来自于《大话设计模式》
如果需要添加其他操作,需要
·添加一个操作类
·修改 OperationFactory
·修改客户代码以使用这个操作
UML 类图如下:
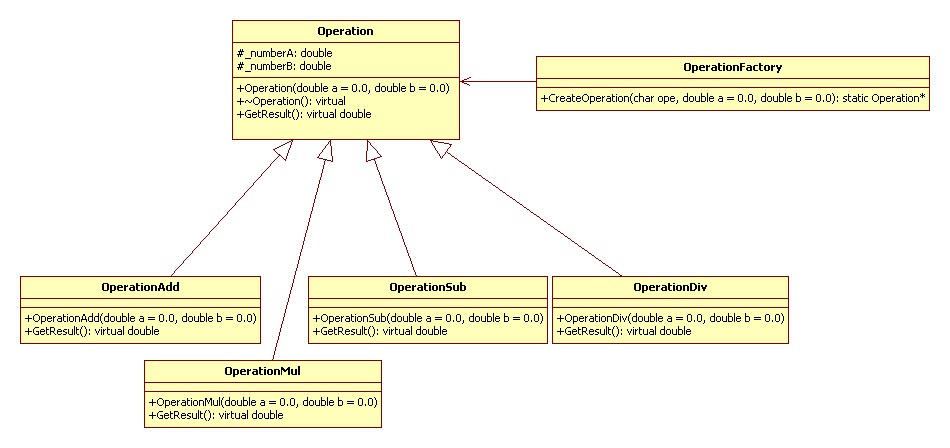
实例代码 C++:
1 #include <iostream>
2 using namespace std;
3
4 class Operation
5 {
6 protected:
7 double _numberA;
8 double _numberB;
9 public:
10 Operation(double a = 0.0, double b = 0.0) : _numberA(a), _numberB(b) {}
11 virtual ~Operation() {}
12 virtual double GetResult()
13 {
14 double result = 0.0;
15 return result;
16 }
17 };
18
19 class OperationAdd : public Operation
20 {
21 public:
22 OperationAdd(double a = 0.0, double b = 0.0) : Operation(a, b) {}
23 virtual double GetResult()
24 {
25 return _numberA + _numberB;
26 }
27 };
28
29 class OperationSub : public Operation
30 {
31 public:
32 OperationSub(double a = 0.0, double b = 0.0) : Operation(a, b) {}
33 virtual double GetResult()
34 {
35 return _numberA - _numberB;
36 }
37 };
38
39 class OperationMul : public Operation
40 {
41 public:
42 OperationMul(double a = 0.0, double b = 0.0) : Operation(a, b) {}
43 virtual double GetResult()
44 {
45 return _numberA * _numberB;
46 }
47 };
48
49 class OperationDiv : public Operation
50 {
51 public:
52 OperationDiv(double a = 0.0, double b = 0.0) : Operation(a, b) {}
53 virtual double GetResult()
54 {
55 if (_numberB == 0.0)
56 {
57 throw runtime_error("Denominator is 0!");
58 }
59 return _numberA / _numberB;
60 }
61 };
62
63 class OperationFactory
64 {
65 public:
66 static Operation* CreateOperation(char ope, double a = 0.0, double b = 0.0)
67 {
68 Operation* ret = 0;
69 switch (ope)
70 {
71 case '+':
72 ret = new OperationAdd(a, b);
73 break;
74 case '-':
75 ret = new OperationSub(a, b);
76 break;
77 case '*':
78 ret = new OperationMul(a, b);
79 break;
80 case '/':
81 ret = new OperationDiv(a, b);
82 break;
83 default:
84 break;
85 }
86 return ret;
87 }
88 };
89
90 int main()
91 {
92 try
93 {
94 Operation* p_ope;
95 p_ope = OperationFactory::CreateOperation('+', 3.0, 5.0);
96 if (p_ope != 0)
97 {
98 cout << p_ope->GetResult() << endl;
99 delete p_ope;
100 p_ope = 0;
101 }
102
103 p_ope = OperationFactory::CreateOperation('-', 3.0, 5.0);
104 if (p_ope != 0)
105 {
106 cout << p_ope->GetResult() << endl;
107 delete p_ope;
108 p_ope = 0;
109 }
110
111 p_ope = OperationFactory::CreateOperation('*', 3.0, 5.0);
112 if (p_ope != 0)
113 {
114 cout << p_ope->GetResult() << endl;
115 delete p_ope;
116 p_ope = 0;
117 }
118
119 p_ope = OperationFactory::CreateOperation('/', 3.0, 5.0);
120 if (p_ope != 0)
121 {
122 cout << p_ope->GetResult() << endl;
123 delete p_ope;
124 p_ope = 0;
125 }
126
127 p_ope = OperationFactory::CreateOperation('/', 3.0, 0.0);
128 if (p_ope != 0)
129 {
130 cout << p_ope->GetResult() << endl;
131 delete p_ope;
132 p_ope = 0;
133 }
134 }
135 catch (const exception& e)
136 {
137 cerr << e.what() << endl;
138 }
139 return 0;
140 }
posted on 2011-04-21 14:19
unixfy 阅读(202)
评论(0) 编辑 收藏 引用