The Gof has 23 design patterns , but in most of the design books , the E.G is written in Java , So from now on , I will write about 23 articles to implement them using C++ , the design pattern itself is discussible , so welcome everyone to give his excelent idea to me , My QQ: 286259397 And My MSN :
cxl82116@msn.com.
Welcome every one to express his ideas!
This is the Second Arcticle: ADAPTER PATTERN
While referring to Adapter pattern , there must be a class that play the role of a adapter which can make two or more umcompatible class compatible . To explain this world , the UML diagram can make it clear easily :
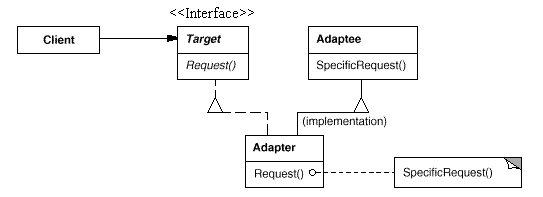
diagram 2-1 Class Adapter UML (referred from http://terrylee.cnblogs.com)
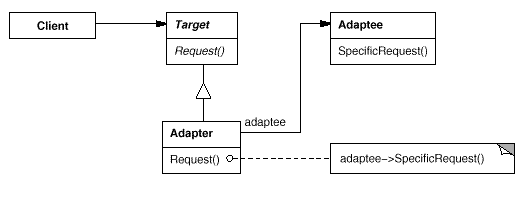
diagram 2-2 Object Adapter UML (referred from http://terrylee.cnblogs.com)
E.G Explain them sparetely:
if a Customer request a CUP WITH A CAP , but the orgin system can only supply a CUP , So you it's not compatible between the Request and the Orgin System , so you must find anthor factory who supply the cap ,and make it together to statisfy the customer , implement it in Class Adapter Pattern , Codes goes below.
Class Adapter Pattern:
1
/**//********************************************************************
2
created: 2007/04/21
3
created: 21:4:2007 18:47
4
filename: c:\Visual Studio 2005\Projects\Frist2005\Frist2005\Frist2005.cpp
5
file path: c:\Visual Studio 2005\Projects\Frist2005\Frist2005
6
file base: Frist2005
7
file ext: cpp
8
author: Chang Xinglong(King.C)
9
10
purpose: Adapter Pattern -Class
11
*********************************************************************/
12
13
#include "stdafx.h"
14
#include <map>
15
#include <iostream>
16
17
using namespace std;
18
19
class OrginSystem
20

{
21
22
public:
23
void Supply()
24
{
25
cout<<"Orgin:SupplyACup"<<endl;
26
}
27
};
28
class CapSystem
29

{
30
public:
31
void Supply()
32
{
33
cout<<"CapSystem:SupplyACap"<<endl;
34
}
35
};
36
class Adapter: public OrginSystem , CapSystem
37

{
38
public:
39
void Supply()
40
{
41
OrginSystem::Supply();
42
CapSystem::Supply();
43
cout<<"So the request has been satisfied!"<<endl;
44
}
45
};
46
int _tmain(int argc, _TCHAR* argv[])
47

{
48
Adapter _adpter;
49
_adpter.Supply();
50
system("pause");
51
return 0;
52
}
The Same question , implement it using Object Adapter Pattern:
1
/**//********************************************************************
2
created: 2007/04/21
3
created: 21:4:2007 18:47
4
filename: c:\Visual Studio 2005\Projects\Frist2005\Frist2005\Frist2005.cpp
5
file path: c:\Visual Studio 2005\Projects\Frist2005\Frist2005
6
file base: Frist2005
7
file ext: cpp
8
author: Chang Xinglong(King.C)
9
10
purpose: Adapter Pattern-Object
11
*********************************************************************/
12
13
#include "stdafx.h"
14
#include <map>
15
#include <iostream>
16
17
using namespace std;
18
19
class OrginSystem
20

{
21
22
public:
23
void Supply()
24
{
25
cout<<"Orgin:SupplyACup"<<endl;
26
}
27
};
28
class CapSystem
29

{
30
public:
31
void Supply()
32
{
33
cout<<"CapSystem:SupplyACap"<<endl;
34
}
35
};
36
class Adapter: public OrginSystem , CapSystem
37

{
38
public:
39
Adapter():_os(new OrginSystem),_cs(new CapSystem)
40
{
41
42
}
43
void Supply()
44
{
45
_os->Supply();
46
_cs->Supply();
47
cout<<"So the request has been satisfied!"<<endl;
48
}
49
private:
50
OrginSystem *_os;
51
CapSystem * _cs;
52
};
53
int _tmain(int argc, _TCHAR* argv[])
54

{
55
OrginSystem _orign=new _adpter();
56
_orign.Supply();
57
system("pause");
58
return 0;
59
}
60
61
In C++ , Muiltple-Derived pattern is not a recommend pattern, So if possible , use the Obeject -Adpter Pattern.
That's all ,Thanks! if any different idea , send me E-mail.