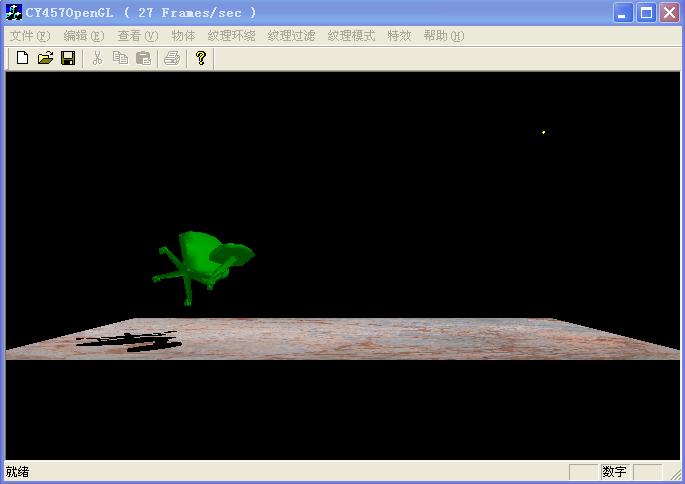
本文将介绍如何从Obj文件格式中创建3D对象,我们使用的是Nate Miller的obj格式加载类。
This would be very useful to create large Virtual Reality applications as we could make use of the readily available 3D model files or make use of modeling tools to create these models and load them instead of creating them programatically. The .obj format is a very simple and popular format and files of other types such 3D Studio (.3ds) can be exported to this format or converted using tools such as 3D Exploration. This .obj loading code cannot read textures, it can only also read .mtl files in addition to the .obj file and thus make use of material data too.
1, Nate Miller的obj文件加载类,其完整源代码可以从http://www.pobox.com/~ndr处下载。

Glm头文件
/*
* Wavefront .obj file format reader.
*
* author: Nate Robins
* email: ndr@pobox.com
* www: http://www.pobox.com/~ndr
*/
/* includes */
#include <GL/glut.h>
#ifndef M_PI
#define M_PI 3.14159265
#endif
/* defines */
#define GLM_NONE (0) /* render with only vertices */
#define GLM_FLAT (1 << 0) /* render with facet normals */
#define GLM_SMOOTH (1 << 1) /* render with vertex normals */
#define GLM_TEXTURE (1 << 2) /* render with texture coords */
#define GLM_COLOR (1 << 3) /* render with colors */
#define GLM_MATERIAL (1 << 4) /* render with materials */
/* structs */
/* GLMmaterial: Structure that defines a material in a model.
*/
typedef struct _GLMmaterial
{
char* name; /* name of material */
GLfloat diffuse[4]; /* diffuse component */
GLfloat ambient[4]; /* ambient component */
GLfloat specular[4]; /* specular component */
GLfloat emmissive[4]; /* emmissive component */
GLfloat shininess; /* specular exponent */
} GLMmaterial;
/* GLMtriangle: Structure that defines a triangle in a model.
*/
typedef struct {
GLuint vindices[3]; /* array of triangle vertex indices */
GLuint nindices[3]; /* array of triangle normal indices */
GLuint tindices[3]; /* array of triangle texcoord indices*/
GLuint findex; /* index of triangle facet normal */
} GLMtriangle;
/* GLMgroup: Structure that defines a group in a model.
*/
typedef struct _GLMgroup {
char* name; /* name of this group */
GLuint numtriangles; /* number of triangles in this group */
GLuint* triangles; /* array of triangle indices */
GLuint material; /* index to material for group */
struct _GLMgroup* next; /* pointer to next group in model */
} GLMgroup;
/* GLMmodel: Structure that defines a model.
