating programs that can reliably and with some assurances of security use WM_COPYDATA.
Introduction to WM_COPYDATA
The WM_COPYDATA message is used to send large blocks of data across process boundaries. It is defined as
SendMessage(target, WM_COPYDATA, (WPARAM)sender, (LPARAM)©DATASTRUCT)
The WPARAM is the handle of the sending window. The LPARAM is a pointer to a structure of the form

typedef struct tagCOPYDATASTRUCT
{
ULONG_PTR dwData;
DWORD cbData;
PVOID lpData;
} COPYDATASTRUCT, *PCOPYDATASTRUCT;
Presumably the dwData member is a small integer that tells the receiving window how to interpret the data referenced by lpData. The cbData value tells how many bytes of data are being passed.
This message can only be sent via SendMessage, which means that it will hang indefinitely if the receiving thread is blocked, or via SendMessageTimeout, if you want reliability. However, it cannot be send via PostMessage.
The data specified appears in the receiver's address space, and is pointed to by a similar COPYDATASTRUCT seen by the receiver. The receiver can examine the dwData to determine what to do, and use at most cbData bytes of the data pointed to by the lpData pointer. The data is intact only as long as the message is being processed. When you return from the OnCopyData handler, the data will disappear from the receiver's address space, so you must not store a copy of the lpData pointer. Instead, if you need the data after the handler returns, you must, in the receiving process, copy the data to a place in the program where it will remain available.
WM_COPYDATA hazards
I don't trust WM_COPYDATA.
It is not that I don't trust it to work. Indeed, it is reliable. It is not that I don't trust it to actually deliver data. It does that, too.
What I don't trust, as the receiver, is the sender. Having the WPARAM value which tells me the sender window handle doesn't help much, because the sender window could be any window of an application, not just the main frame. I have actually been the victim of an incorrect WM_COPYDATA message. The programmer who was sending it had no idea how to find the desired target window, so instead, "cleverly" decided to broadcast the WM_COPYDATA message to all top-level windows, knowing that his window would eventually get it. Of course, mine did also.
Furthermore, sending a bogus WM_COPYDATA is something a malicious program might choose to do in order to cause other programs to crash. I want to be robust against this sort of attack.
Making WM_COPYDATA safe
What I do for security is create a GUID. In this case, I don't want the textual form, I want the binary form. I then place this GUID, which is a 128-bit value, in the first part of my data. When I code the receiver, I test the first 16 bytes of every WM_COPYDATA packet (and there must be at least 16 bytes in the WM_COPYDATA packet, or I ignore it). If the first 16 bytes of the message do not match the GUID, I simply return 0 and ignore the message. Since I started doing this, I have never been the victim of an erroneous WM_COPYDATA send to my application (even in the environment of rogue WM_COPYDATA messages).
Here's an example of the kind of message I send. In this case, I sent it to my own process, but it would work identically across process boundaries. Note the first 16 bytes are the GUID, which will be shown later. I have three other buttons, one to send a message that is too short, one to send a message with a bad GUID, and one to send a message with an unknown dwType.
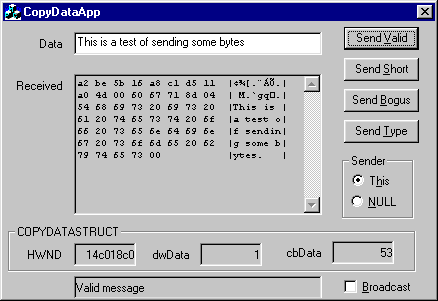
This shows what happens if I send fewer than sizeof(GUID) bytes:
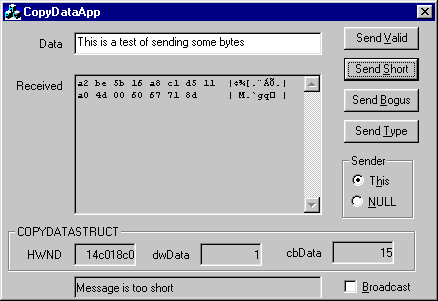
If I send a bad GUID (look at the 5th byte in the first line and compare it to the first version, above), I get
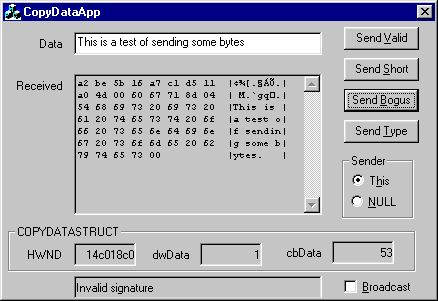
and if I send a bad type, I get the following result. Note that the dwData field is 2, and I was only prepared to accept 1.
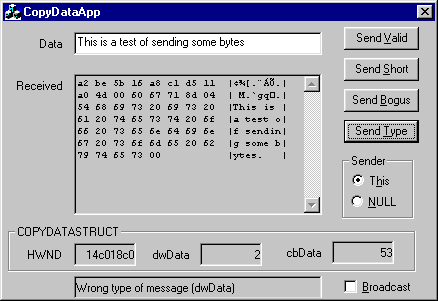
Here's my set of related classes, a file I call CopyData.h.
#ifndef MAX_COPY_LENGTH
#define MAX_COPY_LENGTH 128
#endif


class CopyPacket
{
public:
GUID Signature;
BYTE data[MAX_COPY_LENGTH];
public:
BOOL Same(const GUID & s)

{ return memcmp(&Signature, &s, sizeof(GUID)) == 0; }
};


class CopyData
{
public:
CopyData(UINT id, GUID s)

