1 // bmp_converter_2.cpp : Defines the entry point for the application.
2 //
3
4 #include "stdafx.h"
5 // Primitive BMP to ASCII art generator
6 // Reads source.bmp and outputs art.txt
7 // Source must be 24-bit .bmp
8
9 #include <iostream.h>
10 #include <windows.h>
11
12 #define MAX_SHADES 10
13
14 BITMAPFILEHEADER bfh;
15 BITMAPINFOHEADER bih;
16 RGBTRIPLE *image;
17 DWORD written;
18 HANDLE hfile;
19 int imagesize;
20 char shades[MAX_SHADES] = {'#','$','O','=','+','|','-','^','.',' '};
21 char return1 = 0x0D;
22 char return2 = 0x0A;
23 int needle = 0;
24 int average_color = 0;
25
26 int APIENTRY WinMain(HINSTANCE hInstance,
27 HINSTANCE hPrevInstance,
28 LPSTR lpCmdLine,
29 int nCmdShow)
30 {
31 // Open a channel to source file
32 hfile = CreateFile("source.bmp",GENERIC_READ,FILE_SHARE_READ,NULL,OPEN_EXISTING,NULL,NULL);
33 // Read header
34 ReadFile(hfile,&bfh,sizeof(bfh),&written,NULL);
35 ReadFile(hfile,&bih,sizeof(bih),&written,NULL);
36 // Read image
37 imagesize = bih.biSizeImage;
38 image = new RGBTRIPLE[imagesize];
39 ReadFile(hfile,image,imagesize*sizeof(RGBTRIPLE),&written,NULL);
40 // Close source file
41 CloseHandle(hfile);
42 // Open channel to output
43 hfile = CreateFile("art.txt",GENERIC_WRITE,NULL,NULL,CREATE_ALWAYS,FILE_ATTRIBUTE_NORMAL,NULL);
44 // Keeping in mind the image is upside down, convert and write it
45 // It down-samples the image a bit in res too
46 for(int y = bih.biHeight-1;y >= 0;y--)
47 {
48 for(int x = 0;x < bih.biWidth;x++)
49 {
50 // Get the average color
51 average_color = (image[x+y*bih.biWidth].rgbtBlue+image[x+y*bih.biWidth].rgbtRed+image[x+y*bih.biWidth].rgbtGreen)/3;
52 // Convert to a shade of 8
53 average_color /= (256/MAX_SHADES);
54 if(average_color >= MAX_SHADES)
55 average_color -= 1;
56 // Output
57 WriteFile(hfile,&shades[average_color],1,&written,NULL);
58 //WriteFile(hfile,&shades[average_color],1,&written,NULL);
59 }
60 WriteFile(hfile,&return1,1,&written,NULL);
61 WriteFile(hfile,&return2,1,&written,NULL);
62 }
63 // Close handle to output
64 CloseHandle(hfile);
65 return 0;
66 }
67
68
This code is just a rough implementation to convert a small .bmp file to text file.
It needs to be improved to be fit with bigger file or monofile.
Compile and debug tool: VC++ 6, Win32 Simple Application.
Below is the testing result. The output txt file is often italic if the width is not dividable by 8 well.
Source.bmp (Must be placed in the project directory)
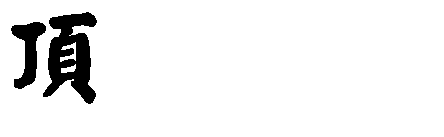
Output txt displayed as(only a small part of the whole pic):
#
=#########=
###############-
-###############=
=##### ###############-
=##########= -######-
###########= -###=
=#########= =###- =#=
-####### #########-
-####= -###########=
-###- =#######=####
-###- #####= -####
-###- -#### -=-####-
-###= -########-####-
-###= -#######--####-
-###= -###- -####-
-###= =###--###=####=
-###= =#############=
-###= =####### -####=
=###- =###= =####-
=###- -###= -#######-
-####- #############-
-##### =############-
######= -#### ######
#####- #### #####=
=##- #### -#####=
=### -#####=
-### -#####-
-#- =####-
=##=
##-