*/
typedef struct {
char* pathname; /* path to this model */
char* mtllibname; /* name of the material library */
GLuint numvertices; /* number of vertices in model */
GLfloat* vertices; /* array of vertices */
GLuint numnormals; /* number of normals in model */
GLfloat* normals; /* array of normals */
GLuint numtexcoords; /* number of texcoords in model */
GLfloat* texcoords; /* array of texture coordinates */
GLuint numfacetnorms; /* number of facetnorms in model */
GLfloat* facetnorms; /* array of facetnorms */
GLuint numtriangles; /* number of triangles in model */
GLMtriangle* triangles; /* array of triangles */
GLuint nummaterials; /* number of materials in model */
GLMmaterial* materials; /* array of materials */
GLuint numgroups; /* number of groups in model */
GLMgroup* groups; /* linked list of groups */
GLfloat position[3]; /* position of the model */
} GLMmodel;
/* public functions */
/* glmUnitize: "unitize" a model by translating it to the origin and
* scaling it to fit in a unit cube around the origin. Returns the
* scalefactor used.
*
* model - properly initialized GLMmodel structure
*/
GLfloat
glmUnitize(GLMmodel* model);
/* glmDimensions: Calculates the dimensions (width, height, depth) of
* a model.
*
* model - initialized GLMmodel structure
* dimensions - array of 3 GLfloats (GLfloat dimensions[3])
*/
GLvoid
glmDimensions(GLMmodel* model, GLfloat* dimensions);
/* glmScale: Scales a model by a given amount.
*
* model - properly initialized GLMmodel structure
* scale - scalefactor (0.5 = half as large, 2.0 = twice as large)
*/
GLvoid
glmScale(GLMmodel* model, GLfloat scale);
/* glmReverseWinding: Reverse the polygon winding for all polygons in
* this model. Default winding is counter-clockwise. Also changes
* the direction of the normals.
*
* model - properly initialized GLMmodel structure
*/
GLvoid
glmReverseWinding(GLMmodel* model);
/* glmFacetNormals: Generates facet normals for a model (by taking the
* cross product of the two vectors derived from the sides of each
* triangle). Assumes a counter-clockwise winding.
*
* model - initialized GLMmodel structure
*/
GLvoid
glmFacetNormals(GLMmodel* model);
/* glmVertexNormals: Generates smooth vertex normals for a model.
* First builds a list of all the triangles each vertex is in. Then
* loops through each vertex in the the list averaging all the facet
* normals of the triangles each vertex is in. Finally, sets the
* normal index in the triangle for the vertex to the generated smooth
* normal. If the dot product of a facet normal and the facet normal
* associated with the first triangle in the list of triangles the
* current vertex is in is greater than the cosine of the angle
* parameter to the function, that facet normal is not added into the
* average normal calculation and the corresponding vertex is given
* the facet normal. This tends to preserve hard edges. The angle to
* use depends on the model, but 90 degrees is usually a good start.
*
* model - initialized GLMmodel structure
* angle - maximum angle (in degrees) to smooth across
*/
GLvoid
glmVertexNormals(GLMmodel* model, GLfloat angle);
/* glmLinearTexture: Generates texture coordinates according to a
* linear projection of the texture map. It generates these by
* linearly mapping the vertices onto a square.
*
* model - pointer to initialized GLMmodel structure
*/
GLvoid
glmLinearTexture(GLMmodel* model);
/* glmSpheremapTexture: Generates texture coordinates according to a
* spherical projection of the texture map. Sometimes referred to as
* spheremap, or reflection map texture coordinates. It generates
* these by using the normal to calculate where that vertex would map
* onto a sphere. Since it is impossible to map something flat
* perfectly onto something spherical, there is distortion at the
* poles. This particular implementation causes the poles along the X
* axis to be distorted.
*
* model - pointer to initialized GLMmodel structure
*/
GLvoid
glmSpheremapTexture(GLMmodel* model);
/* glmDelete: Deletes a GLMmodel structure.
*
* model - initialized GLMmodel structure
*/
GLvoid
glmDelete(GLMmodel* model);
/* glmReadOBJ: Reads a model description from a Wavefront .OBJ file.
* Returns a pointer to the created object which should be free'd with
* glmDelete().