{ packet.Signature = s; cds.dwData = id; cds.cbData = 0; cds.lpData = &packet; }
UINT SetLength(UINT n)

{ cds.cbData = sizeof(packet.Signature) + n; return cds.cbData; }
void SetData(LPCVOID src, size_t length)

{ ::CopyMemory(packet.data, src, length); }
LRESULT Send(HWND target, HWND sender)

{ return ::SendMessage(target, WM_COPYDATA, (WPARAM)sender, (LPARAM)&cds); }
static UINT GetMinimumLength()

{ return sizeof(GUID); }
protected:
COPYDATASTRUCT cds;
CopyPacket packet;
};
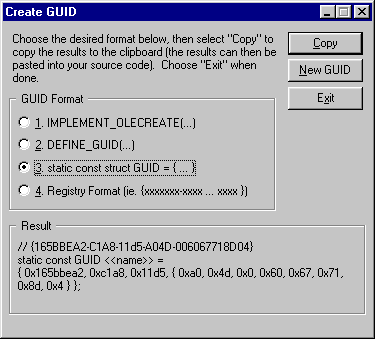
To create the signature, I use GUIDGen, and create a GUID using the option shown: If you are not aware of it, a GUID is
globally unique; that is, once you create one, it is the only one which will ever exist, anywhere in the world. No one else will ever re-create the same GUID.
OK, a fine point. If you don't have a network card, there is something like 1 chance in 263 or something equally large that two 128-bit GUIDs could coincide. Figure out how many computers need to create how many GUIDs at what rate and you'll see this is not a Major Issue To Be Concerned With.
The option I show is the closest one to what I want. I click on the Copy button, then I paste the text into a file called signature.h. I replace the first line with
#define SIGNATURE \
I then declare, in my implementation files, a static global variable which defines the signature. Each module that wants to send or receive a message must contain this declaration.
static const GUID Signature = SIGNATURE;

I need a dwType to distinguish what kind of message I'm sending. In this case, I am only sending one type of message, which I have decided to call COPYDATA_TYPE_1, which I define as the constant 1 (a whimsical choice).
To send a message, such as the valid sending, I do something like shown below (this is simplified, because I use the various check boxes and options to derive the window handles). Also, in my case, I'm sending the message from my application to itself, so the first parameter (target) and the second parameter (sender) are both m_hWnd.
void CCopyDataDlg::OnSendvalid()

{
CopyData sendData(COPYDATA_TYPE_1, Signature);
CString s;
c_Input.GetWindowText(s);

sendData.SetData((LPCVOID)(LPCTSTR)s, s.GetLength()+1);
sendData.SetLength(s.GetLength() + 1);
sendData.Send(m_hWnd, m_hWnd);
}
The receive code, minus the code that loads the messages into the status boxes, is then quite simple:

BOOL CCopyDataDlg::OnCopyData(CWnd* pWnd, COPYDATASTRUCT* cds)

{
CopyPacket * cp = (CopyPacket *)cds->lpData;
BOOL result = TRUE;

if(cds->dwData != COPYDATA_TYPE_1)

{ /**//* bad type */
result = FALSE;

} /**//* bad type */

if(cds->cbData < CopyData::GetMinimumLength())

{ /**//* record too short */
result = FALSE;

} /**//* record too short */

if(!cp->Same(Signature))

{ /**//* record bad signature */
result = FALSE;

} /**//* record bad signature */

if(result)

{ /**//* success */
do something with the message

} /**//* success */

return result;
}

Broadcast Usage
Note that using HWND_BROADCAST as the target window will send the message to every window. In this example, I have two copies of my program running, and the upper-left window receives the same data as the lower right window. However, every other application window in my desktop received this message. I was lucky. None of them were bothered by the WM_COPYDATA. This is not always going to be the case. So sending a message like this using HWND_BROADCAST is to be considered a seriously antisocial act. If you download my files and try to send using HWND_BROADCAST (checking the Broadcast box), you may well crash programs you have running. If so, Let That Be A Lesson To You.
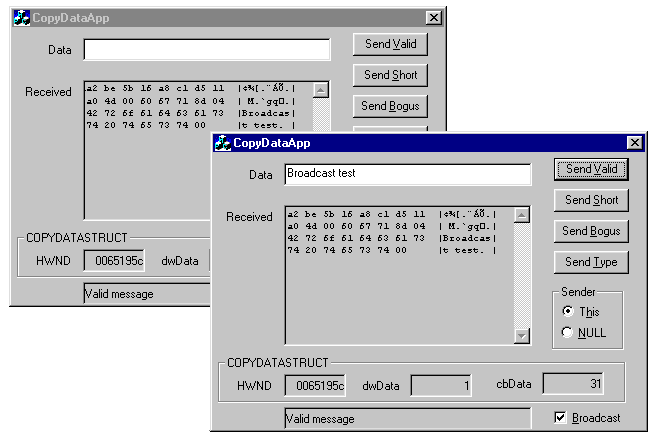
Passing Pointers
Note that everything inside the WM_COPYDATA block must be part of the block. You can't use it to pass a pointer to something. For example, it would be a fatal error to pass a CString variable in the WM_COPYDATA block because the string itself would still be in the address space of the sender. If you need to pass a complex structure, you have to make all of the pointers in the WM_COPYDATA block be based pointers that reference the contents of the data block. That is an essay I may write someday.
Summary
WM_COPYDATA is a powerful and convenient means for transferring information between two processes. It is not without risks. These techniques were developed to minimize those risks.
You can download the code for my test app and my CopyData.h file. However, you should not reuse my GUID because there is always the chance someone else will have also copied it, and you lose the security that having a unique ID provides.