使用说明:
1.在客户区单击鼠标左键,系统记录下点击的位置
2.按住鼠标左键的时候,可以移动鼠标,系统会根据
鼠标移动的速度,记录一系列的点
3.按下回车的时候,程序将各个点连接起来
4.单击鼠标右键,程序重新绘制,并更新客户区
----------------------------------------------------
下载:
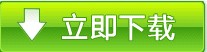
----------------------------------------------------
源代码:
1 /*
2 Written by 徐继开
3 Date: 2012 / 08 /25
4 */
5 #include <Windows.h>
6 LRESULT CALLBACK WndProc(HWND,UINT,WPARAM,LPARAM);
7
8
9 static TCHAR szAppName[]=TEXT("BtnLook");
10 HWND hwnd;
11 MSG msg;
12
13
14 //创建窗口
15 HWND DIYCreateWindow(HINSTANCE hInstance,int iCmdShow);
16
17 int WINAPI WinMain(HINSTANCE hInstance,HINSTANCE hPrevInstance,
18 PSTR szCmdLine,int iCmdShow)
19 {
20 hwnd=DIYCreateWindow(hInstance,iCmdShow);
21 while (GetMessage (&msg,NULL,0,0))
22 {
23 TranslateMessage(&msg);
24 DispatchMessage(&msg);
25
26 }
27 return msg.wParam;
28
29
30 }
31
32
33 HWND DIYCreateWindow(HINSTANCE hInstance,int iCmdShow){
34
35 WNDCLASS wndclass;
36 wndclass.style =CS_HREDRAW|CS_VREDRAW;
37 wndclass.lpfnWndProc=WndProc;
38 wndclass.cbClsExtra=0;
39 wndclass.cbWndExtra=0;
40 wndclass.hInstance=hInstance;
41 wndclass.hIcon=LoadIcon(NULL,IDI_APPLICATION);
42 wndclass.hCursor=LoadCursor(NULL,IDC_ARROW);
43 wndclass.hbrBackground=(HBRUSH)GetStockObject(WHITE_BRUSH);
44 wndclass.lpszMenuName=NULL;
45 wndclass.lpszClassName=szAppName;
46
47 if (!RegisterClass(&wndclass))
48 {
49 MessageBox(NULL,TEXT("This program requries Windows NT!"),
50 szAppName,MB_ICONERROR);
51 return 0;
52 }
53
54 hwnd = CreateWindow(szAppName,TEXT("Button Look"),
55 WS_OVERLAPPEDWINDOW,
56 CW_USEDEFAULT,CW_USEDEFAULT,
57 CW_USEDEFAULT,CW_USEDEFAULT,
58 NULL,NULL,hInstance,NULL);
59 ShowWindow(hwnd,iCmdShow);
60 UpdateWindow (hwnd) ;
61
62 }
63
64 LRESULT CALLBACK WndProc(HWND hwnd, UINT message ,WPARAM wParam,LPARAM lParam)
65 {
66 static TCHAR szSTR[]=TEXT("BtnLook");
67 static POINT pt[100000];
68 static int iCount;
69 HDC hdc;
70 int i,j;
71 int x,y;
72 PAINTSTRUCT ps;
73
74 switch (message)
75 {
76 case WM_CREATE:
77 iCount = 0;
78 return 0;
79
80 case WM_LBUTTONDOWN://处理鼠标左键按下的消息
81 x = LOWORD (lParam);
82 y = HIWORD (lParam);
83 pt[iCount ].x = LOWORD (lParam);
84 pt[iCount++].y = HIWORD (lParam);
85
86 hdc = GetDC(hwnd);
87 Ellipse(hdc , x-5 , y-5 , x+5 , y+5);
88 //SetPixel(hdc, LOWORD(lParam) ,HIWORD(lParam) , #7b20ff);
89 ReleaseDC(hwnd , hdc);
90
91 return 0;
92
93 case WM_KEYDOWN ://按下回车的时候让窗口重绘
94 switch(wParam)
95 {
96 case VK_RETURN:
97 hdc = GetDC(hwnd);
98 TextOut(hdc , 200 ,200 ,szSTR, 3);
99 InvalidateRect(hwnd ,NULL , TRUE);
100 ReleaseDC(hwnd , hdc);
101 break;
102 }
103 return 0;
104
105 case WM_MOUSEMOVE:
106 if(wParam & MK_LBUTTON && iCount < 100000)
107 {
108 x = LOWORD (lParam);
109 y = HIWORD (lParam);
110 pt[iCount ].x = x;
111 pt[iCount++].y = y;
112
113 hdc = GetDC(hwnd);
114 Ellipse(hdc , x-2 , y-2 , x+2 , y+2);
115 //SetPixel(hdc, LOWORD(lParam) ,HIWORD(lParam) , #7b20ff);
116 ReleaseDC(hwnd , hdc);
117 }
118 return 0;
119
120 case WM_RBUTTONDOWN:
121 iCount = 0;
122 InvalidateRect(hwnd , NULL, TRUE);
123 return 0;
124
125 case WM_PAINT:
126 hdc = BeginPaint(hwnd ,&ps);
127
128 SetCursor(LoadCursor(NULL, IDC_WAIT));
129 ShowCursor(TRUE);
130 SelectObject(hdc,CreatePen(PS_SOLID,1,#9b7ba0));
131 for(i = 0 ; i < iCount - 1 ; ++i)
132 for(j = i +1 ;j < iCount ; ++j)
133 {
134 MoveToEx(hdc , pt[i].x , pt[i].y , NULL) ;
135 LineTo (hdc , pt[j].x , pt[j].y) ;
136 }
137
138 ShowCursor (FALSE) ;
139 SetCursor (LoadCursor (NULL , IDC_ARROW) ) ;
140 EndPaint(hwnd , &ps);
141 return 0;
142 case WM_DESTROY:
143 PostQuitMessage(0);
144 return 0;
145 }
146 return DefWindowProc (hwnd , message ,wParam ,lParam);
147 }
148