*
* filename - name of the file containing the Wavefront .OBJ format data.
*/
GLMmodel*
glmReadOBJ(char* filename);
/* glmWriteOBJ: Writes a model description in Wavefront .OBJ format to
* a file.
*
* model - initialized GLMmodel structure
* filename - name of the file to write the Wavefront .OBJ format data to
* mode - a bitwise or of values describing what is written to the file
* GLM_NONE - write only vertices
* GLM_FLAT - write facet normals
* GLM_SMOOTH - write vertex normals
* GLM_TEXTURE - write texture coords
* GLM_FLAT and GLM_SMOOTH should not both be specified.
*/
GLvoid
glmWriteOBJ(GLMmodel* model, char* filename, GLuint mode);
/* glmDraw: Renders the model to the current OpenGL context using the
* mode specified.
*
* model - initialized GLMmodel structure
* mode - a bitwise OR of values describing what is to be rendered.
* GLM_NONE - render with only vertices
* GLM_FLAT - render with facet normals
* GLM_SMOOTH - render with vertex normals
* GLM_TEXTURE - render with texture coords
* GLM_FLAT and GLM_SMOOTH should not both be specified.
*/
GLvoid
glmDraw(GLMmodel* model, GLuint mode);
/* glmList: Generates and returns a display list for the model using
* the mode specified.
*
* model - initialized GLMmodel structure
* mode - a bitwise OR of values describing what is to be rendered.
* GLM_NONE - render with only vertices
* GLM_FLAT - render with facet normals
* GLM_SMOOTH - render with vertex normals
* GLM_TEXTURE - render with texture coords
* GLM_FLAT and GLM_SMOOTH should not both be specified.
*/
GLuint
glmList(GLMmodel* model, GLuint mode);
/* glmWeld: eliminate (weld) vectors that are within an epsilon of
* each other.
*
* model - initialized GLMmodel structure
* epsilon - maximum difference between vertices
* ( 0.00001 is a good start for a unitized model)
*
*/
GLvoid
glmWeld(GLMmodel* model, GLfloat epsilon);
2, 在第17篇的基础上,CCY457OpenGLView类中加入下述变量,用来表示不同物体类型
GLuint m_MonitorList; //显示器
GLuint m_ChairList; //椅子
GLuint m_PotList; //花瓶
GLuint m_ComputerList; //计算机
int m_nObjectNo;
2, 在InitializeOpenGL函数中加入对LoadModelsFromFiles的调用
3, 绘制函数修改如下:
void CCY457OpenGLView::RenderScene ()
{//绘制函数
//Position Camera
gluLookAt(m_PosX,m_PosY,m_PosZ,m_DirX,m_DirY,m_DirZ,0.0f,1.0f,0.0f);
//Draw the Scene
//Draw the floor
// Draw the ground, we do manual shading to a darker green
// in the background to give the illusion of depth
glEnable(GL_TEXTURE_2D);
glBindTexture(GL_TEXTURE_2D, m_Texture[3]);
glBegin(GL_POLYGON);
glColor3ub(0,255,0);
glTexCoord2f(0.0f, 0.0f);
glVertex3f(-2.0f, 0.0f, 0.0f);
glTexCoord2f(1.0f, 0.0f);
glVertex3f(2.0f,0.0f, 0.0f);
glColor3ub(0,100,0);
glTexCoord2f(1.0f, 1.0f);
glVertex3f(2.0f, 0.0f, -2.0f);
glTexCoord2f(0.0f, 1.0f);
glVertex3f(-2.0f,0.0f, -2.0f);
glEnd();
glDisable(GL_TEXTURE_2D);
//Draw the Cube
// Save the matrix state and do the rotations
glPushMatrix();
glTranslatef(-1.0f,0.6f,-1.0f);
// Draw jet at new orientation, put light in correct position
// before rotating the jet
glRotatef(m_xRot,1.0f,0.0f,0.0f);
glRotatef(m_yRot,0.0f,1.0f,0.0f);
