引擎的粒子系统我一直在考虑,以前也设计过,但是感觉以前做过的过于复杂,这里我本着让粒子系统的接口尽可能的简单,功能尽可能的强大,我把粒子系统设计成了这样的形式:
1
2 typedef void(G_CALL *InitParticleFn)(Particle* p,float time);
3 typedef void(G_CALL *UpdateParticleFn)(Particle* p,float time);
4
5 ////////////////////////////////////////////////////////////
6 /// 定义引擎粒子系统描述符
7 ////////////////////////////////////////////////////////////
8 struct ParticleSystemDesc
9 {
10 ParticleSystemDesc():texture_id(0),
11 init_fn(NULL),
12 update_fn(NULL),
13 particle_number(2000),
14 life_span(8.0f),
15 birth_interval(life_span/(float)particle_number),
16 batch_particles(1),
17 particle_size(1.0f)
18 {
19 }
20 ~ParticleSystemDesc(){}
21
22 int texture_id;
23 InitParticleFn init_fn;
24 UpdateParticleFn update_fn;
25 int particle_number;
26 float life_span;
27 float birth_interval;
28 int batch_particles;
29 float particle_size;
30 };
31
32 ////////////////////////////////////////////////////////////
33 /// 定义引擎粒子系统类
34 ////////////////////////////////////////////////////////////
35 class ParticleSystem : NonCopyable, public Renderable
36 {
37 public:
38 ////////////////////////////////////////////////////////
39 /// 构造引擎粒子系统(传入的描述符必须要有粒子初始化和更新回调函数)
40 ////////////////////////////////////////////////////////
41 ParticleSystem(const ParticleSystemDesc &desc):desc(desc){}
42 ~ParticleSystem(){}
43 public:
44 void BeginRender(){}
45 void AfterRender(){}
46
47 protected:
48 ParticleSystemDesc desc;
49 };
提供了2个粒子回调函数一个是粒子初始话的回调函数-表明初始化粒子的方式
一个是粒子更新回调函数-表明如何更新一个例子
通过粒子系统描述符来作为用户和引擎的接口参数.
下面这是几幅贴图:
1.
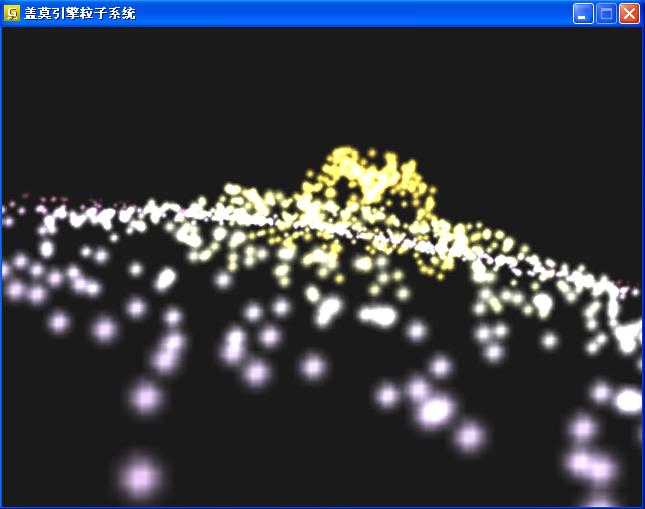
2.
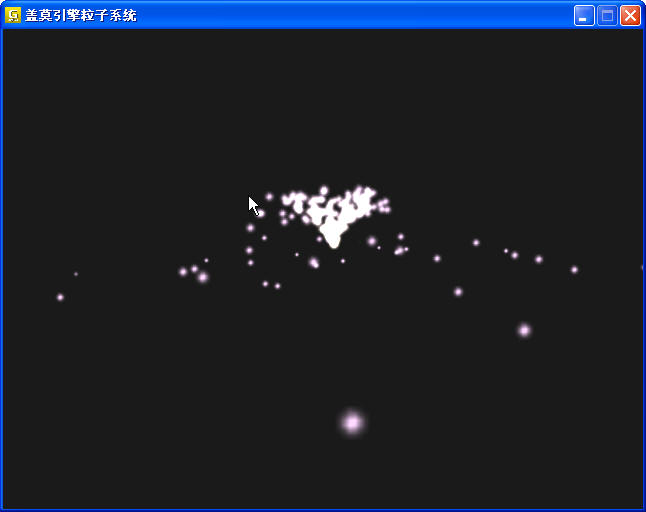
3.
