组合模式(Composite)的定义为:组合多个对象形成树形结构,以表示整体-部分的结构层次。组合模式对单个对象和组合对象的使用具有一致性。其结构图如下:
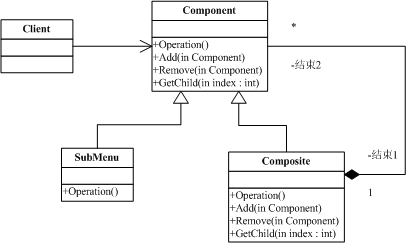
例如一个新闻的树形菜单,它包含很多原始菜单(如国内、国际),以及由其子菜单组成的组合节点(如国内新闻下的时事、社会等),结构图如下:
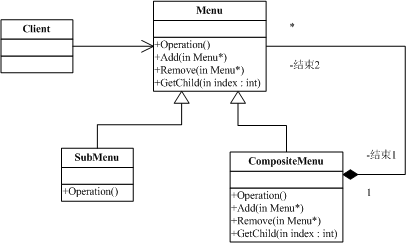
实现代码:
//Menu.h
#include <string>
class Menu
{
public:
virtual ~Menu();
virtual void Add(Menu*);
virtual void Remove(Menu*);
virtual Menu* GetChild(int);
virtual void Display() = 0;
protected:
Menu();
Menu(std::string);
std::string m_strName;
};
//Menu.cpp
#include "stdafx.h"
#include "Menu.h"
Menu::Menu()
{
}
Menu::Menu(std::string strName) : m_strName(strName)
{
}
Menu::~Menu()
{
}
void Menu::Add(Menu* pMenu)
{}
void Menu::Remove(Menu* pMenu)
{}
Menu* Menu::GetChild(int index)
{
return NULL;
}
//SubMenu.h
#include "Menu.h"
class SubMenu : public Menu
{
public:
SubMenu();
SubMenu(std::string);
virtual ~SubMenu();
void Display();
};
//SubMenu.cpp
#include "stdafx.h"
#include "SubMenu.h"
#include <iostream>
using namespace std;
SubMenu::SubMenu()
{
}
SubMenu::SubMenu(string strName) : Menu(strName)
{
}
SubMenu::~SubMenu()
{
}
void SubMenu::Display()
{
cout << m_strName << endl;
}
//CompositMenu.h
#include "Menu.h"
#include <vector>
class CompositMenu : public Menu
{
public:
CompositMenu();
CompositMenu(std::string);
virtual ~CompositMenu();
void Add(Menu*);
void Remove(Menu*);
Menu* GetChild(int);
void Display();
private:
std::vector<Menu*> m_vMenu;
};
//CompositMenu.cpp
#include "stdafx.h"
#include "CompositMenu.h"
#include <iostream>
using namespace std;
CompositMenu::CompositMenu()
{
}
CompositMenu::CompositMenu(string strName) : Menu(strName)
{
}
CompositMenu::~CompositMenu()
{
}
void CompositMenu::Add(Menu* pMenu)
{
m_vMenu.push_back(pMenu);
}
void CompositMenu::Remove(Menu* pMenu)
{
m_vMenu.erase(&pMenu);
}
Menu* CompositMenu::GetChild(int index)
{
return m_vMenu[index];
}
void CompositMenu::Display()
{
cout << "+" << m_strName << endl;
vector<Menu*>::iterator it = m_vMenu.begin();
for (; it != m_vMenu.end(); ++it)
{
cout << "|-";
(*it)->Display();
}
}
#include "stdafx.h"
#include "Menu.h"
#include "SubMenu.h"
#include "CompositMenu.h"
int main(int argc, char* argv[])
{
Menu* pMenu = new CompositMenu("国内新闻");
pMenu->Add(new SubMenu("时事新闻"));
pMenu->Add(new SubMenu("社会新闻"));
pMenu->Display();
pMenu = new CompositMenu("国际新闻");
pMenu->Add(new SubMenu("国际要闻"));
pMenu->Add(new SubMenu("环球视野"));
pMenu->Display();
return 0;
}
最后输出为:
+国内新闻
|-时事新闻
|-社会新闻
+国际新闻
|-国际要闻
|-环球视野