来自于《大话设计模式》
代理模式(Proxy):为其他对象提供一种代理以控制对这个对象的访问。
代理模式的应用:
·远程代理
·虚拟代理
·安全代理
·智能指针
UML 图:
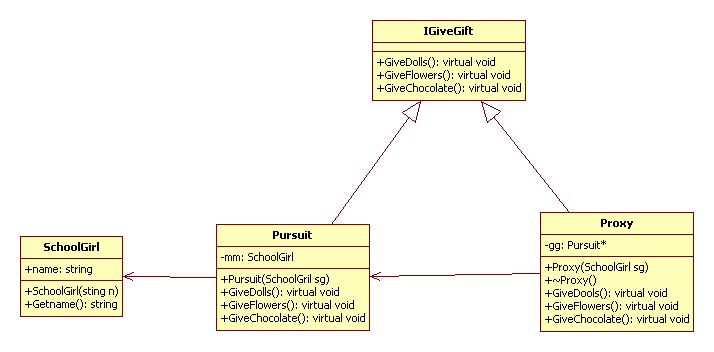
代码实现 C++:
1 #include <iostream>
2 #include <string>
3 using namespace std;
4
5 class SchoolGirl
6 {
7 private:
8 string name;
9 public:
10 SchoolGirl(string n) : name(n) {}
11 string GetName()
12 {
13 return name;
14 }
15 };
16
17 class IGiveGift
18 {
19 public:
20 virtual void GiveDolls() = 0;
21 virtual void GiveFlowers() = 0;
22 virtual void GiveChocolate() = 0;
23 };
24
25 class Pursuit : public IGiveGift
26 {
27 private:
28 SchoolGirl mm;
29 public:
30 Pursuit(SchoolGirl sg) : mm(sg) {}
31 virtual void GiveDolls()
32 {
33 cout << mm.GetName() << " 送你洋娃娃" << endl;
34 }
35 virtual void GiveFlowers()
36 {
37 cout << mm.GetName() << " 送你鲜花" << endl;
38 }
39 virtual void GiveChocolate()
40 {
41 cout << mm.GetName() << " 送你巧克力" << endl;
42 }
43 };
44
45 class Proxy : public IGiveGift
46 {
47 private:
48 Pursuit* gg;
49 public:
50 Proxy(SchoolGirl sg)
51 {
52 gg = new Pursuit(sg);
53 }
54 ~Proxy()
55 {
56 delete gg;
57 }
58 virtual void GiveDolls()
59 {
60 gg->GiveDolls();
61 }
62 virtual void GiveFlowers()
63 {
64 gg->GiveFlowers();
65 }
66 virtual void GiveChocolate()
67 {
68 gg->GiveChocolate();
69 }
70 };
71
72 int main()
73 {
74 SchoolGirl mm("Lili");
75
76 Proxy* pp = new Proxy(mm);
77 pp->GiveDolls();
78 pp->GiveFlowers();
79 pp->GiveChocolate();
80
81 delete pp;
82 return 0;
83 }
posted on 2011-04-23 18:44
unixfy 阅读(246)
评论(0) 编辑 收藏 引用