OpenGL: Texture Mapping: When we apply image to a geometric primitive, we call this texture or texture mapping.
Load texture image: glTexImage /glTexSubImage
Map textures to geometry: glTexCoord
Change the texture environment: glTexEnv
Set texture mapping parameters: glTexparameter
Generate mipmaps: gluBuildMipmaps
Manage multiple textures: glBindTexture
dramatic differnece: 戏剧性的.
richness: 丰富.
stark contrast: 对比.
texel: the individual elements in a texture.
pixel: the individual elements in a pixmap.
A one-to-one correspondence seldom exists between texels and pixels on the screen.
shrink: 收缩.
bias: 误差, 偏差.
Step on applying a texture map to geometry:
First: Load the texture into memory:
void glTexImage2D(GLenum target, GLint level,
GLint internalformat,
GLsizei width, GLsizei height,
GLint border, GLenum format,
GLenum type, void *data);
target: GL_TEXTURE_1D, GL_TEXTURE_2D, GL_TEXTURE_3D
level: specifies the mipmap level being loaded, for non-mipmaped texutres always to set this to 0.
internalformat: how many color components you want to store per texel.
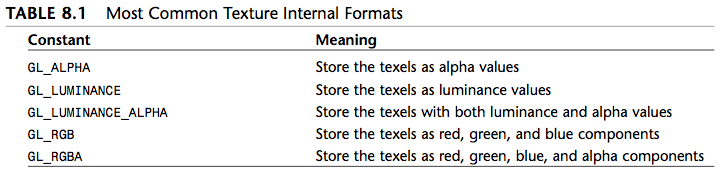
format: e.g. GL_RGB, GL_RGBA
type: e.g. GL_UNSIGNED_BYTE, GL_BYTE, GL_FLOAT,
data: picture data.
You should also be aware that OpenGL copies the texture information from data when you call one of these functions(after loading, delete the data array, the wanted data is copied into the memory). Loaded textures are not applied to geometry unless the appropriate texture state is enabled.
Second: specify texture coordinates.
glTexCoord2f(s, t);
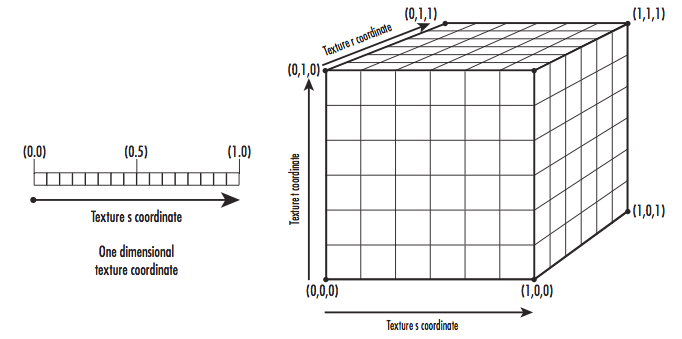
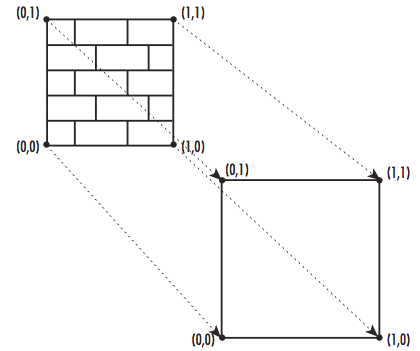
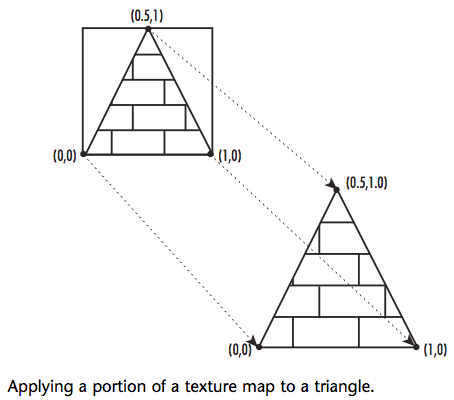
Texture matrix: glMatrixMode(GL_TEXTURE)
texture coordinates can also be transformated, rotated, and even scaled.
coordinate wrapping, texture filters, and the texture environment.
glTexImage2D(GL_TEXTURE_2D, 0, iComponents, iWidth, iHeight, 0, eFormat, GL_UNSIGNED_BYTE, pBytes);
free(pBytes);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE);
glTexEnvi(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_MODULATE);
glEnable(GL_TEXTURE_2D);
Texture environment: How OpenGL combines the colors from texels and the color of the underlying geometry.
glTexEnvi(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_MODULATE);
GL_MODULATE: The modulate environment mode multiplies the texel color by the geometry color (after lighting calculations).
GL_REPLACE: Simply replace the color of the underlying geometry.
GL_BLEND: If the texture has an alpha channel, you can enable blending. Otherwise GL_BLEND behavors the same way as GL_REPLACE.
