来自于《大话设计模式》
组合模式(Composite):将对象组合成树形结构以表示‘部分-整体’的层次结构。组合模式使得用户对单个对象和组合对象的使用具有一致性。
UML 类图:
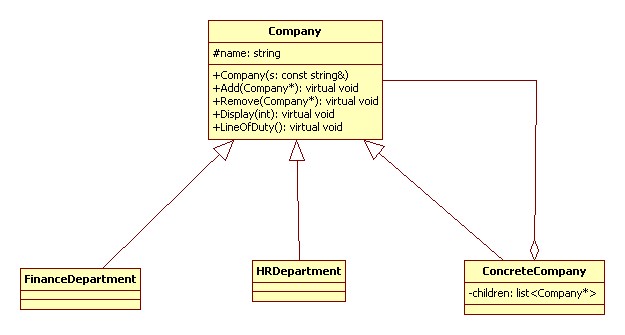
代码实现 C++:
1 #include <iostream>
2 #include <string>
3 #include <list>
4 #include <algorithm>
5 using namespace std;
6
7 class Company
8 {
9 protected:
10 string name;
11 public:
12 Company(const string& s) : name(s) {}
13 virtual void Add(Company* c) = 0;
14 virtual void Remove(Company* c) = 0;
15 virtual void Display(int depth) = 0;
16 virtual void LineOfDuty() = 0;
17 };
18
19 class ConcreteCompany : public Company
20 {
21 private:
22 list<Company*> children;
23 public:
24 ConcreteCompany(const string& s) : Company(s) {}
25 virtual void Add(Company* c)
26 {
27 children.push_back(c);
28 }
29 virtual void Remove(Company* c)
30 {
31 list<Company*>::iterator iter = find(children.begin(), children.end(), c);
32 if (iter != children.end())
33 {
34 children.erase(iter);
35 }
36 }
37 virtual void Display(int depth)
38 {
39 string str(depth, '-');
40 str += name;
41 cout << str << endl;
42 for (list<Company*>::iterator iter = children.begin(); iter != children.end(); ++iter)
43 {
44 (*iter)->Display(depth + 2);
45 }
46 }
47 virtual void LineOfDuty()
48 {
49 for (list<Company*>::iterator iter = children.begin(); iter != children.end(); ++iter)
50 {
51 (*iter)->LineOfDuty();
52 }
53 }
54 };
55
56 class HRDepartment : public Company
57 {
58 public:
59 HRDepartment(const string& s) : Company(s) {}
60 virtual void Add(Company* c) {}
61 virtual void Remove(Company* c) {}
62 virtual void Display(int depth)
63 {
64 string str(depth, '-');
65 str += name;
66 cout << str << endl;
67 }
68 virtual void LineOfDuty()
69 {
70 cout << name << " 员工招聘培训管理!" << endl;
71 }
72 };
73
74 class FinanceDepartment : public Company
75 {
76 public:
77 FinanceDepartment(const string& s) : Company(s) {}
78 virtual void Add(Company* c) {}
79 virtual void Remove(Company* c) {}
80 virtual void Display(int depth)
81 {
82 string str(depth, '-');
83 str += name;
84 cout << str << endl;
85 }
86 virtual void LineOfDuty()
87 {
88 cout << name << " 公司财务收支管理!" << endl;
89 }
90 };
91
92 int main()
93 {
94 ConcreteCompany root("北京总公司");
95 root.Add(new HRDepartment("总公司人力资源部"));
96 root.Add(new FinanceDepartment("总公司财务部"));
97
98 ConcreteCompany* comp = new ConcreteCompany("上海华东分公司");
99 comp->Add(new HRDepartment("华东分公司人力资源部"));
100 comp->Add(new FinanceDepartment("华东分公司财务部"));
101 root.Add(comp);
102
103 ConcreteCompany* comp2 = new ConcreteCompany("南京办事处");
104 comp2->Add(new HRDepartment("南京办事处人力资源部"));
105 comp2->Add(new FinanceDepartment("南京办事处财务部"));
106 root.Add(comp2);
107
108 ConcreteCompany* comp3 = new ConcreteCompany("杭州办事处");
109 comp3->Add(new HRDepartment("杭州办事处人力资源部"));
110 comp3->Add(new FinanceDepartment("杭州办事处财务部"));
111 root.Add(comp3);
112
113 cout << "结构图:" << endl;
114 root.Display(1);
115
116 cout << "职责:" << endl;
117 root.LineOfDuty();
118
119 return 0;
120 }
posted on 2011-04-29 16:14
unixfy 阅读(213)
评论(0) 编辑 收藏 引用