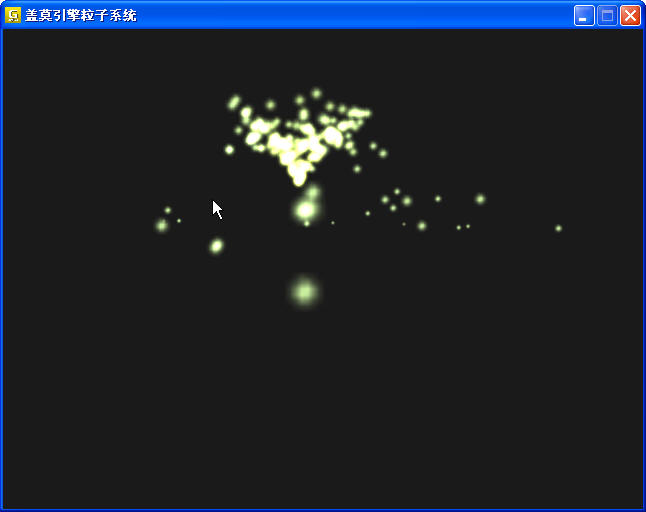
相关的代码如下:
1 #include <GEngine/Main.hpp>
2
3 #define WIN_WIDTH 640
4 #define WIN_HEIGHT 480
5
6 //! 粒子纹理
7 static const unsigned char particle_texture[] =
8 {
9 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
10 0x00, 0x00, 0x11, 0x22, 0x22, 0x11, 0x00, 0x00,
11 0x00, 0x11, 0x33, 0x88, 0x77, 0x33, 0x11, 0x00,
12 0x00, 0x22, 0x88, 0xff, 0xee, 0x77, 0x22, 0x00,
13 0x00, 0x22, 0x77, 0xee, 0xff, 0x88, 0x22, 0x00,
14 0x00, 0x11, 0x33, 0x77, 0x88, 0x33, 0x11, 0x00,
15 0x00, 0x00, 0x11, 0x33, 0x22, 0x11, 0x00, 0x00,
16 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
17 };
18
19 //! 粒子大小
20 #define PARTICLE_SIZE 0.7f
21
22 //! 粒子重力加速度
23 #define GRAVITY 9.8f
24
25 //! 粒子初始化速度
26 #define VELOCITY 8.0f
27
28 //! 粒子喷泉高度
29 #define FOUNTAIN_HEIGHT 3.0f
30
31 //! 粒子喷泉半径
32 #define FOUNTAIN_RADIUS 1.6f
33
34 //! 粒子纹理大小
35 #define P_TEX_WIDTH 8
36 #define P_TEX_HEIGHT 8
37
38 //! 粒子弹性碰撞摩擦力系数(1为无摩擦,0为最大摩擦)
39 #define FRICTION 0.75f
40
41 #define FOUNTAIN_R2 (FOUNTAIN_RADIUS+PARTICLE_SIZE/2)*(FOUNTAIN_RADIUS+PARTICLE_SIZE/2)
42
43 ////////////////////////////////////////////////////////////
44 /// 给出一个初始化粒子的方法
45 ////////////////////////////////////////////////////////////
46 void G_CALL InitParticle(core::Particle* p,float t);
47
48 ////////////////////////////////////////////////////////////
49 /// 更新粒子函数
50 ////////////////////////////////////////////////////////////
51 void G_CALL UpdateParticle(core::Particle* p,float time);
52
53 ////////////////////////////////////////////////////////////
54 /// 场景旋转和偏移
55 ////////////////////////////////////////////////////////////
56 float TransForm(double t);
57
58 ////////////////////////////////////////////////////////////
59 /// 构造纹理
60 ////////////////////////////////////////////////////////////
61 void BuildTexture(GLuint& texture_id);
62
63 ////////////////////////////////////////////////////////////
64 /// 渲染场景
65 ////////////////////////////////////////////////////////////
66 void RenderScene();
67
68 core::Device* device = NULL;
69 libmath::TriTable* table = NULL;
70
71 int main(int argc, char **argv)
72 {
73 int i;
74 double t0, t;
75
76 core::VideoMode mode;
77 mode.width = WIN_WIDTH;
78 mode.height = WIN_HEIGHT;
79
80 device = core::InitDevice("盖莫引擎粒子系统",false,mode);
81 table = device->GetTriTable();
82
83 core::ParticleSystemDesc desc;
84
85 GLuint texture_id;
86 BuildTexture(texture_id);
87 desc.texture_id = texture_id;
88 desc.init_fn = &InitParticle;
89 desc.update_fn = &UpdateParticle;
90 desc.batch_particles = 80;
91 desc.particle_size = 0.6f;
92 desc.life_span = 8;
93
94 core::ParticleSystem* ps = device->GetParticleSystem(desc);
95
96 t0 = device->GetTime();
97 BEGIN_LOOP(device)
98 t = device->GetTime() - t0;
99 RenderScene();
100 float dt = TransForm(t);
101 ps->Render();
102 END_LOOP(device)
103
104 device->Close();
105 device->Drop();
106
107 return 0;
108 }
109
110 void BuildTexture(GLuint& texture_id)
111 {
112 glGenTextures( 1, &texture_id );
113 glBindTexture( GL_TEXTURE_2D, texture_id);
114 glPixelStorei( GL_UNPACK_ALIGNMENT, 1 );
115 glTexParameteri( GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP );
116 glTexParameteri( GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP );
117 glTexParameteri( GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR );
118 glTexParameteri( GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR );
