原型模式的定义:用原型实例指定创建对象的种类,并且通过拷贝这些原型创建新的对象。
UML图:
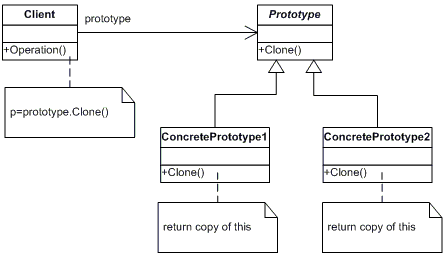
C++代码:
//filename: prototype.h
//author: Qinglong.mark.He
#include <iostream>
#include <string>
class Prototype
{
protected:
std::string name;
public:
Prototype(){}
virtual ~Prototype(){}
virtual Prototype *clone(){return nullptr;}
virtual void set(char *n){}
virtual void show(){}
};
class PrototypeA: public Prototype
{
public:
PrototypeA(){
name = "PrototypeA";
}
PrototypeA(const PrototypeA &r){
name = r.name;
}
~PrototypeA(){
}
PrototypeA *clone(){
return new PrototypeA(*this);
}
void show(){
std::cout<<"PrototypeA name: "<<name<<std::endl;
}
};
class PrototypeB: public Prototype
{
public:
PrototypeB(){
name = "PrototypeB";
}
PrototypeB(const PrototypeB &r){
name = r.name;
}
~PrototypeB(){
}
PrototypeB *clone(){
return new PrototypeB(*this);
}
void show(){
std::cout<<"PrototypeB name: "<<name<<std::endl;
}
};
测试用例:
#include "prototype.h"
int main(){
auto r1 = new PrototypeA;
auto r2 = new PrototypeB;
auto r3 = r1->clone();
auto r4 = r2->clone();
r1->show();
r2->show();
delete r1;
delete r2;
r3->show();
r4->show();
delete r3;
delete r4;
return 0;
}