DrawCube(FALSE);
// Restore original matrix state
glPopMatrix();
// Get ready to draw the shadow and the ground
// First disable lighting and save the projection state
glDisable(GL_DEPTH_TEST);
glDisable(GL_LIGHTING);
glPushMatrix();
// Multiply by shadow projection matrix
glMultMatrixf((GLfloat *)m_ShadowMat);
glTranslatef(-1.0f,0.6f,-1.0f);
glRotatef(m_xRot,1.0f,0.0f,0.0f);
glRotatef(m_yRot,0.0f,1.0f,0.0f);
// Pass true to indicate drawing shadow
DrawCube(TRUE);
// Restore the projection to normal
glPopMatrix();
// Restore lighting state variables
glEnable(GL_DEPTH_TEST);
// Draw the light source
glPushMatrix();
glTranslatef(1.5f,1.5f,-1.0f);
glColor3ub(255,255,0);
glutSolidSphere(0.01f,10,10);
glPopMatrix();
glEnable(GL_LIGHTING);
}
void CCY457OpenGLView::DrawCube (BOOL bShadow)
{
// Set material color, note we only have to set to black
// for the shadow once
if(!bShadow)
{
switch (m_nObjectNo)
{
case 0: glCallList(m_ChairList);
break;
case 1: glCallList(m_PotList);
break;
case 2: glCallList(m_ComputerList);
break;
case 3: glCallList(m_MonitorList);
break;
case 4: DrawCubeTex();
break;
}
}
else
{
glColor3ub(0,0,0);
switch (m_nObjectNo)
{
case 0: glCallList(m_ChairList);
break;
case 1: glCallList(m_PotList);
break;
case 2: glCallList(m_ComputerList);
break;
case 3: glCallList(m_MonitorList);
break;
case 4: DrawCubeNoTex();
break;
}
}
}
4, 加载Obj文件的具体实现代码:
//Load all the Models from the Files of type .obj
void CCY457OpenGLView::LoadModelsFromFiles()
{
GLfloat scalefactor = 0.0;
//Load Computer from file
GLMmodel *object1;
object1 = glmReadOBJ("models/computer.obj");
if(!scalefactor)
{
scalefactor = glmUnitize(object1);
}
else
{
glmScale(object1, scalefactor);
}
glmScale(object1, 2.5);
/* build a display list */
m_ComputerList = glmList(object1, GLM_SMOOTH);
/* nuke it, we don't need it anymore */
glmDelete(object1);
//Load Chair From File
GLMmodel *object2;
scalefactor = 0.0;
object2 = glmReadOBJ("models/chair04.obj");
if(!scalefactor)
{
scalefactor = glmUnitize(object2);
}
else
{
glmScale(object2, scalefactor);
}
glmScale(object2, 5.0);
/* build a display list */
m_ChairList = glmList(object2, GLM_SMOOTH);
/* nuke it, we don't need it anymore */
glmDelete(object2);
//Load Monitor from file
GLMmodel *object5;
scalefactor = 0.0;
object5 = glmReadOBJ("models/samsung.obj");
if(!scalefactor)
{
scalefactor = glmUnitize(object5);
}
else
{
glmScale(object5, scalefactor);
}
glmScale(object5, 0.5);
/* build a display list */
m_MonitorList = glmList(object5, GLM_SMOOTH);
/* nuke it, we don't need it anymore */
glmDelete(object5);
//Load Phone Object from file
GLMmodel *object6;
scalefactor = 0.0;
object6 = glmReadOBJ("models/plant2.obj");
if(!scalefactor)
{
scalefactor = glmUnitize(object6);
}
else
{
glmScale(object6, scalefactor);
}
glmScale(object6, 0.5);
/* build a display list */
m_PotList = glmList(object6, GLM_SMOOTH);
/* nuke it, we don't need it anymore */
glmDelete(object6);
}
原文链接:
http://www.cnblogs.com/phinecos/archive/2008/11/07/1328957.html