命令模式(Command)的目标是将一个请求封装成一个对象,因此可以参数化多个客户的不同请求,将请求排除,记录请求日志,并支持撤消操作。 结构图如下:
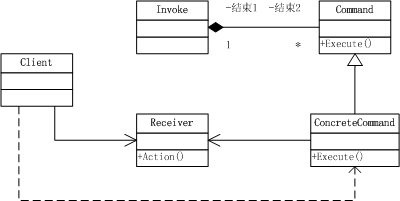
其实现思想是将一个请求封装到一个类中(Command),再提供接收对象(Receiver),最后Command命令由Invoker调用。
以一个电灯开关为例,命令的执行、不执行相对于开关的打开、关闭操作,由开关发出命令,电灯接收命令,结构图如下:
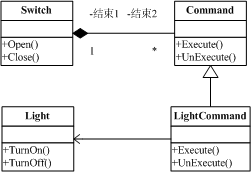
实现代码:
//Light.h
class Light
{
public:
Light();
virtual ~Light();
void TurnOn();
void TurnOff();
};
//Light.cpp
#include "stdafx.h"
#include "Light.h"
#include <iostream>
using namespace std;
Light::Light()
{
}
Light::~Light()
{
}
void Light::TurnOn()
{
cout << "电灯打开了" << endl;
}
void Light::TurnOff()
{
cout << "电灯关闭了" << endl;
}
//Command.h
class Command
{
public:
virtual ~Command();
virtual void Execute() = 0;
virtual void UnExecute() = 0;
protected:
Command();
};
//Command.cpp
#include "stdafx.h"
#include "Command.h"
Command::Command()
{
}
Command::~Command()
{
}
//LightCommand.h
#include "Command.h"
class Light;
class LightCommand : public Command
{
public:
LightCommand(Light*);
virtual ~LightCommand();
void Execute();
void UnExecute();
private:
Light* m_pLight;
};
//LightCommand.cpp
#include "stdafx.h"
#include "LightCommand.h"
#include "Light.h"
LightCommand::LightCommand(Light* pLight)
{
m_pLight = pLight;
}
LightCommand::~LightCommand()
{
if(m_pLight != NULL)
{
delete m_pLight;
m_pLight = NULL;
}
}
void LightCommand::Execute()
{
m_pLight->TurnOn();
}
void LightCommand::UnExecute()
{
m_pLight->TurnOff();
}
//Switch.h
class Command;
class Switch
{
public:
Switch(Command*);
virtual ~Switch();
void Open();
void Close();
private:
Command* m_pCommand;
};
//Switch.cpp
#include "stdafx.h"
#include "Switch.h"
#include "Command.h"
Switch::Switch(Command* pCommand)
{
m_pCommand = pCommand;
}
Switch::~Switch()
{
}
void Switch::Open()
{
m_pCommand->Execute();
}
void Switch::Close()
{
m_pCommand->UnExecute();
}
//main.cpp
#include "stdafx.h"
#include "Switch.h"
#include "Light.h"
#include "LightCommand.h"
int main(int argc, char* argv[])
{
Light* pLight = new Light;
Command* pCommand = new LightCommand(pLight);
Switch* pSwitch = new Switch(pCommand);
pSwitch->Open();
pSwitch->Close();
return 0;
}
最后输出为:
电灯打开了
电灯关闭了