Textures can also be blended with a constant blending color using the GL_BLENDtexture
environment. If you set this environment mode, you must also set the texture environ-
ment color:
GLfloat fColor[4] = { 1.0f, 0.0f, 0.0f, 0.0f };
glTexEnvi(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_BLEND);
glTexEnvfv(GL_TEXTURE_ENV, GL_TEXTURE_ENV_COLOR, fColor);
GL_ADD: Simply add the texel color values to the color of the underlying fragment.
Texture Parameters:
GL_TEXTURE_MAG_FILTER, GL_TEXTURE_MIN_FILTER
GL_NEAREST, GL_LINE
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_NEAREST);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST);
Texture Wrap(s, t):
Normally, you specify texture coordinates between 0.0 and 1.0 to map out the texels in a texture map. If texture coordinates fall out side this range, OpenGL handles them according to the current texture wrapping mode. You can set the wrap mode for each coordinate individually by calling glTexParameteri with GL_TEXTURE_WRAP_S, GL_TEXTURE_WRAP_T, or GL_TEXTURE_WRAP_R as the parameter name. The wrap mode can then be set to one of the following values: GL_REPEAT, GL_CLAMP, GL_CLAMP_TO_EDGE, or GL_CLAMP_TO_BORDER. The GL_REPEAT wrap mode simply causes the texture to repeat in the direction in which the texture coordinate has exceeded 1.0. The texture repeats again for every integer texture coordinate. This mode is very useful for applying a small tiled texture to large geometric surfaces.
Toon-shading, which is often refered to cell-shading, idea:
The basic idea is to use a surface normal from the geometry and a vector to the light source to find the intensity of the light striking the surface of the model. The dot product of these two vectors gives a value between 0.0 and 1.0 and is used as a one dimensional texture coordinate.
Mipmapping(many things in a small space):
Mipmappingis a powerful texturing technique that can improve both the rendering performance and the visual quality of a scene.
In essence, you load not a single image into the texture state, but a whole series of images from lagerest to smallest into a single "mipmapped" texture state. OpenGL use a new set of filter modes to choose the best-fitting texture or textures for given geometry.
Using a square set of mipmapps requires about one-third more memory than not using mipmapps.
Each one half the size of the previous image.
自动生成的, 质量比较差的Mipmaps:
gluBuild2DMipmaps: it automatically creates the scaled images for you and loads them appropriately with glTexImage.
int gluBuild2DMipmaps(GLenum target, GLint internalFormat,
GLint width, GLint height,
GLenum format, GLenum type, const void *data);
You should also be aware that using these functions may not produce mip level images with the same quality you can obtain with other tools such as Photoshop.
自动生成的, 质量比较好的Mipmaps:
With newer versions of the GLU library, you can also obtain a finer-grained control over which mip levels are loaded with these functions:
int gluBuild2DMipmapLevels(GLenum target, GLint internalFormat,
GLint width, GLint height,
GLenum format, GLenum type, GLint level,
GLint base, GLint max, const void *data);
With these functions, level is the mip level specified by the data parameter. This texture data is used to build mip levels base through max.
使用硬件加速生成Mipmaps(对于整个场境都使用mipmaps):
Hardware Generation of Mipmaps:
If you know beforehand that you want all mip levels loaded, you can also use OpenGL hardware acceleration to quickly generate all the necessary mip levels. You do so by setting the texture parameter GL_GENERATE_MIPMAP to GL_TRUE:
glTexParameteri(GL_TEXTURE_2D, GL_GENERATE_MIPMAP, GL_TRUE);
When this parameter is set, all calls to glTexImage or glTexSubImage that update the base texture map (mip level 0) automatically update all the lower mip levels. By making use of the graphics hardware, this feature is substantially faster than using gluBuildMipmaps. However, you should be aware that this feature was originally an extension and was promoted to the OpenGL core API only as of version 1.4. This is definitely the fastest and easiest way to build mipmaps on-the-fly.
Texture Objects:
Loading and maintaining the texture state occupies a considerable portion of many texture-heavy OpenGL applications (games in particular).
Texture objects allow you to load up more than one texture state at a time.
为texture对象分配内存:
You allocate a number of texture objects with the following function:
void glGenTextures(GLsizei n, GLuint *textures);
With this function, you specify the number of texture objects and a pointer to an array of unsigned integers that will be populated with the texture object identifiers.
指定texture对象(用一个整数来标志一个纹理对象):
void glBindTexture(GLenum target, GLuint texture);
The target parameter needs to specify GL_TEXTURE_1D, GL_TEXTURE_2D, or GL_TEXTURE_3D, and textureis the specific texture object to bind to. Hereafter, all texture loads and texture parameter settings affect only the currently bound texture object.
每一个texture都要设置自己的parameter, 因为每个parameter只对某个绑定的texture有效.
删除texture对象的内存空间, 释放内存:
To delete texture objects, you call the following function:
void glDeleteTextures(GLsizei n, GLuint *textures);
Calling glDeleteTextures multiple times may incur some delay, but only because you are deallocating possibly large amounts of texture memory.
测试是否有效的texture, 如被删除后:
You can test texture object names (or handles) to see whether they are valid by using the following function:
GLboolean glIsTexture(GLuint texture);
This function returns GL_TRUE if the integer is a previously allocated texture object name or GL_FALSE if not.