由于接下去要用uniscribe(这是一个可以告诉我们在渲染一个超长unicode字符串的时候,什么地方可以换行,什么地方要换顺序,什么字符要用一种神奇的方法来渲染之类的库)做可以插入图片和其它乱七八糟东西的rich text box,为了更方便做实验,而且也考虑到很多软件也需要直接绘图的功能,所以我写了这么两个Demo:
1、Rendering.RawAPI.GDI(http://www.gaclib.net/Demos/Rendering.RawAPI.GDI/Demo.html)
2、Rendering.RawAPI.Direct2D(http://www.gaclib.net/Demos/Rendering.RawAPI.Direct2D/Demo.html)
由于这两个Demo很像,而且Direct2D的比较复杂,所以我在这里介绍一下这个Direct2D的demo。
在Demo里面可以看到,我们可以使用GuiGDIElement或者GuiDirect2DElement来进行手工的绘图操作。这两个Element的使用有限制。当GacUI使用GDI绘图(SetupWindowsGDIRenderer)的时候才可以使用GuiGDIElement,对于Direct2D也是一样的。在使用它们进行绘图的时候,坐标用的是窗口的坐标。但是GacUI会在绘制的时候先加入一个clip,这样我们在绘制的时候就算绘制出了边界,也不会有任何不好的影响。而且这个clip的矩形范围会在渲染事件触发的时候给出。在这里我们先来看一下Direct2D的demo。
首先,整个程序的框架是这样子的:
#include "..\..\Public\Source\GacUI.h"
#include <math.h>
#include <Windows.h>
int CALLBACK WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int CmdShow)
{
// SetupWindowsDirect2DRenderer() is required for GuiDirect2DElement
return SetupWindowsDirect2DRenderer();
}
class Direct2DWindow : public GuiWindow
{
protected:
// arguments.rt is ID2D1RenderTarget.
void element_Rendering(GuiGraphicsComposition* sender, GuiDirect2DElementEventArgs& arguments)
{
}
// The render target is going to be destroyed, any binded resources should be released.
void element_BeforeRenderTargetChanged(GuiGraphicsComposition* sender, GuiDirect2DElementEventArgs& arguments)
{
}
// The new render target is prepared, any binded resources are allowed to recreate now.
void element_AfterRenderTargetChanged(GuiGraphicsComposition* sender, GuiDirect2DElementEventArgs& arguments)
{
}
public:
Direct2DWindow()
:GuiWindow(GetCurrentTheme()->CreateWindowStyle())
{
SetText(L"Rendering.RawAPI.Direct2D");
SetClientSize(Size(640, 480));
GetBoundsComposition()->SetPreferredMinSize(Size(640, 480));
MoveToScreenCenter();
{
GuiDirect2DElement* element=GuiDirect2DElement::Create();
element->Rendering.AttachMethod(this, &Direct2DWindow::element_Rendering);
element->BeforeRenderTargetChanged.AttachMethod(this, &Direct2DWindow::element_BeforeRenderTargetChanged);
element->AfterRenderTargetChanged.AttachMethod(this, &Direct2DWindow::element_AfterRenderTargetChanged);
GuiBoundsComposition* composition=new GuiBoundsComposition;
composition->SetAlignmentToParent(Margin(0, 0, 0, 0));
composition->SetOwnedElement(element);
GetContainerComposition()->AddChild(composition);
}
}
};
void GuiMain()
{
Direct2DWindow window;
GetApplication()->Run(&window);
}
在构造函数里面,我们创建了一个GuiDirect2DElement,然后把它放进一个会自动充满整个窗口的composition里面。然后我们需要监听三个事件(GDI只有一个,就是Rendering):
1、Rendering。这个事件在窗口被绘制的时候调用。GacUI才用了一个低功耗的方法让程序不断的绘制自己,所以我们并不需要担心“如何刷新窗口”的这个问题。
2、BeforeRenderTargetChanged。在这个时候我们要清理掉我们创建出来的资源,譬如说画刷等等。
3、AfterRenderTargetChanged。在这个时候我们要建立一些绘图资源,譬如说画刷等等。
为什么下面两个函数那么蛋疼呢?因为Direct2D的类似画刷这样的东西,是必须跟一个ID2D1RenderTarget绑定在一起的,不同的render target之间的画刷不能共享。而且那个可怜的render target还有可能会失效,这个时候GacUI就要重新创建他们。所以无论如何,都必须监听这三个对象,除非我们只用GuiDirect2DElement来渲染文字(因为文字相关的资源是IDWriteFactory控制的,跟render target无关)。
在这个Demo里面,我们要画的是一个会动的钟。在这个钟里面我们要绘制4种线形:边框、时针、分针、秒针。因此我们需要4个不同的ID2D1SolidColorBrush。由于操作COM对象的时候总要去记得操作那个引用计数,特别的麻烦,而且还容易忘掉。所以我特地为大家提供了一个叫做ComPtr的东西。所以我们就可以这么声明、创建和释放他们:
ComPtr<ID2D1SolidColorBrush> borderBrush;
ComPtr<ID2D1SolidColorBrush> secondBrush;
ComPtr<ID2D1SolidColorBrush> minuteBrush;
ComPtr<ID2D1SolidColorBrush> hourBrush;
// The render target is going to be destroyed, any binded resources should be released.
