装饰模式(Decorator)目的是给对象添加职责,其结构图如下:
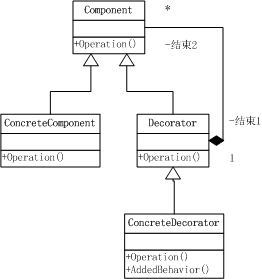
假设现在有一个图书馆,存有大量的书或影碟,相对于书或影碟,其本身没有出借服务,现在就可以借助装饰模式为其添加出借功能,结构图如下:
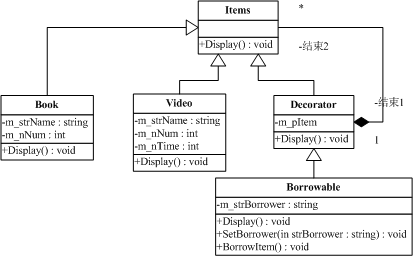
实现代码:
//Items.h
class Items
{
public:
virtual ~Items();
virtual void Display() = 0;
protected:
Items();
};
//Items.cpp
#include "stdafx.h"
#include "Items.h"
Items::Items()
{
}
Items::~Items()
{
}
//Book.h
#include "Items.h"
#include <string>
class Book : public Items
{
public:
Book();
Book(std::string, int);
virtual ~Book();
void Display();
private:
std::string m_strName;
int m_nNum;
};
//Book.cpp
#include "stdafx.h"
#include "Book.h"
#include <iostream>
using namespace std;
Book::Book()
{
m_strName = "";
m_nNum = 0;
}
Book::Book(string strName, int nNum)
{
m_strName = strName;
m_nNum = nNum;
}
Book::~Book()
{
}
void Book::Display()
{
cout << "书名:" << m_strName << " 数量:" << m_nNum << endl;
}
//Video.cpp
#include "Items.h"
#include <string>
class Video : public Items
{
public:
Video();
Video(std::string, int, int);
virtual ~Video();
void Display();
private:
std::string m_strName;
int m_nNum;
int m_nTime;
};
//Video.cpp
#include "stdafx.h"
#include "Video.h"
#include <iostream>
using namespace std;
Video::Video()
{
m_strName = "";
m_nNum = 0;
m_nTime = 0;
}
Video::Video(string strName, int nNum, int nTime)
{
m_strName = strName;
m_nNum = nNum;
m_nTime = nTime;
}
Video::~Video()
{
}
void Video::Display()
{
cout << "电影名:" << m_strName << " 数量:" << m_nNum<< " 播放时间:" << m_nTime << "分钟" << endl;
}
//Decorator.h
#include "Items.h"
class Decorator : public Items
{
public:
Decorator();
Decorator(Items*);
virtual ~Decorator();
virtual void Display();
protected:
Items* m_pItem;
};
//Decorator.cpp
#include "stdafx.h"
#include "Decorator.h"
Decorator::Decorator()
{
m_pItem = NULL;
}
Decorator::Decorator(Items* pItem)
{
m_pItem = pItem;
}
Decorator::~Decorator()
{
if(m_pItem != NULL)
{
delete m_pItem;
m_pItem = NULL;
}
}
void Decorator::Display()
{
m_pItem->Display();
}
//Borrowable.h
#include "Decorator.h"
#include <string>
class Borrowable : public Decorator
{
public:
Borrowable();
Borrowable(Items*);
virtual ~Borrowable();
void SetBorrower(std::string);
void Display();
void BorrowItem();
private:
std::string m_strBorrower;
};
//Borrowable.cpp
#include "stdafx.h"
#include "Borrowable.h"
#include <iostream>
using namespace std;
Borrowable::Borrowable()
{
}
Borrowable::Borrowable(Items* pItem) : Decorator(pItem)
{
}
Borrowable::~Borrowable()
{
}
void Borrowable::SetBorrower(string strBorrower)
{
m_strBorrower = strBorrower;
}
void Borrowable::Display()
{
m_pItem->Display();
BorrowItem();
}
void Borrowable::BorrowItem()
{
cout << "借给:" << m_strBorrower << endl;
}
//main.cpp
#include "stdafx.h"
#include "Items.h"
#include "Book.h"
#include "Video.h"
#include "Decorator.h"
#include "Borrowable.h"
int main(int argc, char* argv[])
{
Items* pItem = new Book("深入浅出设计模式", 10);
pItem->Display();
pItem = new Video("反恐24小时", 24, 200);
Borrowable* pBorrow = new Borrowable(pItem);
pBorrow->SetBorrower("张三");
pBorrow->Display();
return 0;
}
上面,我们显示了一本书的信息,并且将一本影碟借给张三。
最后输出为:
书名:深入浅出设计模式 数量:10
电影名:反恐24小时 数量:24 播放时间:200分钟
借给:张三