做网络模块的时候经常会遇到有关网址的处理.一般大致的情形是3种:
1.得到的是域名,如:www.3322.org
2.得到的是实际的IP地址,如:61.160.235.203
3.得到的是经过inet_addr处理过的IP,为unsigned long(DWORD)
一.那么如果是给出点分制的IP要转为DWORD型是如何转化呢?这个其实最简单,有专门的函数专门处理此事
unsigned long dwIP = inet_addr("222.212.12.77");
printf("IP(%s)->DWORD(%lu)\n");
//output
IP(222.212.12.77)->DWORD(1292686558)
二.第一种情况的逆转化
#include <stdio.h>
#include <stdlib.h>
#include <sys/select.h>
#include <netdb.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <errno.h>
#include <string.h>
int main(int argc, char *argv[])
{
struct in_addr net;
char tmp[16] = {0};
if(argc != 3)
{
printf("You MUST enter 3 varibal.No.1:func name No.2:case.No.3:ip(string or DWORD)\n");
return 0;
}
if(strcmp("1", argv[1]) == 0)
{
char* ip_string;
ip_string = argv[2];
unsigned long dword = inet_addr(ip_string);
printf("IP(%s)-->DWORD(%lu)\n", ip_string, dword);
}
else if(strcmp("2", argv[1]) == 0)
{
net.s_addr = (unsigned long)atol(argv[2]);
strcpy(tmp, inet_ntoa(net));
printf("DWORD(%s)-->IP(%s)\n",argv[2], tmp);
}
return 0;
}
这里给出一个点分制IP和DWORD相互转化的程序
三.如果给出的是域名而想得到点分制的IP呢?
这里给出一个接口,支持输入的类型是点分制和域名2中类型,返回的是DWORD型的IP
有一点要声明的是gethostbyname这个函数必须在网络连通的情况下才能正确完成域名的解析,你想,连个网都不通,它怎么解析?
#include <stdio.h>
#include <stdlib.h>
#include <sys/select.h>
#include <netdb.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <errno.h>
#include <string.h>
#define DWORD unsigned long
DWORD platform_domain2ip(const char *szDomain, char *szDotNumIP)
{
char szAddr[32] = {0};
struct hostent *pHost;
printf("input domain name(%s)\n", szDomain);
if((pHost = gethostbyname(szDomain)) == NULL)
{
printf("can not parse domain\n");
return -1;
}
printf("HostName :%s\n",pHost->h_name);
strcpy(szAddr, inet_ntoa(*((struct in_addr *)pHost->h_addr)));
printf("IP Address :%s\n", szAddr);
strcpy(szDotNumIP, szAddr);
return inet_addr(szAddr);
}
int main(int argc, char *argv[])
{
DWORD dwip;
char *ip = malloc(32);
//dwip = platform_domain2ip("www.3322.org", ip);
dwip = platform_domain2ip("61.160.235.203", ip);
printf("ip 1 (%s) 2 dw(%lu)\n", ip, dwip);
return 0;
}
//可以将main的注册分别打开来判断下结果是否正确,这里给出运行的结果,有图有真相
编译的命令再说下吧,怕有人不知道
gcc gethost.c –Wall –o gethost//在linux下
arm-hismall-linux-gcc gethost.c –Wall –o gethost//嵌入式环境下
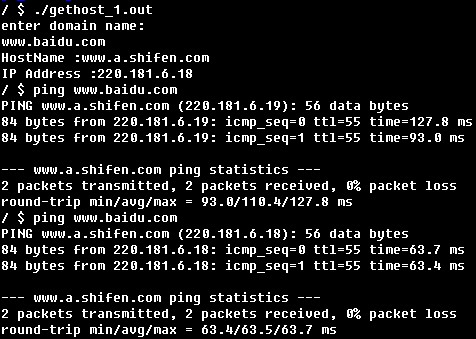
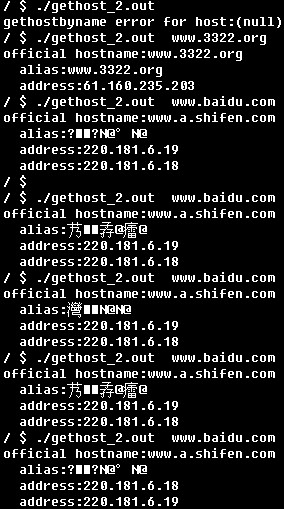
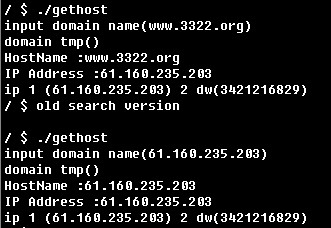
此接口已经在我的工程中使用,在平台IP的解析和3322的解析中得到了应用,所以是稳定可行的.
这3中IP的转化都了解了的话,那么网络编程不就扫除了一个大石头吗?呵呵,大家功能进步
网上比较流行的gethostbyname的例子如下,受到了启发
#include <stdio.h>
#include <stdlib.h>
#include <sys/select.h>
#include <netdb.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <errno.h>
#include <string.h>
int main(int argc, char **argv)
{
char *ptr,**pptr;
struct hostent *hptr;
char str[32];
/* 取得命令后第一个参数,即要解析的域名或主机名 */
ptr = argv[1];
/* 调用gethostbyname()。调用结果都存在hptr中 */
if((hptr = gethostbyname(ptr)) == NULL)
{
printf("gethostbyname error for host:%s\n", ptr);
return 1; /* 如果调用gethostbyname发生错误,返回1 */
}
printf("official hostname:%s\n",hptr->h_name);
/* 主机可能有多个别名,将所有别名分别打出来 */
for(pptr = hptr->h_aliases; *pptr != NULL; pptr++)
printf(" alias:%s\n",*pptr);
/* 根据地址类型,将地址打出来 */
switch(hptr->h_addrtype)
{
case AF_INET:
case AF_INET6:
pptr=hptr->h_addr_list;
/* 将刚才得到的所有地址都打出来。其中调用了inet_ntop()函数*/
for(;*pptr!=NULL;pptr++)
printf(" address:%s\n", inet_ntop(hptr->h_addrtype,*pptr, str, sizeof(str)));
break;
default:
printf("unknown address type\n");
break;
}
return 0;
}