void element_BeforeRenderTargetChanged(GuiGraphicsComposition* sender, GuiDirect2DElementEventArgs& arguments)
{
borderBrush=0;
secondBrush=0;
minuteBrush=0;
hourBrush=0;
}
// The new render target is prepared, any binded resources are allowed to recreate now.
void element_AfterRenderTargetChanged(GuiGraphicsComposition* sender, GuiDirect2DElementEventArgs& arguments)
{
ID2D1SolidColorBrush* brush;
{
brush=0;
arguments.rt->CreateSolidColorBrush(D2D1::ColorF(0.0f, 0.0f, 0.0f), D2D1::BrushProperties(), &brush);
borderBrush=brush;
}
{
brush=0;
arguments.rt->CreateSolidColorBrush(D2D1::ColorF(0.0f, 0.0f, 1.0f), D2D1::BrushProperties(), &brush);
secondBrush=brush;
}
{
brush=0;
arguments.rt->CreateSolidColorBrush(D2D1::ColorF(0.0f, 1.0f, 0.0f), D2D1::BrushProperties(), &brush);
minuteBrush=brush;
}
{
brush=0;
arguments.rt->CreateSolidColorBrush(D2D1::ColorF(1.0f, 0.0f, 0.0f), D2D1::BrushProperties(), &brush);
hourBrush=brush;
}
}
想必大家都应该看清楚了。Before和After事件里面,GacUI都会提供用来绘图的ID2D1RenderTarget,这个时候必须正确的创建和释放资源。只要这些资源都建立了起来,那么剩下的就只有把一个时钟画出来了。画一个时钟还是很容易的,只需要那么几行代码就行了:
static const int Radius=200;
static const int LongScale=10;
static const int ShortScale=5;
static const int SecondLength=180;
static const int MinuteLength=150;
static const int HourLength=120;
float GetAngle(float second)
{
return (second-15.0f)*3.1416f/30.0f;
}
void DrawLine(ID2D1RenderTarget* rt, ComPtr<ID2D1SolidColorBrush> brush, float angle, int width, int startLength, int endLength, int x, int y)
{
float s=sin(angle);
float c=cos(angle);
float x1=(c*startLength)+(float)(x+Radius);
float y1=(s*startLength)+(float)(y+Radius);
float x2=(c*endLength)+(float)(x+Radius);
float y2=(s*endLength)+(float)(y+Radius);
rt->DrawLine(D2D1::Point2F(x1, y1), D2D1::Point2F(x2, y2), brush.Obj(), (float)width);
}
// arguments.rt is ID2D1RenderTarget.
void element_Rendering(GuiGraphicsComposition* sender, GuiDirect2DElementEventArgs& arguments)
{
int w=arguments.bounds.Width();
int h=arguments.bounds.Height();
int x=arguments.bounds.Left()+(w-Radius*2)/2;
int y=arguments.bounds.Left()+(h-Radius*2)/2;
arguments.rt->DrawEllipse(D2D1::Ellipse(D2D1::Point2F((float)(x+Radius), (float)(y+Radius)), (float)Radius, (float)Radius), borderBrush.Obj());
for(int i=0;i<60;i++)
{
int scale=i%5==0?LongScale:ShortScale;
float angle=GetAngle((float)i);
DrawLine(arguments.rt, borderBrush, angle, 1, Radius-scale, Radius, x, y);
}
DateTime dt=DateTime::LocalTime();
{
float angle=GetAngle(dt.hour*5+dt.minute/12.0f+dt.second/720.0f);
DrawLine(arguments.rt, hourBrush, angle, 5, 0, HourLength, x, y);
}
{
float angle=GetAngle(dt.minute+dt.second/60.0f);
DrawLine(arguments.rt, minuteBrush, angle, 3, 0, MinuteLength, x, y);
}
{
float angle=GetAngle((float)dt.second);
DrawLine(arguments.rt, secondBrush, angle, 1, 0, SecondLength, x, y);
}
}
然后我们就获得了下图:(LiveWrite真是太好了,cppblog的傻逼编辑器每次插入图片都会插入到一个诡异的位置中去)
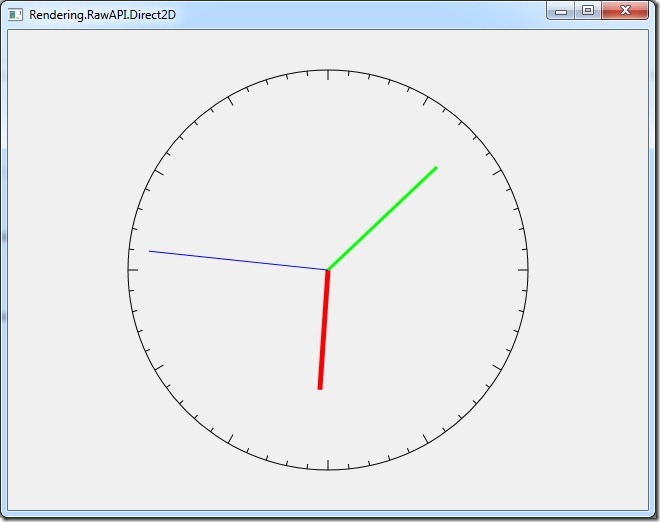
这样我们就完成了一个时钟的制作了,而且也学会了如何在GacUI里面直接使用GDI和Direct2D绘图了。
posted on 2012-11-05 07:14
陈梓瀚(vczh) 阅读(4560)
评论(2) 编辑 收藏 引用 所属分类:
2D 、
GacUI