119 glTexImage2D(GL_TEXTURE_2D, 0, GL_LUMINANCE, P_TEX_WIDTH, P_TEX_HEIGHT,
120 0, GL_LUMINANCE, GL_UNSIGNED_BYTE, particle_texture);
121 }
122
123 ////////////////////////////////////////////////////////////
124 /// 场景旋转和偏移
125 ////////////////////////////////////////////////////////////
126 float TransForm(double t)
127 {
128 double xpos, ypos, zpos, angle_x, angle_y, angle_z;
129 static double t_old = 0.0;
130 float dt = (float)(t-t_old);
131 t_old = t;
132
133 angle_x = 90.0 - 10.0;
134 angle_y = 10.0 * sin( 0.3 * t );
135 angle_z = 10.0 * t;
136 glRotated( -angle_x, 1.0, 0.0, 0.0 );
137 glRotated( -angle_y, 0.0, 1.0, 0.0 );
138 glRotated( -angle_z, 0.0, 0.0, 1.0 );
139
140 xpos = 15.0 * sin( (M_PI/180.0) * angle_z ) +
141 2.0 * sin( (M_PI/180.0) * 3.1 * t );
142 ypos = -15.0 * cos( (M_PI/180.0) * angle_z ) +
143 2.0 * cos( (M_PI/180.0) * 2.9 * t );
144 zpos = 4.0 + 2.0 * cos( (M_PI/180.0) * 4.9 * t );
145 glTranslated( -xpos, -ypos, -zpos );
146 return dt;
147 }
148
149 void RenderScene()
150 {
151 glViewport( 0, 0, WIN_WIDTH,WIN_HEIGHT);
152 glClearColor( 0.1f, 0.1f, 0.1f, 1.0f );
153 glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT );
154 glMatrixMode( GL_PROJECTION );
155 glLoadIdentity();
156 gluPerspective(65.0, 640.0/480.0, 1.0, 60.0 );
157 glMatrixMode( GL_MODELVIEW );
158 glLoadIdentity();
159 }
160
161 ////////////////////////////////////////////////////////////
162 /// 给出一个初始化粒子的方法(t为粒子系统启动时间(单位:秒))
163 ////////////////////////////////////////////////////////////
164 void G_CALL InitParticle(core::Particle* p,float t)
165 {
166 //! 初始化粒子位置
167 p->position = Vector3f(0,0,FOUNTAIN_HEIGHT);
168
169 //! 设定粒子初始z轴速度
170 p->velocity.z = 0.7f + (0.3/4096.0) * (float) (rand() & 4095);
171
172 //! 获取随机xy平面旋转角度
173 float xy_angle = (2.0*M_PI/4096.0) * (float) (rand() & 4095);
174 p->velocity.x = 0.45f * table->CosTable(xy_angle);
175 p->velocity.y = 0.45f * table->SinTable(xy_angle);
176
177 //! 根据时间调整粒子初始速度
178 float velocity = VELOCITY*(0.8f + 0.1f*(float)(table->SinTable(0.5*t)+table->SinTable(0.31*t)));
179 p->velocity.x *= velocity;
180 p->velocity.y *= velocity;
181 p->velocity.z *= velocity;
182
183 //! 设定粒子初始颜色
184 p->color.red = 0.7f + 0.3f * table->SinTable(0.34*t + 0.1);
185 p->color.green = 0.6f + 0.4f * table->SinTable(0.63*t + 1.1);
186 p->color.blue = 0.6f + 0.4f * table->SinTable(0.91*t + 2.1);
187 }
188
189 ////////////////////////////////////////////////////////////
190 /// 更新粒子函数
191 ////////////////////////////////////////////////////////////
192 void G_CALL UpdateParticle(core::Particle* p,float time)
193 {
194 float dt = time;
195 //! 修正粒子生命
196 p->life = p->life - dt * (1.0f / 8.0f);
197 //! 修正粒子速度
198 p->velocity.z = p->velocity.z - GRAVITY *dt;
199 p->position += p->velocity * dt;
200
201 //! 处理简单的碰撞
202 if( p->velocity.z < 0.0f )
203 {
204 if((p->position.x*p->position.x + p->position.y*p->position.y) < FOUNTAIN_R2 &&
205 p->position.z < (FOUNTAIN_HEIGHT + PARTICLE_SIZE/2) )
206 {
207 p->velocity.z = -FRICTION * p->velocity.z;
208 p->position.z = FOUNTAIN_HEIGHT + PARTICLE_SIZE/2 +
209 FRICTION * (FOUNTAIN_HEIGHT +
210 PARTICLE_SIZE/2 - p->position.z);
211 }
212
213 //! 当粒子碰撞到地面应该跳起来
214 else if( p->position.z < PARTICLE_SIZE/2 )
215 {
216 p->velocity.z = -FRICTION * p->velocity.z;
217 p->position.z = PARTICLE_SIZE/2 + FRICTION * (PARTICLE_SIZE/2 - p->position.z);
218 }
219 }
220 }
221
222
这个场景例子的原型源于glfw库附带粒子